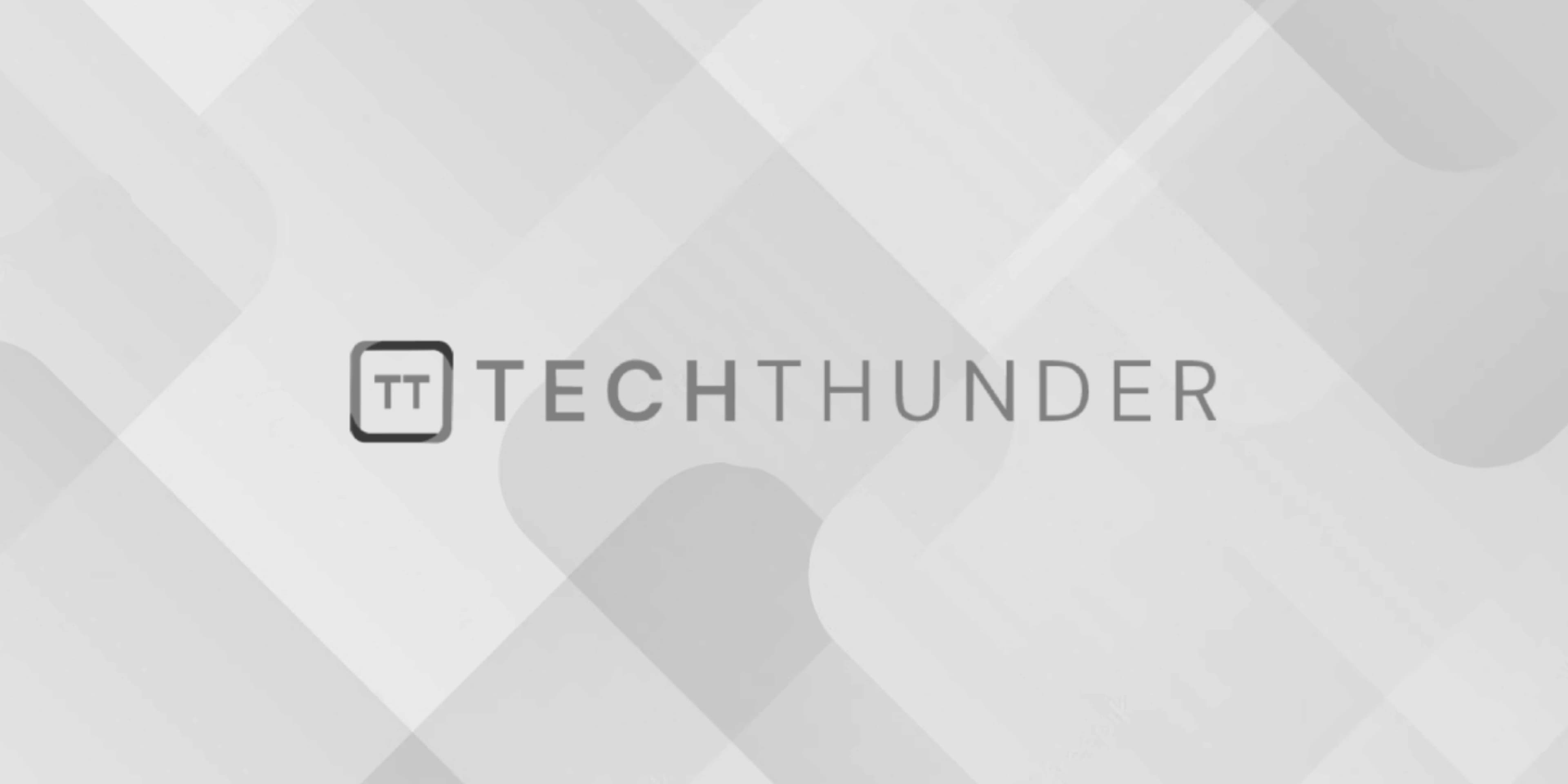
291 views
C String Test
The testing strings often involves comparing strings for equality or performing other string-related operations. Here’s a simple example of testing strings in C:
C
#include <stdio.h>
#include <string.h>
int main() {
char str1[] = "Hello";
char str2[] = "World";
char str3[] = "Hello";
char str4[] = "HELLO";
// Compare two strings for equality
if (strcmp(str1, str3) == 0) {
printf("str1 and str3 are equal.\n");
} else {
printf("str1 and str3 are not equal.\n");
}
// Case-insensitive string comparison
if (strcasecmp(str1, str4) == 0) {
printf("str1 and str4 are equal (case-insensitive).\n");
} else {
printf("str1 and str4 are not equal (case-insensitive).\n");
}
// Test string length
if (strlen(str2) < 5) {
printf("str2 is shorter than 5 characters.\n");
} else {
printf("str2 is 5 characters or longer.\n");
}
// Concatenate strings
strcat(str1, " ");
strcat(str1, str2);
printf("Concatenated string: %s\n", str1);
return 0;
}
In this example, we perform various string-related tests:
- We use
strcmp()
to comparestr1
andstr3
for equality and print the result. - We use
strcasecmp()
to perform a case-insensitive comparison betweenstr1
andstr4
and print the result. - We use
strlen()
to check the length ofstr2
and print whether it’s shorter than 5 characters or not. - We use
strcat()
to concatenatestr1
andstr2
, adding a space in between, and then print the concatenated string.
You can adapt and extend these string tests based on your specific requirements in your C program. Testing and comparing strings are common tasks in C programming, especially when working with user input or data processing.