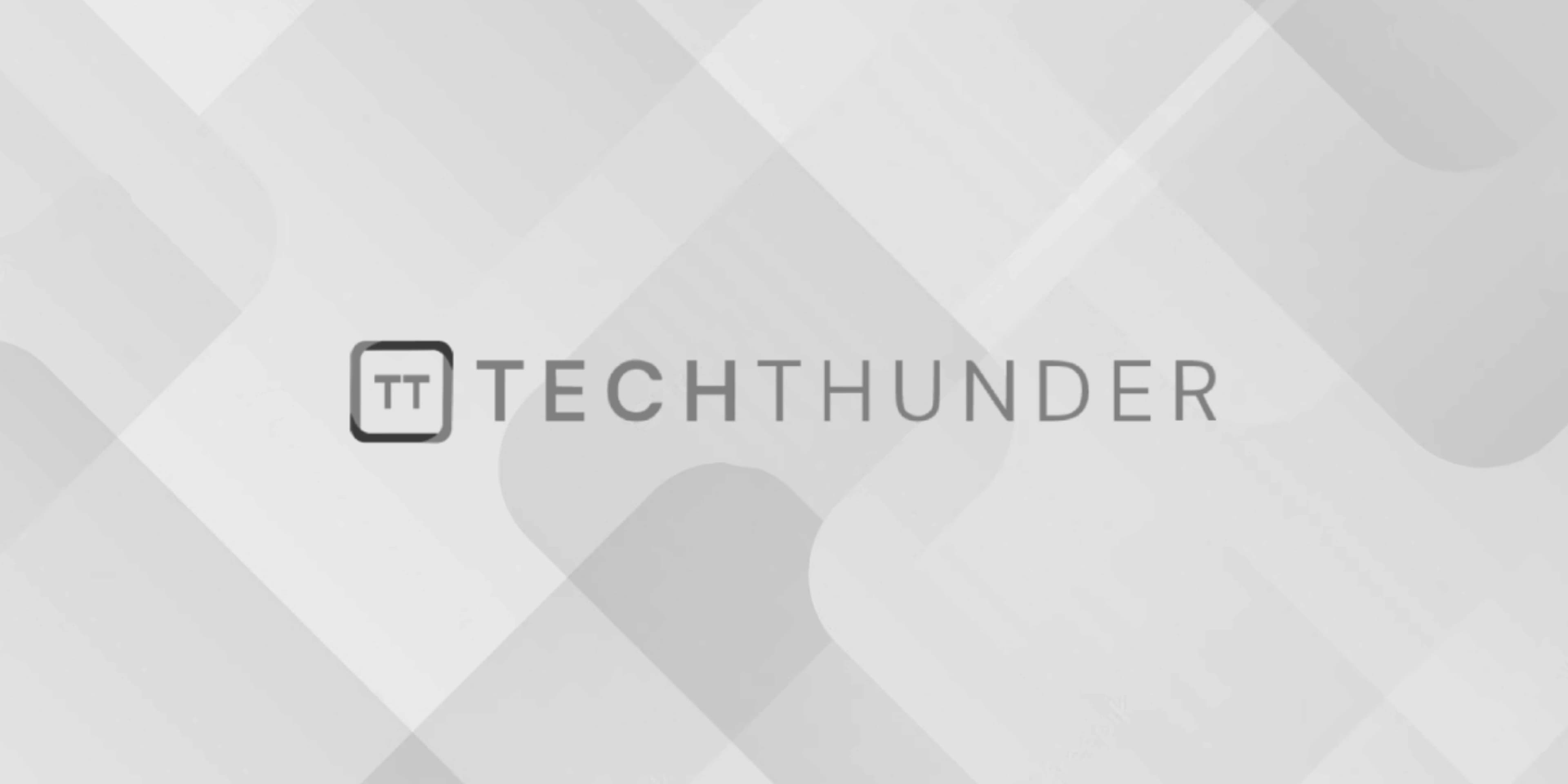
173 views
Fibonacci Numbers List in C
Generating a list of Fibonacci numbers is a classic programming exercise. Fibonacci numbers are a sequence of numbers where each number is the sum of the two preceding ones. Here’s a simple C program that generates a list of Fibonacci numbers within a specified range:
C
#include <stdio.h>
// Function to generate Fibonacci numbers
void generateFibonacci(int n) {
int prev = 0, curr = 1;
printf("Fibonacci numbers up to %d:\n", n);
while (curr <= n) {
printf("%d ", curr);
int next = prev + curr;
prev = curr;
curr = next;
}
printf("\n");
}
int main() {
int upperBound;
printf("Enter the upper bound: ");
scanf("%d", &upperBound);
generateFibonacci(upperBound);
return 0;
}
In this program, the generateFibonacci
function generates Fibonacci numbers up to a specified upper bound. The main program takes user input for the upper bound and then calls the generateFibonacci
function.
Please note that Fibonacci numbers can grow quickly, so if you’re working with larger ranges, you might need to consider optimizations for generating Fibonacci numbers efficiently.
Additionally, remember to validate user input to ensure it’s within an appropriate range.