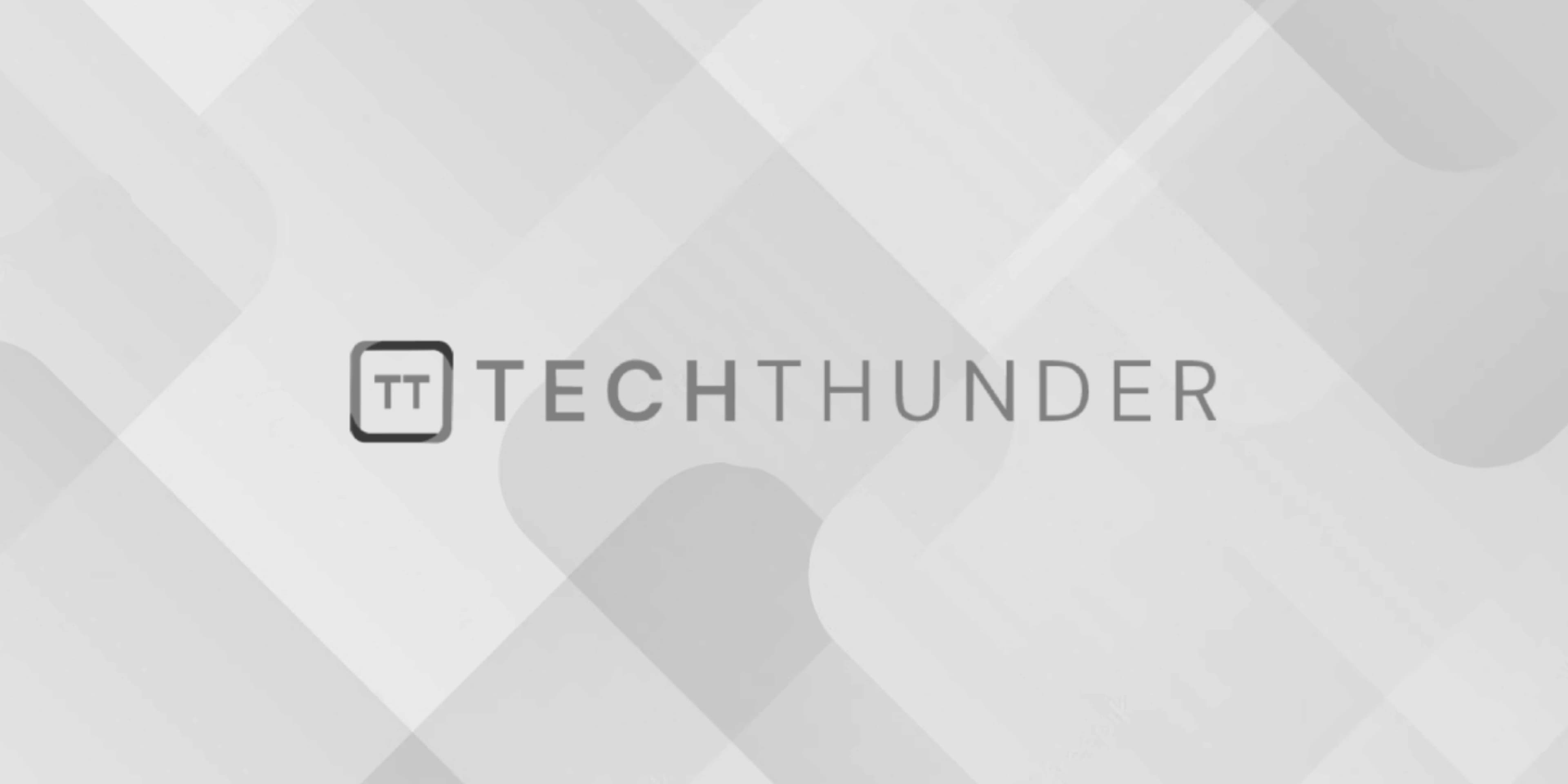
C strrev()
There is no standard library function named strrev()
for reversing a string in place. However, you can easily implement your own function to reverse a string. Here’s an example of how you can do it:
#include <stdio.h>
#include <string.h>
void reverseString(char *str) {
int length = strlen(str);
for (int i = 0, j = length - 1; i < j; i++, j--) {
char temp = str[i];
str[i] = str[j];
str[j] = temp;
}
}
int main() {
char str[] = "Hello, World!";
printf("Original string: %s\n", str);
reverseString(str);
printf("Reversed string: %s\n", str);
return 0;
}
In this example, we define a reverseString()
function that takes a string as an argument and reverses it in place. It uses two pointers, one starting at the beginning of the string (i
) and the other starting at the end of the string (j
), and swaps characters until they meet in the middle of the string.
Please note that this code modifies the original string directly. If you need to keep the original string unchanged, you should make a copy of the string and then reverse the copy.
Keep in mind that this implementation assumes that the input string is null-terminated. If the string is not null-terminated, you should pass the length of the string as an additional argument to the reverseString()
function.