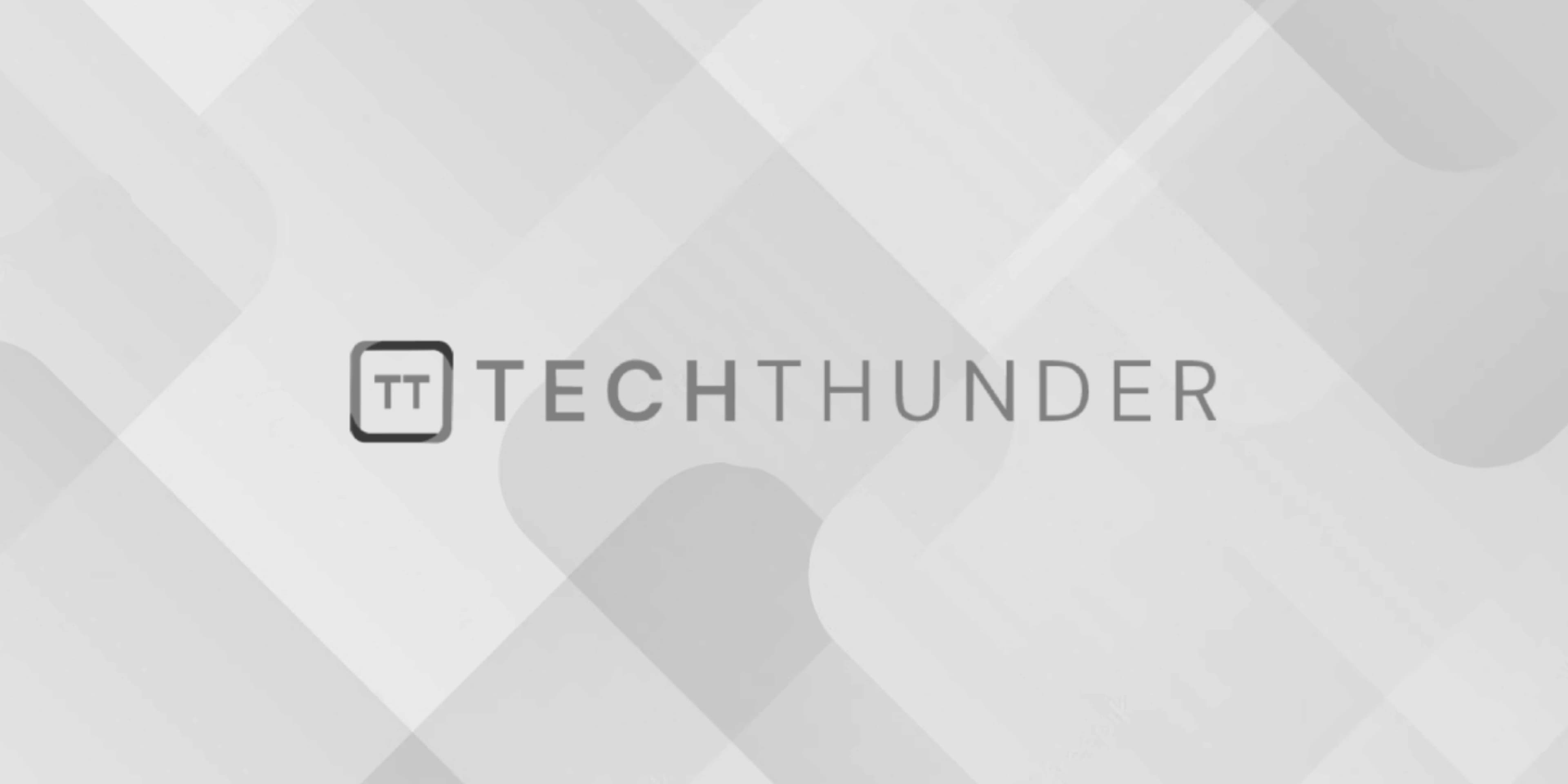
266 views
Memory Layout in C
Memory layout in C refers to the organization of memory segments or regions used by a C program during its execution. Understanding the memory layout is crucial for writing efficient and safe C programs. The memory layout can vary slightly depending on the system and compiler, but the basic structure includes the following segments:
Text Segment (Code Segment):
- The text segment contains the executable code of the program.
- It is typically marked as read-only, meaning that you cannot modify the code while the program is running.
- The machine code instructions of your program reside in this segment.
- Also known as the “code segment” because it contains the program’s instructions.
Data Segment:
- The data segment is further divided into two parts:
- Initialized Data Segment (or Data Section):
- This part of the data segment contains global and static variables that are explicitly initialized with values.
- Initialized variables are stored in a dedicated region of memory with their initial values.
- Uninitialized Data Segment (BSS – Block Started by Symbol):
- The BSS segment contains global and static variables that are uninitialized or explicitly initialized to zero.
- These variables are allocated space in memory but not initialized to specific values.
Heap:
- The heap is a dynamically allocated region of memory used for storing data that can be allocated and deallocated at runtime.
- Memory allocated on the heap is managed by functions like
malloc
,calloc
,realloc
, and freed usingfree
. - The heap is used for dynamic data structures like linked lists, trees, and other data that must persist across function calls.
Stack:
- The stack is a region of memory used for function call management and local variables.
- Each function call in C typically creates a new stack frame, which includes the function’s parameters, return address, and local variables.
- The stack follows a last-in, first-out (LIFO) order, meaning that the most recently called function is at the top of the stack.
- As functions return, their stack frames are removed (popped) from the stack.
Environment Variables (Environment Variables Segment):
- This segment stores environment variables that can be accessed by the program.
- Environment variables are key-value pairs that can affect program behavior.
- You can access environment variables using functions like
getenv
in C.
Command Line Arguments (Command Line Arguments Segment):
- This segment stores command-line arguments passed to the program when it’s executed.
- Command-line arguments can be accessed through the
main
function’s argument list (argc
andargv
).
Understanding these memory segments and managing memory properly is essential for writing efficient and reliable C programs. Incorrect memory access or management can lead to bugs, crashes, or security vulnerabilities.