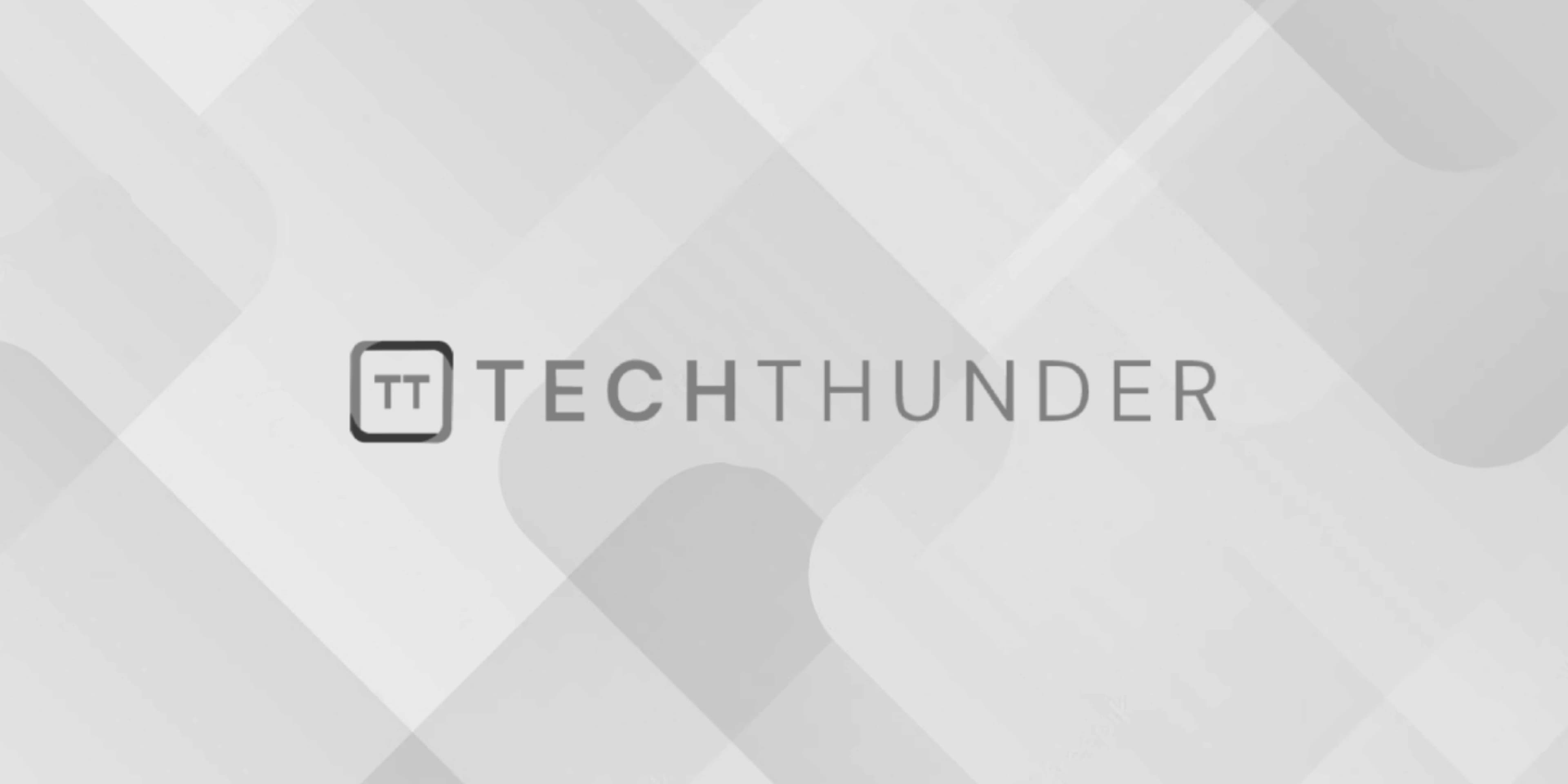
164 views
Boolean in C
The concept of a boolean type is not natively supported as it is in some other programming languages like C++, Java, or Python. However, C uses integers to represent boolean values, where 0
typically represents “false,” and any non-zero value represents “true.” Here’s how boolean logic works in C:
- False: In C, a condition or expression is considered “false” if its value is
0
. For example:
C
int isTrue = 0; // Represents false
if (isTrue) {
// This code won't be executed
}
- True: Any non-zero value is considered “true.” This includes positive integers and negative integers. For example:
C
int isTrue = 42; // Represents true
if (isTrue) {
// This code will be executed
}
- Logical Operators: C provides logical operators to work with boolean expressions. These operators include:
&&
(logical AND): Returns true if both operands are true.||
(logical OR): Returns true if at least one operand is true.!
(logical NOT): Negates the value of the operand.
C
int a = 10;
int b = 20;
if (a > 5 && b < 30) {
// This code will be executed because both conditions are true
}
- Boolean Typedef (Optional): Although C doesn’t have a built-in boolean type, you can create your own boolean typedef for code clarity:
C
typedef int bool;
#define true 1
#define false 0
int main() {
bool isTrue = true;
if (isTrue) {
// This code will be executed
}
return 0;
}
This approach improves code readability by using true
and false
identifiers.
While C lacks a native boolean type, you can effectively use integers to represent boolean values by adhering to the convention that 0
means “false” and non-zero values mean “true.” This approach is widely accepted in C programming.