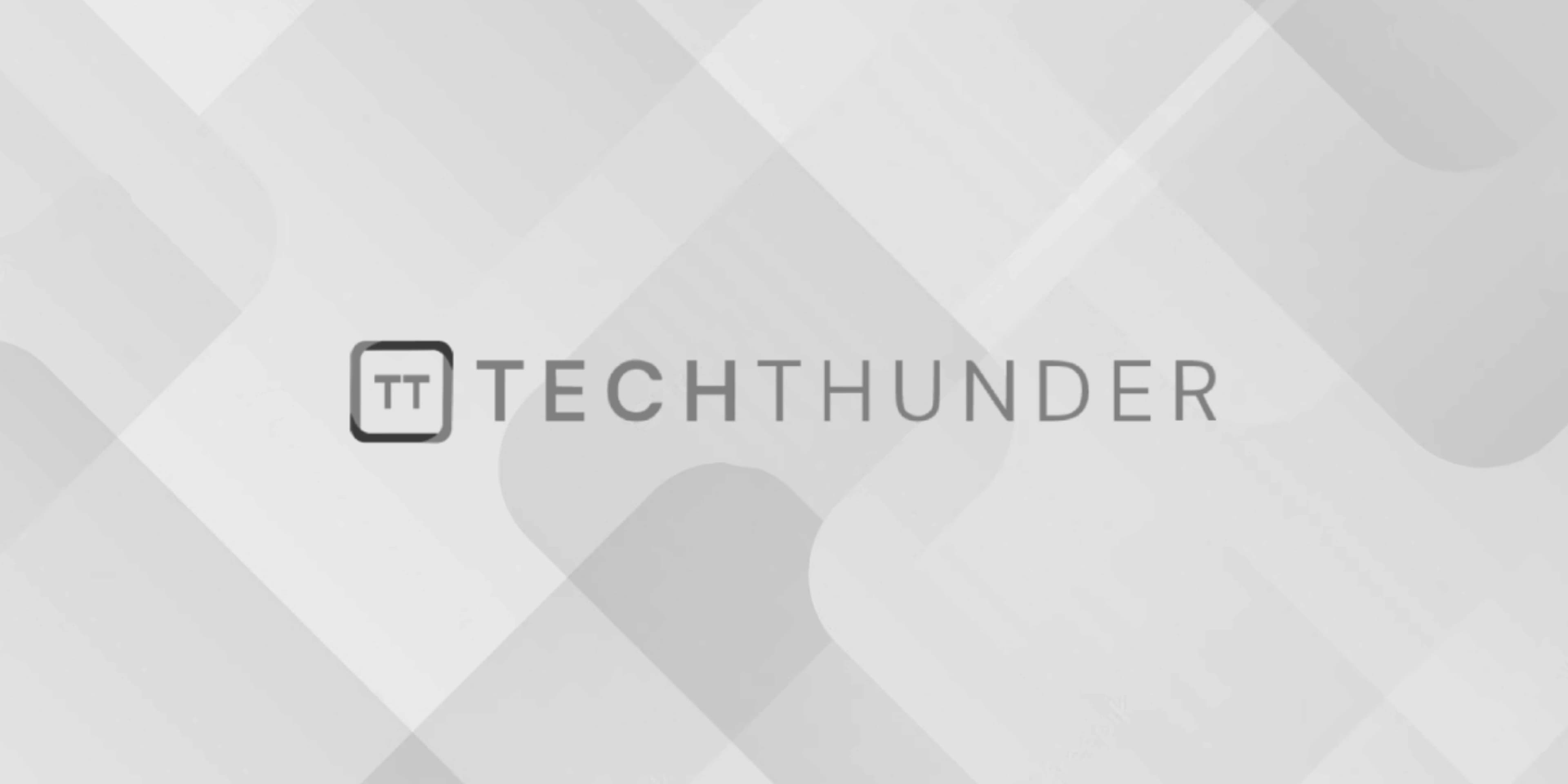
113 views
Add Element in Array in C
To add an element to an array in C, you need to create a new array with a size that is one element larger than the original array, copy the elements from the original array to the new array, and then add the new element to the end of the new array. Here’s a C program that demonstrates how to add an element to an array:
C
#include <stdio.h>
int main() {
int originalArray[100]; // Declare an array (you can change the size as needed)
int newArray[101]; // Declare a new array with one extra element
int size; // Size of the original array
int newValue; // Value to be added
int i;
// Input the size of the original array
printf("Enter the size of the array: ");
scanf("%d", &size);
// Input the elements of the original array
printf("Enter %d elements for the array:\n", size);
for (i = 0; i < size; i++) {
scanf("%d", &originalArray[i]);
}
// Input the new value to be added
printf("Enter the new value to add: ");
scanf("%d", &newValue);
// Copy elements from the original array to the new array
for (i = 0; i < size; i++) {
newArray[i] = originalArray[i];
}
// Add the new value to the end of the new array
newArray[size] = newValue;
// Update the size of the new array
size++;
// Display the new array
printf("Updated array:\n");
for (i = 0; i < size; i++) {
printf("%d ", newArray[i]);
}
printf("\n");
return 0;
}
In this program:
- We declare two arrays,
originalArray
to hold the original elements andnewArray
with one extra element to hold the updated array. - We input the size of the original array and its elements.
- We input the new value (
newValue
) to be added to the array. - We copy the elements from the original array to the new array using a loop.
- We add the
newValue
to the end of the new array and update the size of the new array. - Finally, we display the updated array with the added element.
Make sure to adjust the array size as needed for your specific use case.