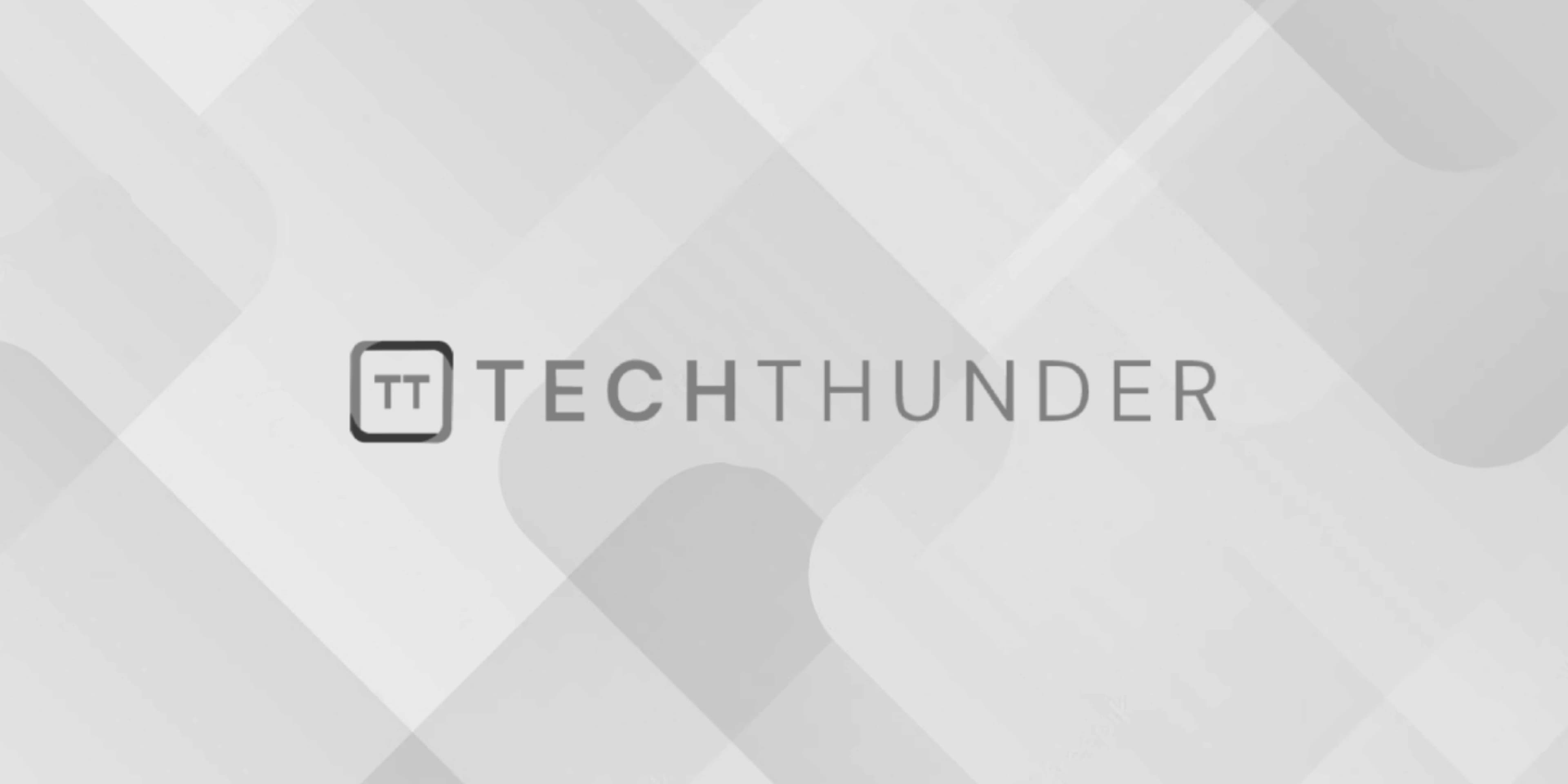
105 views
Bisection Method in C
The bisection method is a simple numerical technique used to find the root of a continuous function within a given interval. It relies on the intermediate value theorem, which states that if a continuous function changes sign within an interval, it must have at least one root within that interval.
Here’s a C program that implements the bisection method to find the root of a function within a specified interval:
C
#include <stdio.h>
#include <math.h>
// Define the function for which you want to find the root
double f(double x) {
return x * x - 4; // Example: f(x) = x^2 - 4
}
// Bisection method to find the root of a function
double bisection(double a, double b, double tol) {
double mid, fmid;
while ((b - a) / 2.0 > tol) {
mid = (a + b) / 2.0;
fmid = f(mid);
if (fmid == 0.0) {
return mid; // Root found
} else if (f(a) * fmid < 0) {
b = mid;
} else {
a = mid;
}
}
return (a + b) / 2.0; // Approximation of the root
}
int main() {
double a, b, tol;
printf("Enter the interval [a, b]: ");
scanf("%lf %lf", &a, &b);
printf("Enter the tolerance (e.g., 0.001): ");
scanf("%lf", &tol);
if (f(a) * f(b) >= 0) {
printf("Cannot apply the bisection method on this interval. The function values at 'a' and 'b' must have opposite signs.\n");
return 1;
}
double root = bisection(a, b, tol);
printf("Approximate root within the tolerance: %lf\n", root);
return 0;
}
In this program:
- The
f
function represents the function for which you want to find the root. You can modify thef
function to match the specific function you are interested in. - The
bisection
function implements the bisection method. It takes the interval[a, b]
and a tolerance value as inputs and iteratively refines the interval until the root is found within the specified tolerance. - The
main
function takes user input for the interval[a, b]
and the tolerance. It checks whether the function values ata
andb
have opposite signs (a requirement for the bisection method), and then it calls thebisection
function to find the root.
This program will approximate the root of the specified function within the given interval and tolerance using the bisection method.