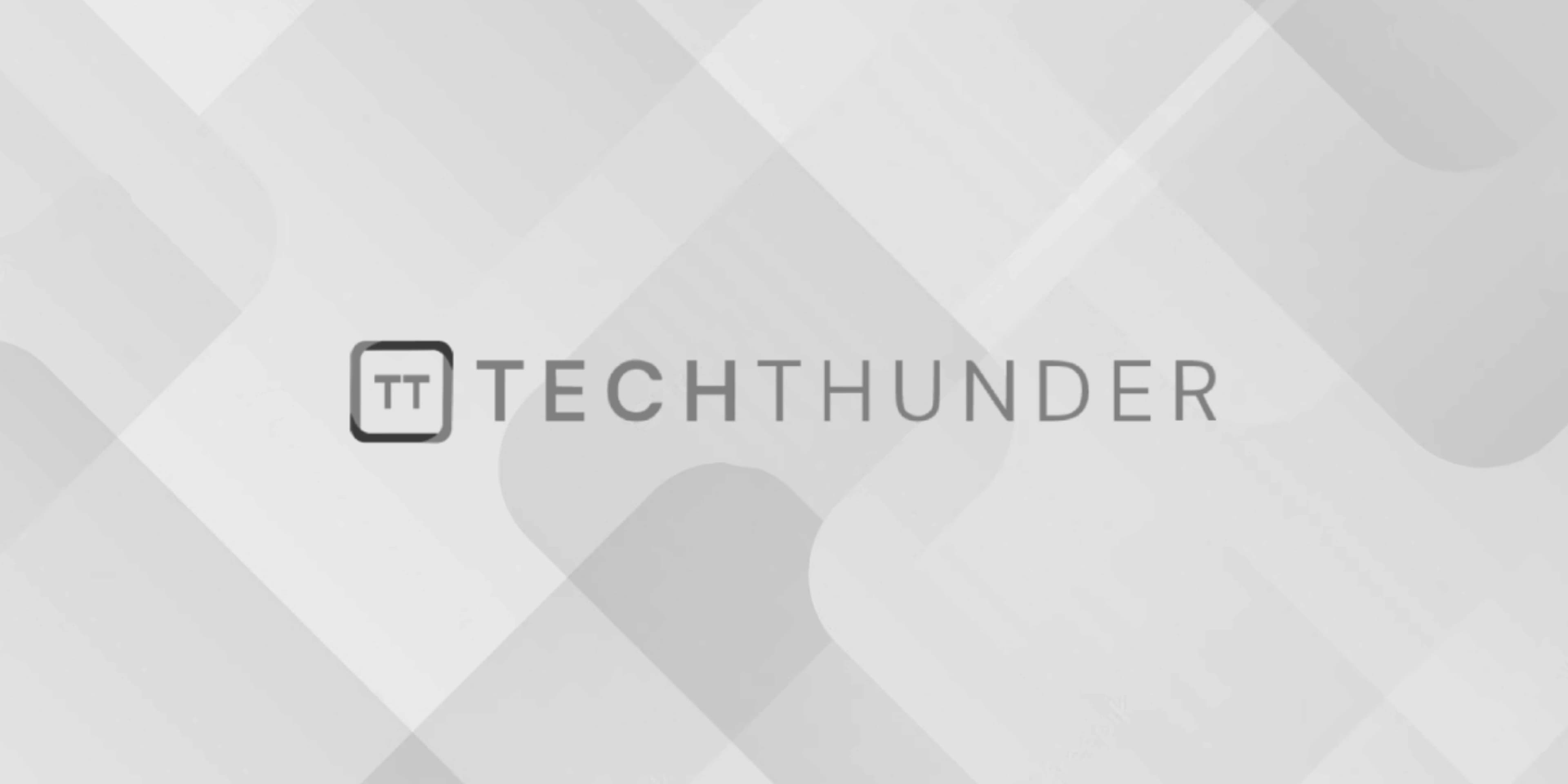
235 views
C #else
The #else
directive is used in combination with conditional compilation directives like #ifdef
, #ifndef
, and #if
to specify code that should be executed when a certain condition is not met. It is typically used to provide an alternative code path when the condition specified in the previous #ifdef
, #ifndef
, or #if
directive is false.
Here’s the basic syntax of the #else
directive:
C
#ifdef macro_name
// Code to execute if macro_name is defined
#else
// Code to execute if macro_name is not defined
#endif
macro_name
is the name of the macro being tested.
Here’s an example that demonstrates the usage of #else
:
C
#include <stdio.h>
#define DEBUG // Define DEBUG macro
int main() {
#ifdef DEBUG
printf("Debug mode is enabled.\n");
#else
printf("Debug mode is disabled.\n");
#endif
#ifndef RELEASE
printf("This code will be executed because RELEASE is not defined.\n");
#else
printf("This code will not be executed because RELEASE is defined.\n");
#endif
return 0;
}
In this example:
- The
DEBUG
macro is defined at the beginning of the program using#define
. - Inside the program, conditional compilation (
#ifdef
and#ifndef
) is used to check whetherDEBUG
andRELEASE
macros are defined. - If
DEBUG
is defined, the program prints “Debug mode is enabled.” Otherwise, it prints “Debug mode is disabled.” - If
RELEASE
is not defined, the program prints “This code will be executed because RELEASE is not defined.” Otherwise, it would print “This code will not be executed because RELEASE is defined.”
The #else
directive provides a way to specify an alternative code path based on whether a certain macro is defined or not. This allows you to create flexible and configurable code that can be adapted for different build configurations or purposes.