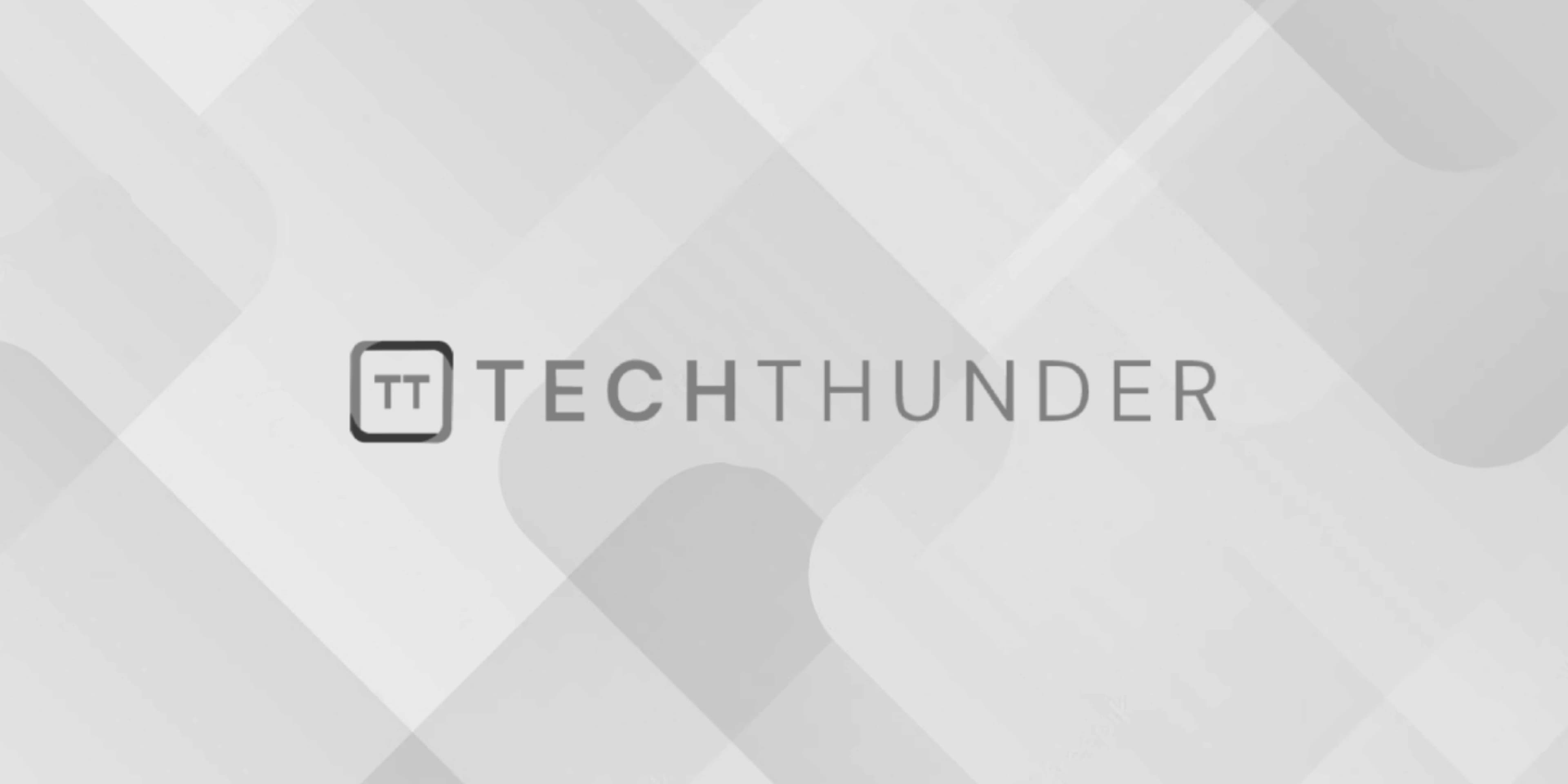
254 views
Window Sliding Technique in C
The sliding window technique is a common algorithmic approach used to solve problems that involve subarrays or subsequences of a given array or string. It is especially useful when you need to efficiently track and update a subset of elements within a larger data structure while iterating through it.
Here’s a simple C program that demonstrates the sliding window technique for finding the maximum sum of a subarray of a fixed size k
within an array:
C
#include <stdio.h>
// Function to find the maximum sum of a subarray of size k
int maxSumSubarray(int arr[], int n, int k) {
int maxSum = 0;
int currentSum = 0;
// Calculate the sum of the first k elements
for (int i = 0; i < k; i++) {
currentSum += arr[i];
}
maxSum = currentSum;
// Slide the window and update the sums
for (int i = k; i < n; i++) {
currentSum += arr[i] - arr[i - k];
if (currentSum > maxSum) {
maxSum = currentSum;
}
}
return maxSum;
}
int main() {
int arr[] = {1, 2, 3, 4, 5, 6, 7, 8, 9};
int n = sizeof(arr) / sizeof(arr[0]);
int k = 3;
int maxSum = maxSumSubarray(arr, n, k);
printf("Maximum sum of a subarray of size %d is: %d\n", k, maxSum);
return 0;
}
In this program:
- We define the
maxSumSubarray
function, which takes an arrayarr
, its sizen
, and the subarray sizek
as parameters. - We initialize
maxSum
andcurrentSum
to 0. - We calculate the sum of the first
k
elements in the array and store it incurrentSum
. - We set
maxSum
tocurrentSum
. - We then slide the window from index
k
ton-1
, updatingcurrentSum
by subtracting the element that goes out of the window and adding the new element that enters the window. - We keep track of the maximum sum found in
maxSum
. - Finally, we return
maxSum
.
In the main
function, we call maxSumSubarray
with a sample array and subarray size and print the maximum sum.
You can modify this program to solve other sliding window problems by adapting the logic inside the maxSumSubarray
function.