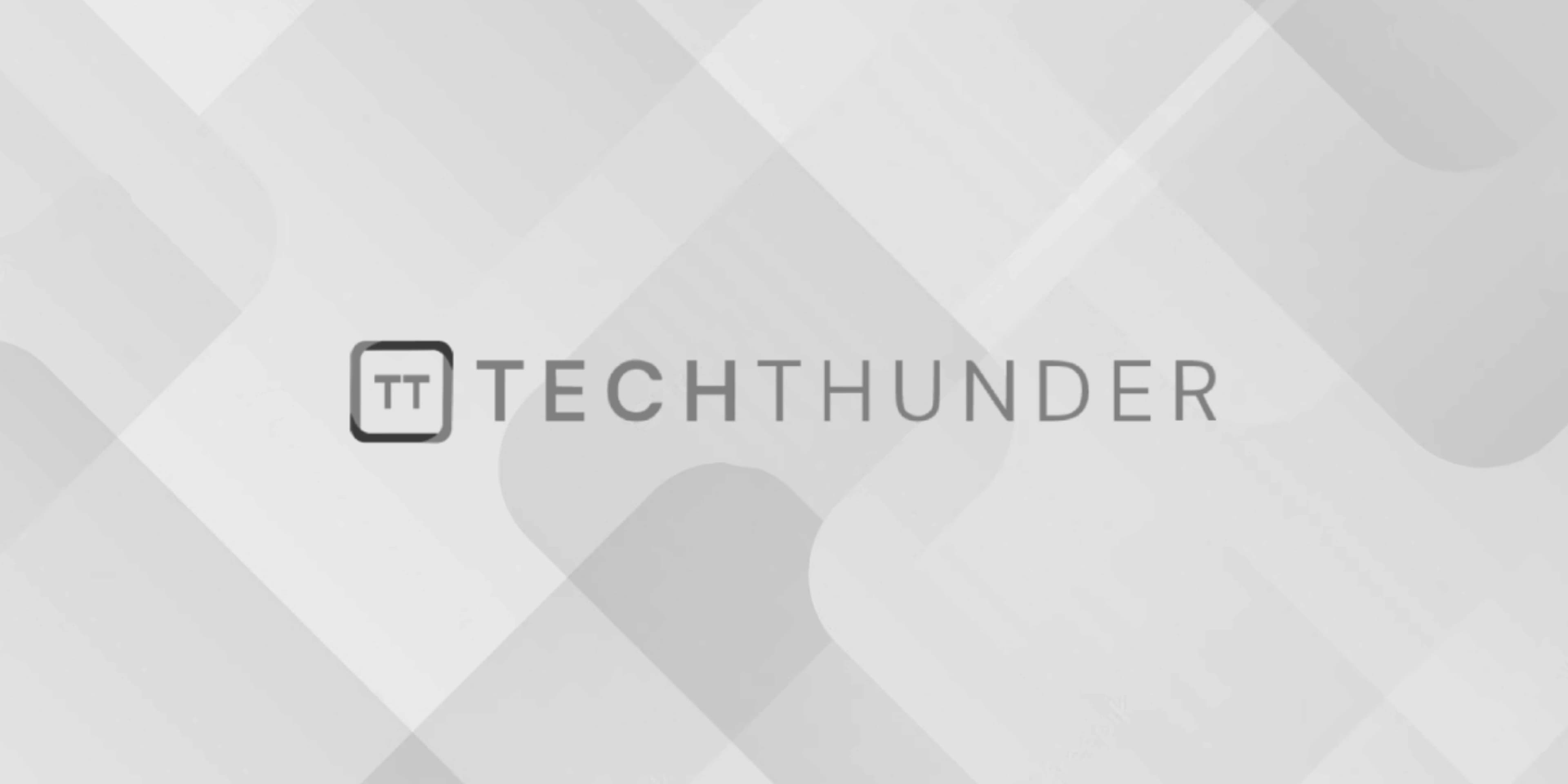
Conditional Operator in C
The conditional operator (also known as the ternary operator) is a shorthand way to express a conditional statement. It allows you to evaluate a condition and choose between two possible values or expressions based on whether the condition is true or false. The conditional operator has the following syntax:
condition ? expression1 : expression2
condition
: An expression that is evaluated as either true (non-zero) or false (zero).expression1
: The value or expression to be returned if the condition is true.expression2
: The value or expression to be returned if the condition is false.
The conditional operator is often used as a more concise alternative to an if-else
statement when assigning a value based on a condition.
Here’s an example that demonstrates the use of the conditional operator to find the maximum of two numbers:
#include <stdio.h>
int main() {
int a = 5, b = 7;
int max = (a > b) ? a : b; // Find the maximum of a and b using the conditional operator
printf("The maximum of %d and %d is %d\n", a, b, max);
return 0;
}
In this example:
- The condition
(a > b)
is evaluated first. - If the condition is true (i.e.,
a
is greater thanb
), the value ofa
is assigned tomax
. - If the condition is false (i.e.,
a
is not greater thanb
), the value ofb
is assigned tomax
.
So, the output of this program will be “The maximum of 5 and 7 is 7.”
The conditional operator is particularly useful when you want to make a quick decision between two values or expressions based on a condition. It can make your code more concise and easier to read in such cases. However, it should be used judiciously to maintain code readability and avoid excessive complexity.