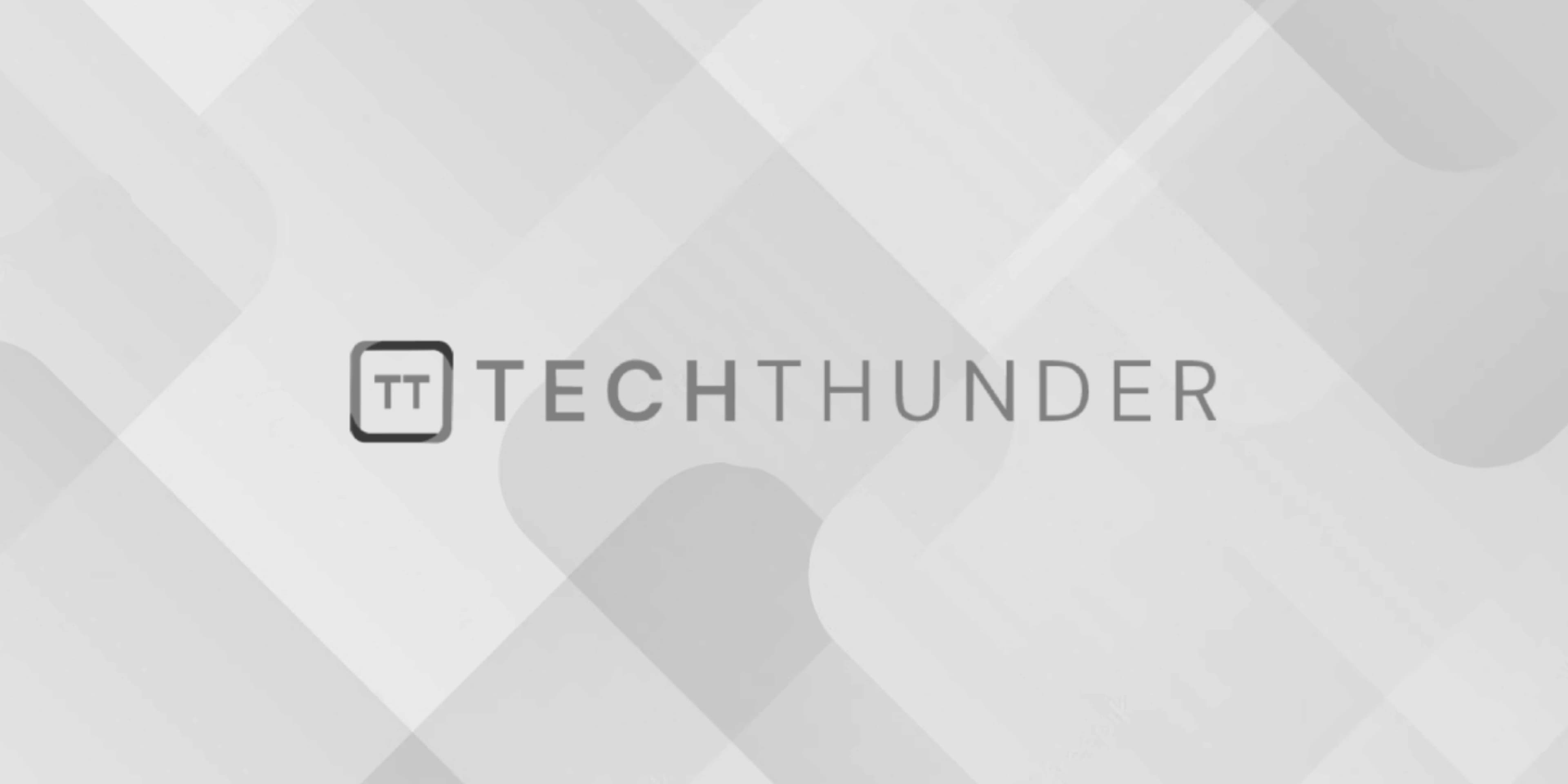
236 views
C Dereference Pointer
The process of accessing the value stored at a memory location pointed to by a pointer is called “dereferencing” a pointer. Dereferencing is performed using the unary *
operator. When you use *
followed by a pointer variable, it retrieves the value stored at the memory address the pointer is pointing to.
Here’s an example of dereferencing a pointer:
C
#include <stdio.h>
int main() {
int x = 42; // Declare an integer variable x and assign it a value
int *ptr = &x; // Declare a pointer ptr and initialize it with the address of x
printf("Value of x: %d\n", x); // Prints the value of x
printf("Value via pointer: %d\n", *ptr); // Dereferencing ptr to get the value of x
return 0;
}
In this example:
- We declare an integer variable
x
and assign it a value of42
. - We declare a pointer
ptr
and initialize it with the address ofx
using the address-of operator&
. - To access the value stored at the memory location pointed to by
ptr
, we use*ptr
. The expression*ptr
dereferences the pointer and retrieves the value stored inx
. - We use
printf
to print both the value ofx
and the value accessed via the pointerptr
.
When you run this program, it will display:
Plaintext
Value of x: 42
Value via pointer: 42
Dereferencing pointers is essential in C programming when you want to manipulate or access data stored in memory through pointer variables. It allows you to work with the values that pointers point to, making pointers a powerful tool for memory management and data manipulation in C.