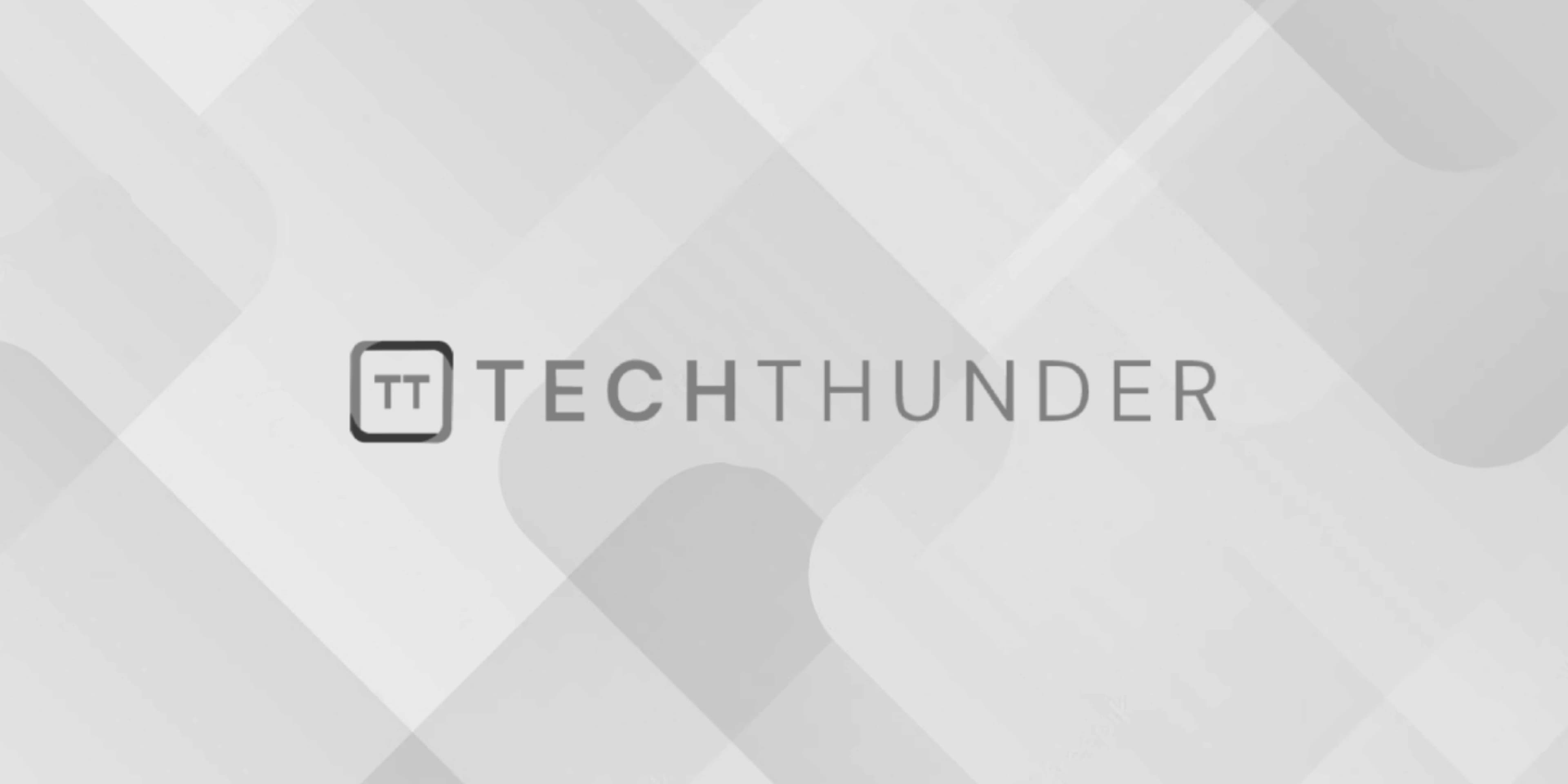
C strlwr()
The strlwr()
is not a standard C library function. It’s worth noting that standard C libraries do not include a direct function for converting an entire string to lowercase. However, you can achieve this by iterating over the characters in the string and converting each character to its lowercase equivalent using the tolower()
function from the <ctype.h>
header.
Here’s an example of how you can manually convert a string to lowercase in C:
#include <stdio.h>
#include <ctype.h>
void stringToLower(char *str) {
for (int i = 0; str[i]; i++) {
str[i] = tolower((unsigned char)str[i]);
}
}
int main() {
char str[] = "Hello, World!";
stringToLower(str);
printf("Lowercase string: %s\n", str);
return 0;
}
In this example, we define a function stringToLower()
that takes a string as an argument and converts it to lowercase character by character using tolower()
. The tolower()
function is used to convert each character to its lowercase equivalent. This approach allows you to convert an entire string to lowercase in a portable manner.
Keep in mind that tolower()
expects an int
argument and should be used with (unsigned char)
typecast to handle all possible character values correctly, including the special character EOF
and non-ASCII characters.