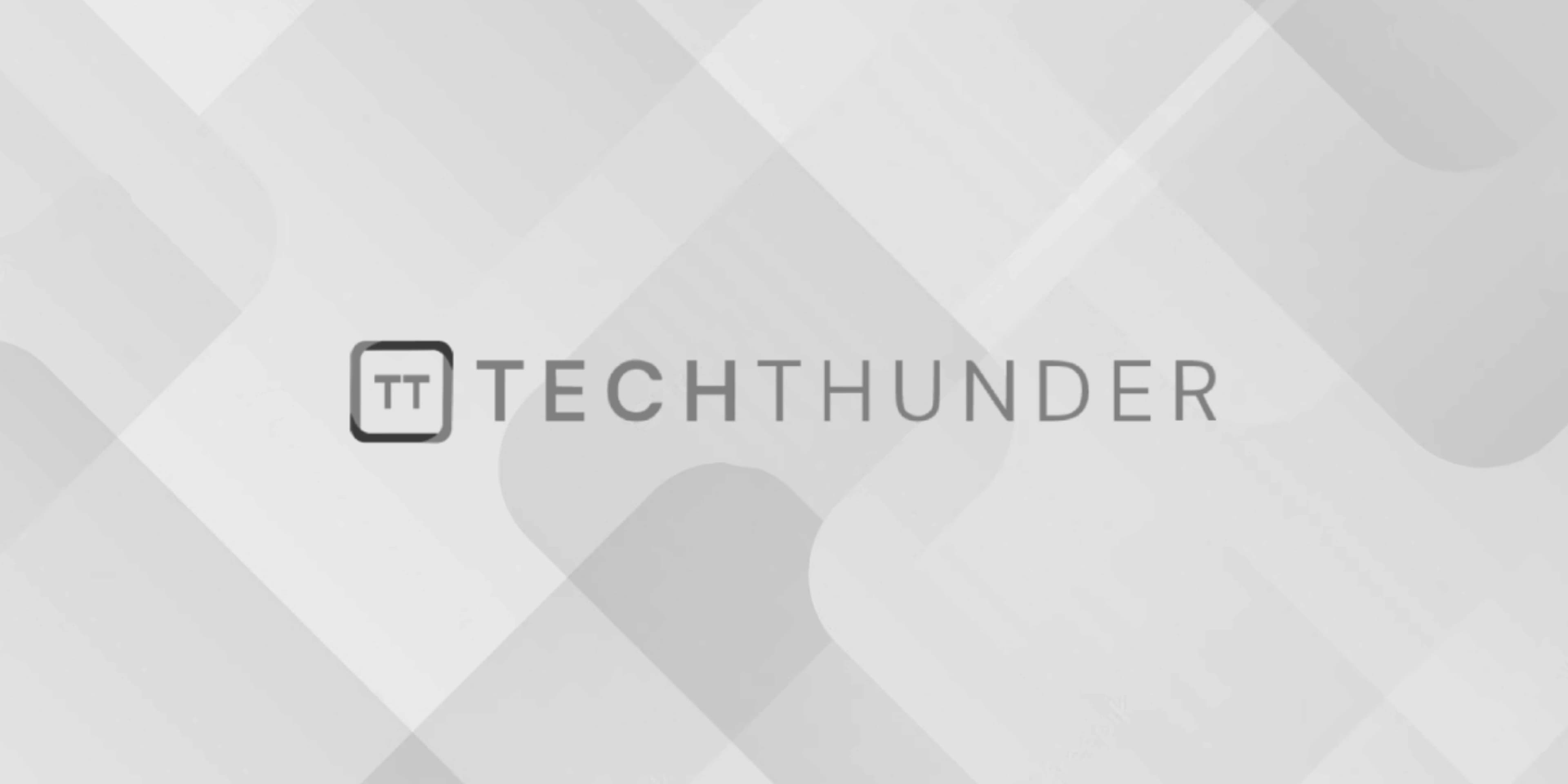
Algorithm in C language
The C language, an algorithm is a step-by-step procedure for solving a problem or performing a specific task. Algorithms in C are typically implemented as functions or blocks of code that outline the logic and operations required to achieve a particular objective. Here is a general structure of an algorithm in C:
1. Start
2. Declare variables and initialize them if necessary.
3. Input data if required (e.g., user input).
4. Perform computations and logical operations.
5. Output results.
6. End
Here’s an example of a simple algorithm in C that calculates the sum of two numbers entered by the user:
#include <stdio.h>
int main() {
// Step 1: Start
int num1, num2, sum;
// Step 2: Declare variables
// Step 3: Input data
printf("Enter the first number: ");
scanf("%d", &num1);
printf("Enter the second number: ");
scanf("%d", &num2);
// Step 4: Perform computations
sum = num1 + num2;
// Step 5: Output results
printf("Sum of %d and %d is %d\n", num1, num2, sum);
// Step 6: End
return 0;
}
In this example:
- Step 1 initializes the program.
- Step 2 declares the variables
num1
,num2
, andsum
. - Step 3 inputs data from the user using
scanf
. - Step 4 performs the addition operation.
- Step 5 outputs the result using
printf
. - Step 6 ends the program.
This is a simple illustration of an algorithm in C. In more complex programs, algorithms can involve loops, conditionals, functions, and more, and they are used to solve a wide range of problems from simple calculations to complex data processing and manipulation.
Remember that writing clear and well-documented algorithms is important for program readability and maintainability, especially in larger software projects.