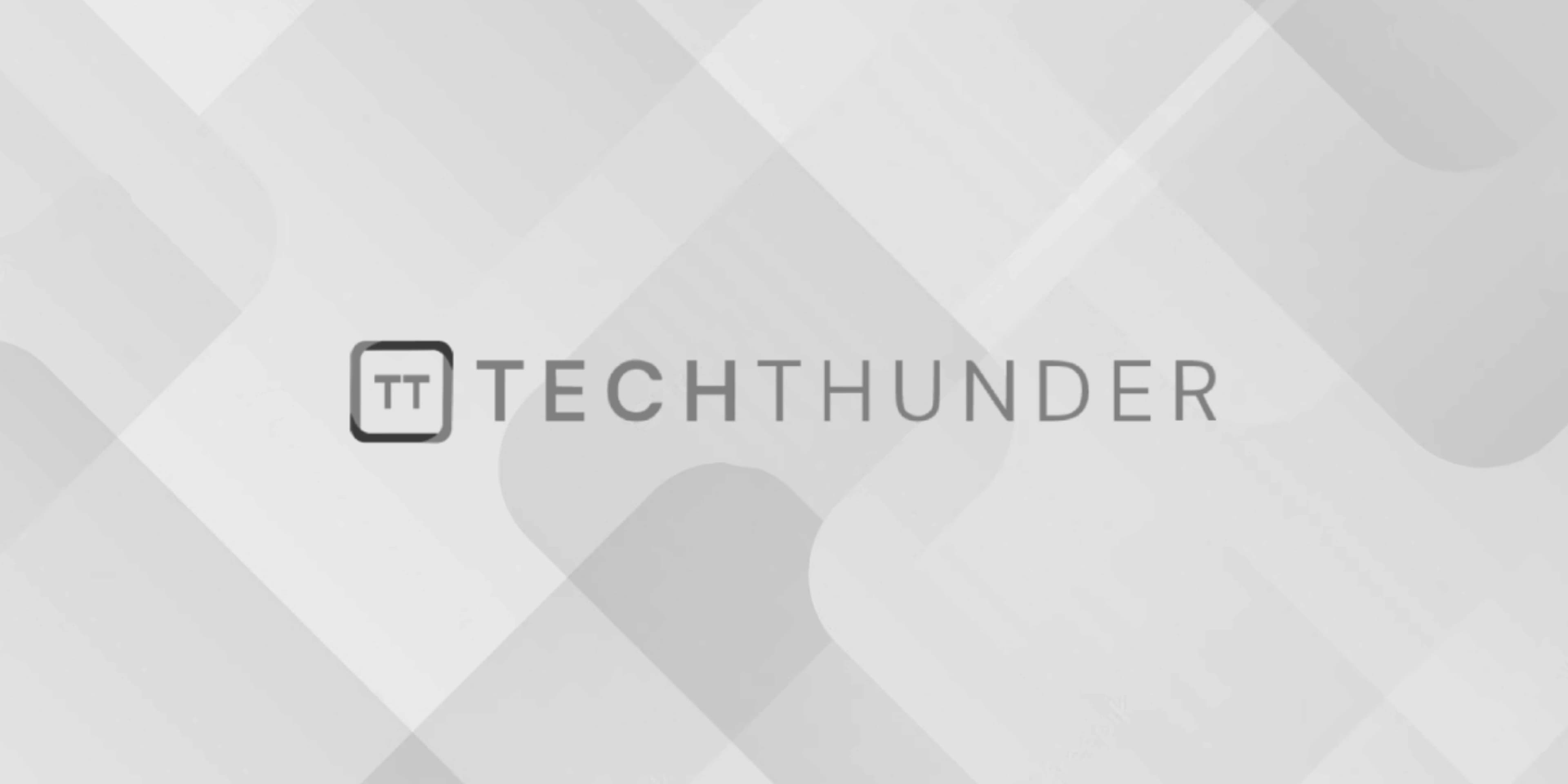
127 views
Add Digits of Number in C
To add the digits of a number in C, you can extract each digit one by one and accumulate their sum. Here’s a C program to add the digits of a given number:
C
#include <stdio.h>
int main() {
int num, originalNum, remainder, sum = 0;
// Input the number
printf("Enter an integer: ");
scanf("%d", &num);
originalNum = num;
// Calculate the sum of digits
while (num != 0) {
remainder = num % 10; // Get the last digit
sum += remainder; // Add the digit to the sum
num /= 10; // Remove the last digit
}
// Display the sum
printf("Sum of digits of %d = %d\n", originalNum, sum);
return 0;
}
In this program:
- We input an integer (
num
) from the user. - We create a variable
originalNum
to store the original number for later display. - We use a
while
loop to repeatedly extract the last digit ofnum
, add it to thesum
, and remove the last digit by dividingnum
by 10. - The loop continues until
num
becomes 0, indicating that all digits have been processed. - Finally, we display the sum of the digits.
For example, if the user enters the number 12345, the program will calculate and display the sum of its digits, which is 1 + 2 + 3 + 4 + 5 = 15.