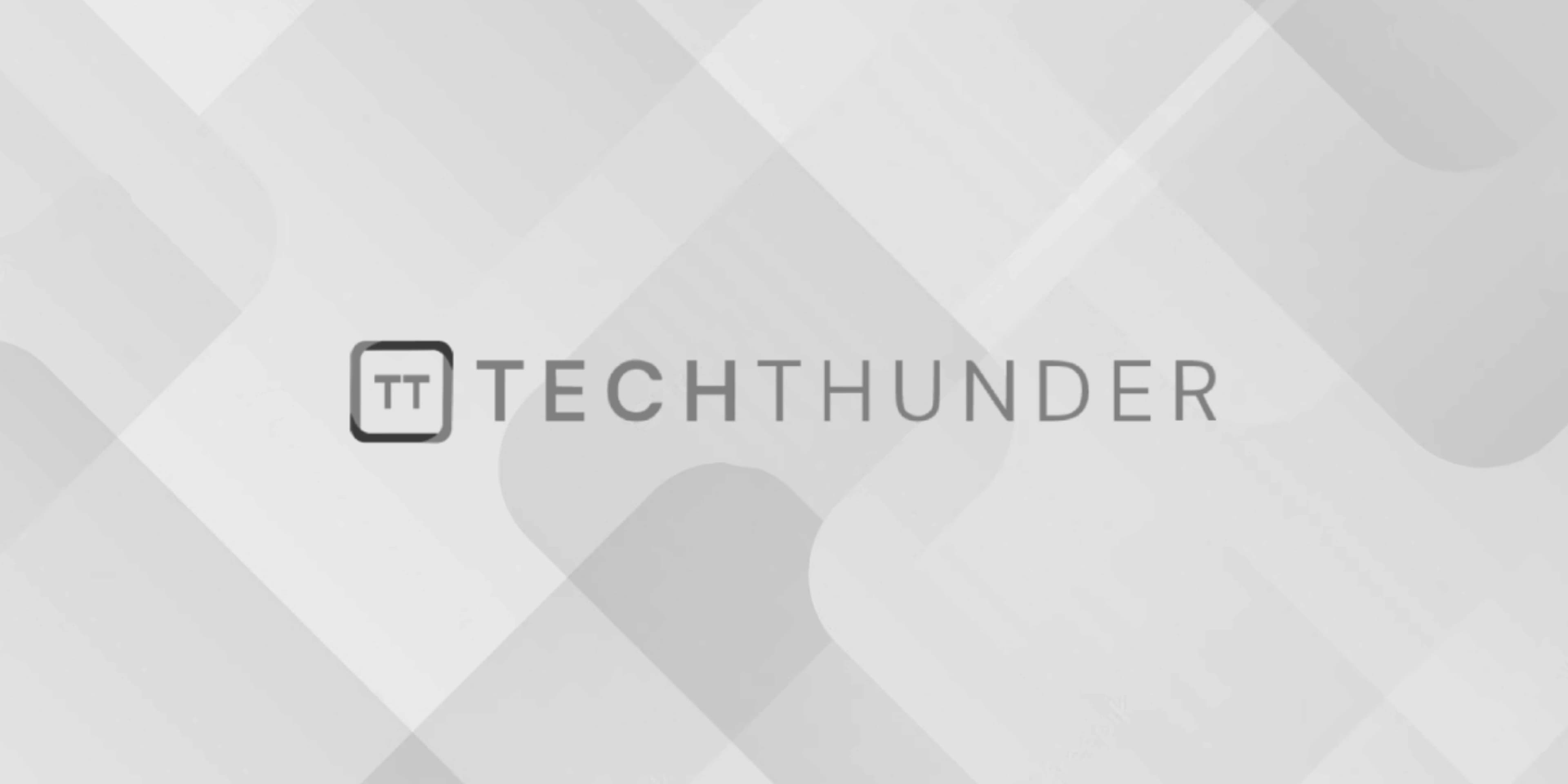
157 views
School Billing System in C
Creating a basic school billing system in C involves managing student records, fees, and generating bills. Here’s a simplified example of how you can implement such a system:
C
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// Structure to represent student information
struct Student {
int rollNumber;
char name[100];
double feesPaid;
double totalFees;
};
// Function to create a new student record
void createStudent() {
struct Student newStudent;
printf("Enter Roll Number: ");
scanf("%d", &newStudent.rollNumber);
printf("Enter Name: ");
scanf("%s", newStudent.name);
printf("Enter Total Fees: ");
scanf("%lf", &newStudent.totalFees);
newStudent.feesPaid = 0;
FILE *file = fopen("students.txt", "a");
if (file == NULL) {
perror("Error opening file");
exit(1);
}
fprintf(file, "%d %s %.2lf %.2lf\n", newStudent.rollNumber, newStudent.name, newStudent.totalFees, newStudent.feesPaid);
fclose(file);
printf("Student record created successfully.\n");
}
// Function to update fees payment for a student
void updateFees() {
int rollNumber;
double paymentAmount;
printf("Enter Roll Number: ");
scanf("%d", &rollNumber);
printf("Enter Payment Amount: ");
scanf("%lf", &paymentAmount);
FILE *file = fopen("students.txt", "r+");
if (file == NULL) {
perror("Error opening file");
exit(1);
}
struct Student student;
int found = 0;
while (fscanf(file, "%d %s %lf %lf", &student.rollNumber, student.name, &student.totalFees, &student.feesPaid) != EOF) {
if (student.rollNumber == rollNumber) {
if (student.feesPaid + paymentAmount <= student.totalFees) {
student.feesPaid += paymentAmount;
fseek(file, -sizeof(student), SEEK_CUR);
fprintf(file, "%d %s %.2lf %.2lf", student.rollNumber, student.name, student.totalFees, student.feesPaid);
found = 1;
break;
} else {
printf("Payment amount exceeds total fees.\n");
found = 1;
break;
}
}
}
fclose(file);
if (found) {
printf("Payment updated successfully.\n");
} else {
printf("Student not found.\n");
}
}
// Function to generate a bill for a student
void generateBill() {
int rollNumber;
printf("Enter Roll Number: ");
scanf("%d", &rollNumber);
FILE *file = fopen("students.txt", "r");
if (file == NULL) {
perror("Error opening file");
exit(1);
}
struct Student student;
int found = 0;
while (fscanf(file, "%d %s %lf %lf", &student.rollNumber, student.name, &student.totalFees, &student.feesPaid) != EOF) {
if (student.rollNumber == rollNumber) {
double remainingFees = student.totalFees - student.feesPaid;
printf("Student Name: %s\n", student.name);
printf("Total Fees: %.2lf\n", student.totalFees);
printf("Fees Paid: %.2lf\n", student.feesPaid);
printf("Remaining Fees: %.2lf\n", remainingFees);
found = 1;
break;
}
}
fclose(file);
if (!found) {
printf("Student not found.\n");
}
}
int main() {
int choice;
while (1) {
printf("\nSchool Billing System Menu:\n");
printf("1. Create Student Record\n");
printf("2. Update Fees Payment\n");
printf("3. Generate Bill\n");
printf("4. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
createStudent();
break;
case 2:
updateFees();
break;
case 3:
generateBill();
break;
case 4:
exit(0);
default:
printf("Invalid choice. Please try again.\n");
}
}
return 0;
}
In this code, we’ve created a simple school billing system where you can create student records, update fees payments, and generate bills. Student data is stored in a text file named “students.txt.” This example is simplified and doesn’t include advanced features such as error handling or data validation, which you would typically find in a production system. For a more robust and secure implementation, additional features and safeguards should be added.