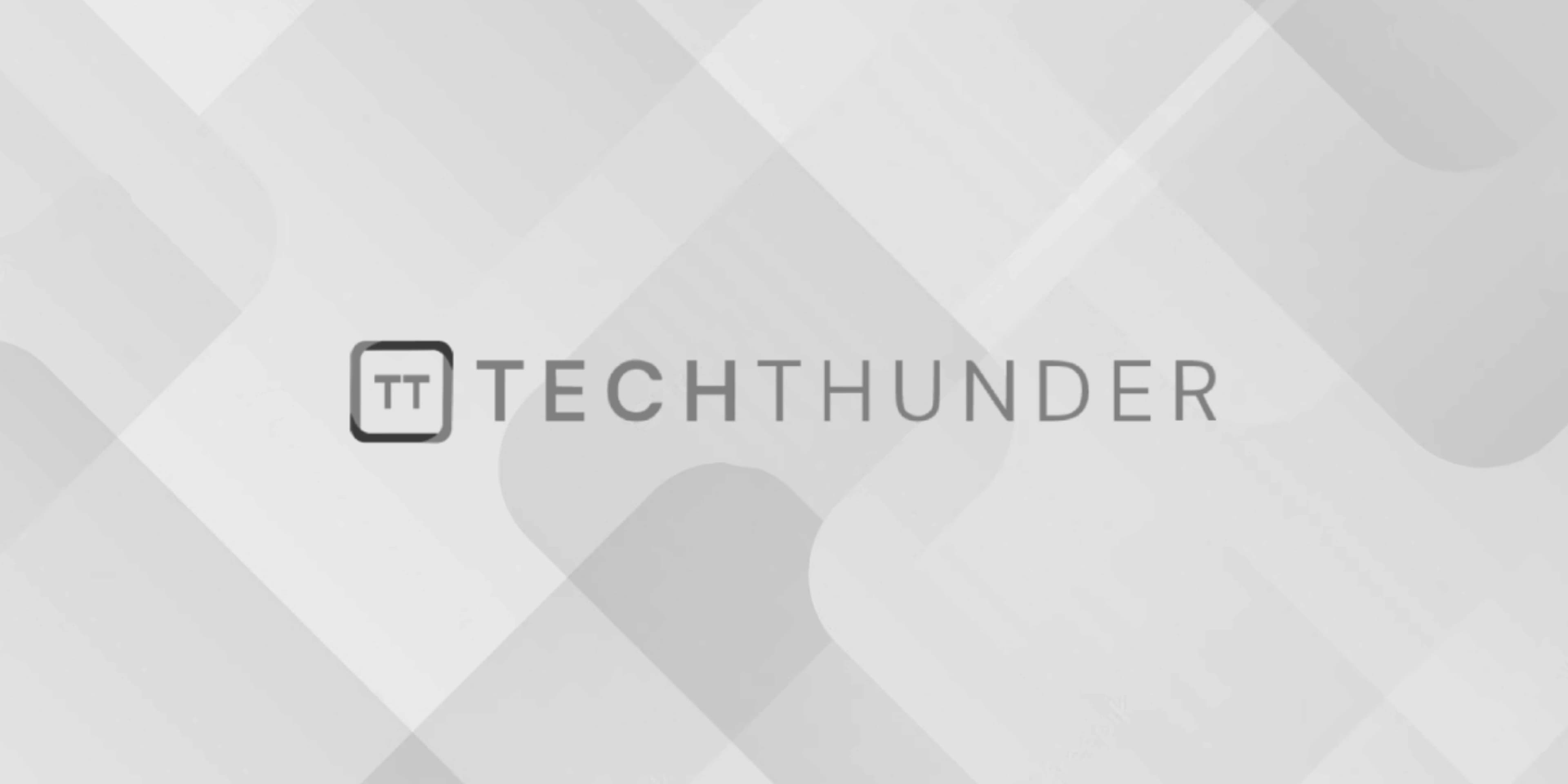
143 views
Reverse an Array in C
To reverse an array in C, you can swap elements from the beginning of the array with elements from the end of the array until you reach the middle of the array. Here’s a C program to reverse an array:
C
#include <stdio.h>
void reverseArray(int arr[], int start, int end) {
while (start < end) {
// Swap the elements at the start and end positions
int temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
start++;
end--;
}
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int n = sizeof(arr) / sizeof(arr[0]);
printf("Original Array: ");
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
// Reverse the array
reverseArray(arr, 0, n - 1);
printf("Reversed Array: ");
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
In this program:
- The
reverseArray
function takes an arrayarr
, a starting indexstart
, and an ending indexend
. It swaps elements atstart
andend
, then movesstart
one step to the right andend
one step to the left. This process is repeated untilstart
is greater than or equal toend
. - In the
main
function, we initialize an array, print the original array, callreverseArray
to reverse the array, and then print the reversed array.
When you run this program, it will reverse the elements of the array, resulting in the reversed order.