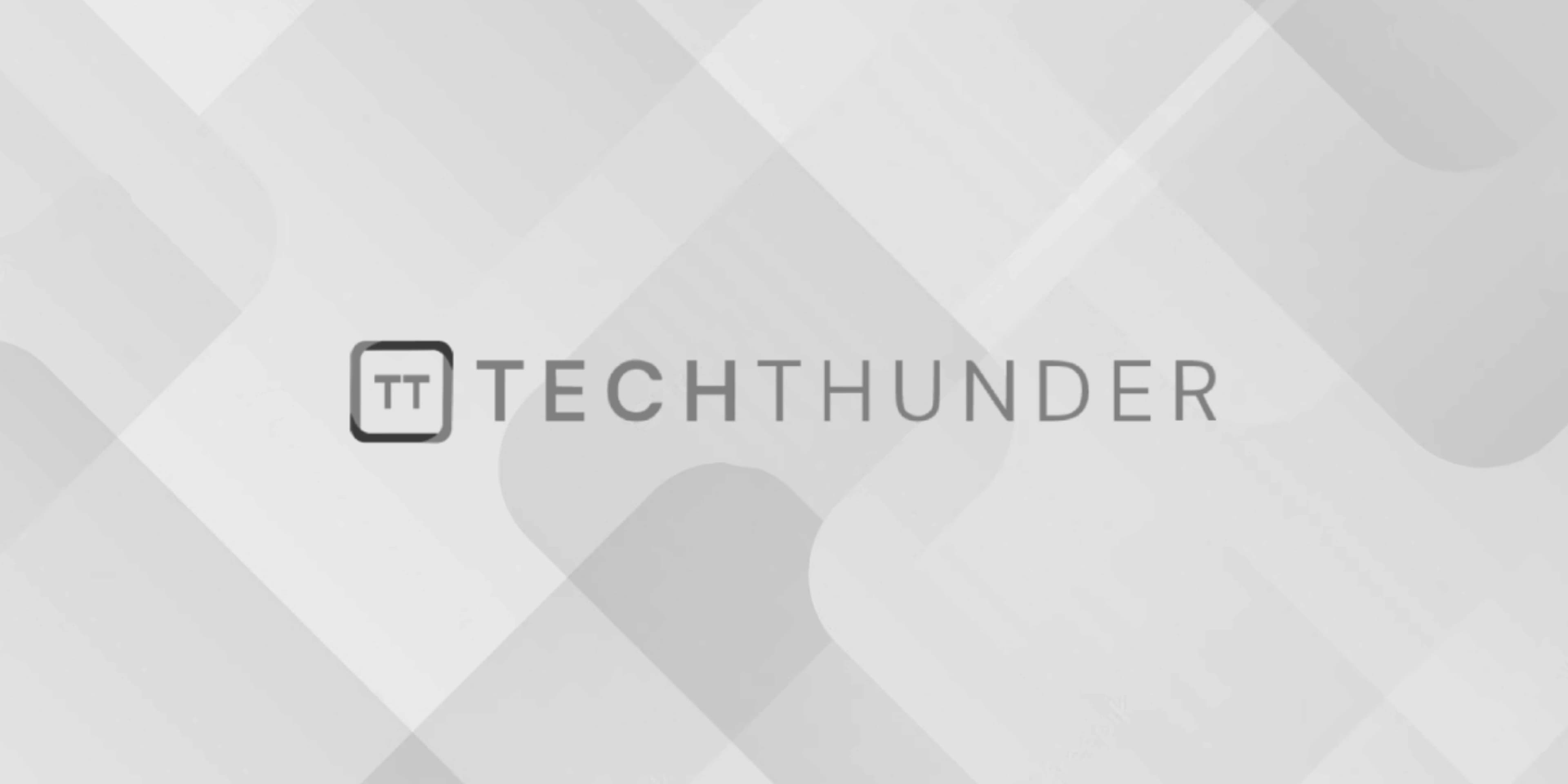
267 views
Bit Fields in C
The bit fields are a way to specify the number of bits used to store a particular data member within a structure. They allow you to optimize memory usage by packing multiple smaller data members into a single storage unit, typically an integer or other integral type. Bit fields are declared within a structure or union definition and specify the number of bits allocated for each data member. Here’s how bit fields work in C:
C
#include <stdio.h>
// Define a structure with bit fields
struct Flags {
unsigned int isOn : 1; // 1 bit for isOn (0 or 1)
unsigned int isHigh : 1; // 1 bit for isHigh (0 or 1)
unsigned int brightness : 6; // 6 bits for brightness (0 to 63)
};
int main() {
struct Flags lamp;
// Assign values to bit fields
lamp.isOn = 1; // Set isOn to 1
lamp.isHigh = 0; // Set isHigh to 0
lamp.brightness = 42; // Set brightness to 42
// Access and print bit fields
printf("isOn: %u\n", lamp.isOn);
printf("isHigh: %u\n", lamp.isHigh);
printf("brightness: %u\n", lamp.brightness);
return 0;
}
In this example:
- We define a structure called
Flags
with three bit fields:isOn
,isHigh
, andbrightness
. - Each bit field specifies the number of bits allocated for that member. For example,
isOn
andisHigh
each use 1 bit, whilebrightness
uses 6 bits. - We create a variable
lamp
of typestruct Flags
. - We assign values to the bit fields using simple assignment statements.
- We access and print the values of the bit fields.
Important points about bit fields in C:
- Bit Order: The order in which bits are stored within a bit field may be platform-dependent. Some systems store the most significant bit (MSB) on the left, while others store the least significant bit (LSB) on the left. You should be cautious about bit order when working with external data or hardware.
- Bit Field Size: The size of a bit field must be less than or equal to the size of the underlying data type (e.g.,
int
). In the example above,brightness
uses 6 bits, which is within the range of anint
. - Bit Fields and Portability: The use of bit fields can make your code less portable across different compilers and platforms because their behavior may vary. It’s essential to be aware of the specific behavior of bit fields on the target platform.
- Bit Fields and Efficiency: Bit fields can be used to optimize memory usage when dealing with data structures where space is a concern, such as in embedded systems or when working with hardware registers.
Bit fields can be a useful tool for managing memory efficiently in certain situations, but they should be used with caution, especially when portability is a concern.