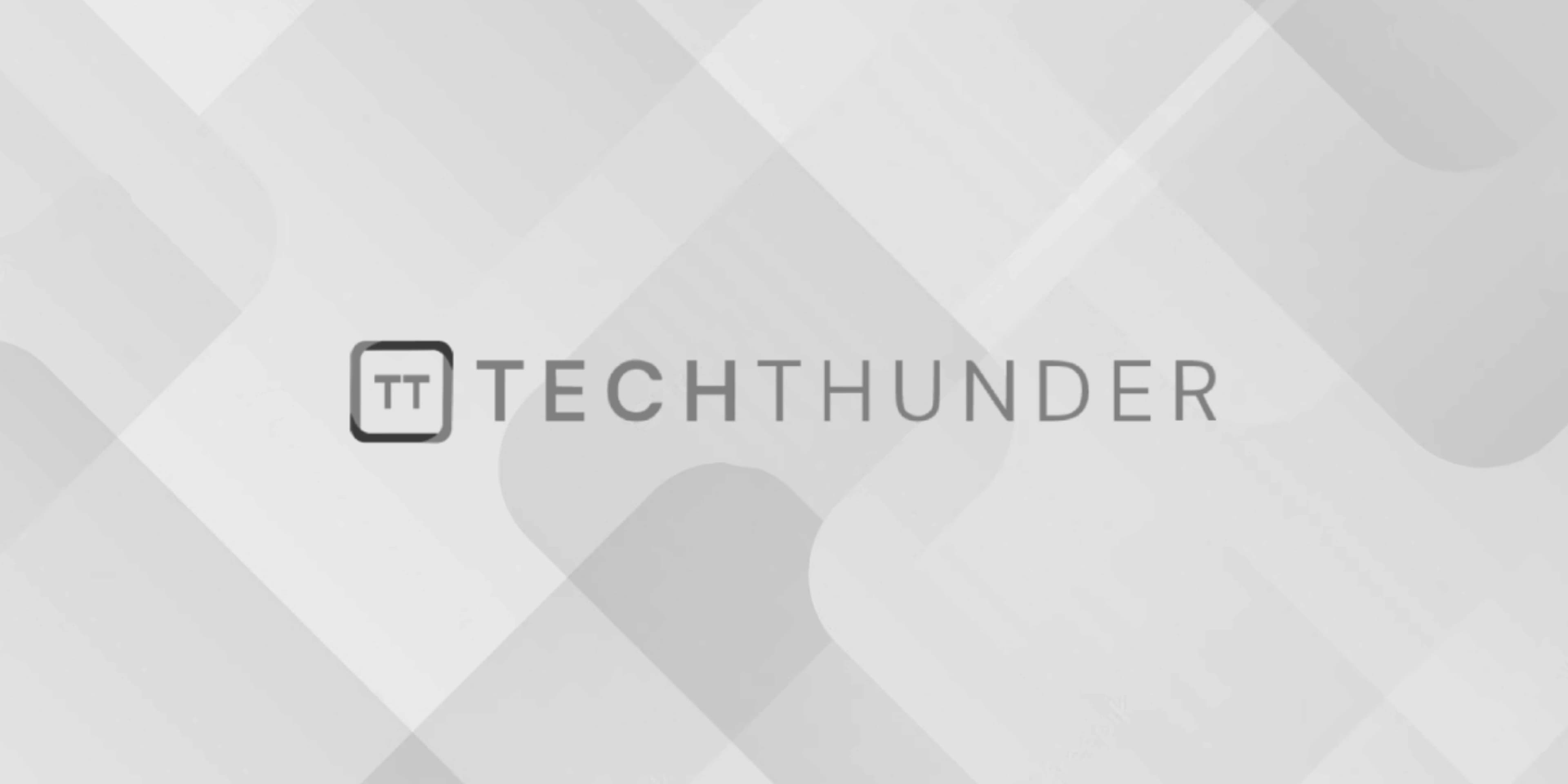
180 views
An Array of Strings in C
The C programming to create an array of strings by declaring an array of character arrays (strings) where each element is a character array representing a string. Here’s an example of how to create and use an array of strings:
C
#include <stdio.h>
#include <string.h>
int main() {
// Declare an array of strings
char strings[5][50]; // Array of 5 strings, each with a maximum length of 50 characters
// Initialize the array with strings
strcpy(strings[0], "Hello");
strcpy(strings[1], "World");
strcpy(strings[2], "C");
strcpy(strings[3], "Programming");
strcpy(strings[4], "Example");
// Access and print the strings
for (int i = 0; i < 5; i++) {
printf("String %d: %s\n", i + 1, strings[i]);
}
return 0;
}
In this example:
- We declare an array
strings
of size 5, where each element is a character array (string) with a maximum length of 50 characters. You can adjust the size and maximum length to fit your requirements. - We initialize the array by using the
strcpy
function to copy strings into each element of the array. - We access and print the strings using a loop.
You can modify the number of strings, their lengths, and the contents according to your specific needs. Just make sure that the maximum length specified for each string can accommodate the longest string you intend to store in that element.