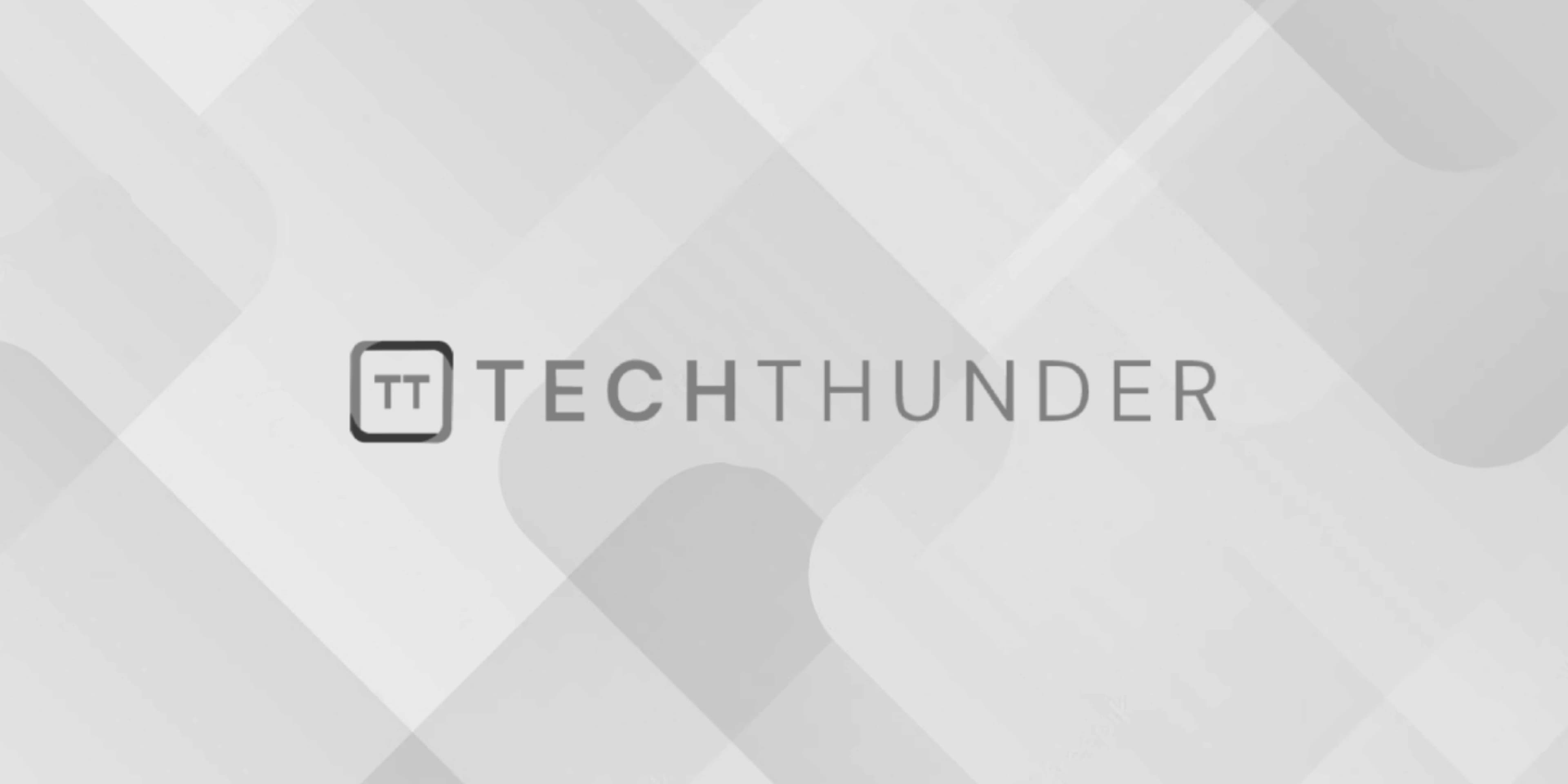
C strlen()
The strlen()
is a standard library function used to calculate the length of a null-terminated string. It’s part of the <string.h>
header and is commonly used for string manipulation in C programs. The length of a string is defined as the number of characters in the string, excluding the null character (‘\0’).
Here’s the syntax of strlen()
:
size_t strlen(const char *str);
str
: A pointer to the null-terminated string for which you want to calculate the length.
The strlen()
function counts the characters in the string pointed to by str
until it reaches the null character. It returns the length of the string as a value of type size_t
, which is an unsigned integer type.
Here’s an example of using strlen()
:
#include <stdio.h>
#include <string.h>
int main() {
const char str[] = "Hello, World!";
size_t length = strlen(str);
printf("Length of the string: %zu\n", length);
return 0;
}
In this example, we declare a str
string containing “Hello, World!” and use strlen()
to calculate its length. The result is then printed to the console.
The strlen()
function is often used when working with strings to determine their length before performing various string operations. Keep in mind that it counts the characters up to, but not including, the null character, so the length of the string “Hello” is 5 (not 6, since it doesn’t include the null character at the end).