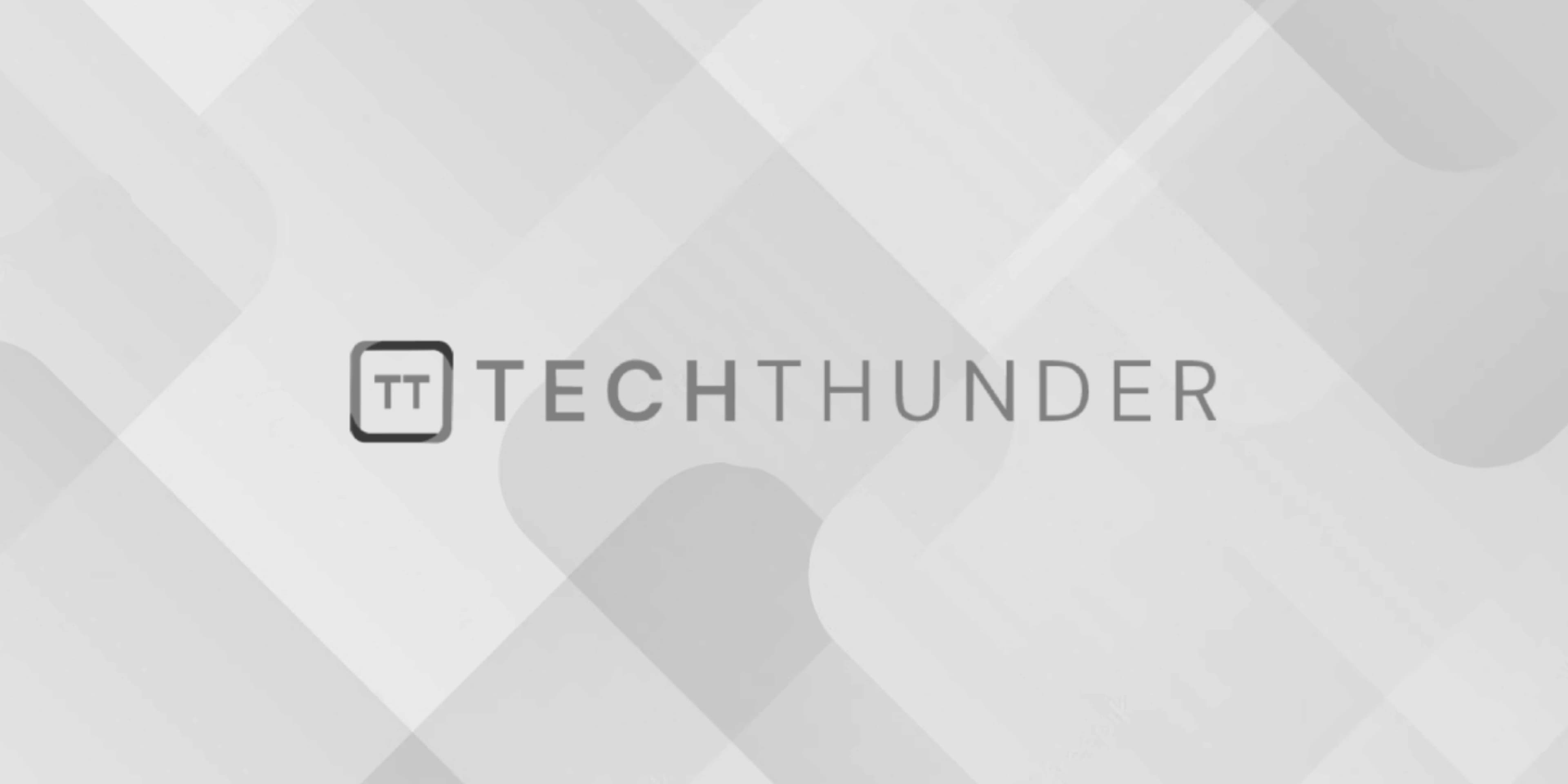
C Comments
The C programming, comments are text within the source code that is not meant to be compiled or executed as part of the program. Comments are added to provide explanations, documentation, and annotations to make the code more understandable to humans, including the programmers who read and maintain the code. C supports two types of comments:
- Single-Line Comments: These comments are used to document a single line of code or provide a brief explanation. In C, single-line comments are created using the
//
symbol, and everything following//
on the same line is treated as a comment and ignored by the compiler.
// This is a single-line comment
int num = 42; // This is another single-line comment
- Multi-Line Comments (Block Comments): Multi-line comments are used to comment out multiple lines of code or to provide more extensive explanations. In C, multi-line comments are enclosed between
/*
and*/
, and everything between these delimiters is treated as a comment and ignored by the compiler.
/*
This is a multi-line comment.
It can span multiple lines and is often used for detailed explanations.
*/
int x = 10; /* This is another example of a multi-line comment */
Comments are an essential part of writing maintainable code because they provide context and explanations for what the code is doing. They can also help other programmers (including your future self) understand the purpose and functionality of specific code sections. It’s a good practice to include comments in your code to make it more readable and easier to maintain.
Here are some additional tips for using comments effectively:
- Use comments to explain complex or non-obvious code.
- Comment code that may need to be revisited or modified in the future.
- Document function and variable declarations, including their purpose and expected usage.
- Avoid excessive commenting; comments should add value and not clutter the code unnecessarily.
- Keep comments up to date when you make changes to the code to ensure they remain accurate and helpful.