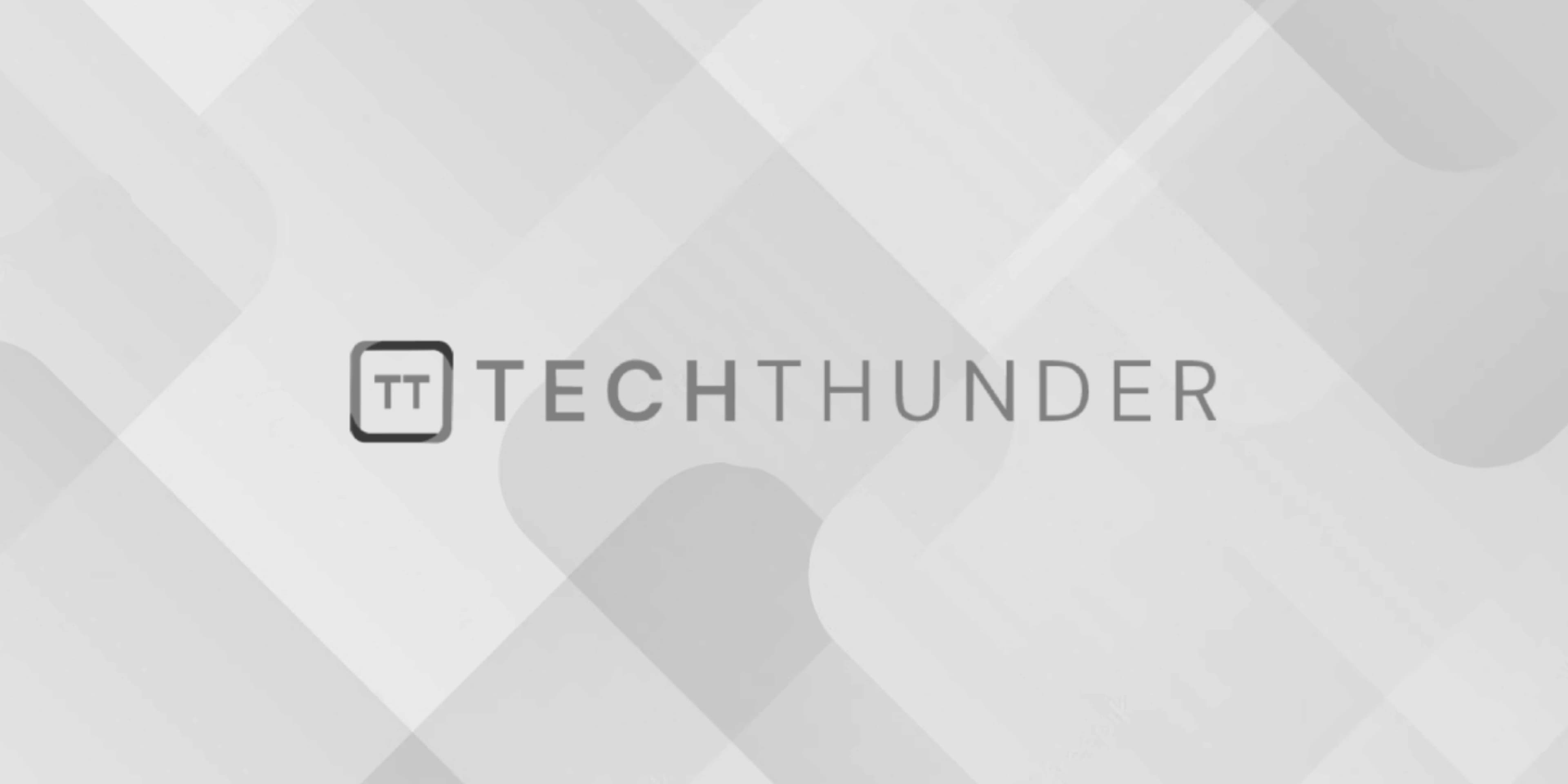
111 views
Student Record System in C
A student record system in C allows you to manage student data, including information such as student ID, name, grades, and other relevant details. Below is a basic implementation of a student record system in C:
C
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STUDENTS 100
// Structure to represent a student
struct Student {
int id;
char name[100];
float grade;
};
// Function to add a new student
void addStudent(struct Student students[], int *studentCount) {
if (*studentCount >= MAX_STUDENTS) {
printf("Student database is full. Cannot add more students.\n");
return;
}
struct Student newStudent;
printf("Enter Student ID: ");
scanf("%d", &newStudent.id);
printf("Enter Student Name: ");
scanf("%s", newStudent.name);
printf("Enter Student Grade: ");
scanf("%f", &newStudent.grade);
students[*studentCount] = newStudent;
(*studentCount)++;
printf("Student added successfully.\n");
}
// Function to display all students
void displayStudents(struct Student students[], int studentCount) {
if (studentCount == 0) {
printf("No students to display.\n");
return;
}
printf("ID\tName\tGrade\n");
for (int i = 0; i < studentCount; i++) {
printf("%d\t%s\t%.2f\n", students[i].id, students[i].name, students[i].grade);
}
}
int main() {
struct Student students[MAX_STUDENTS];
int studentCount = 0;
int choice;
while (1) {
printf("\nStudent Record System Menu:\n");
printf("1. Add Student\n");
printf("2. Display Students\n");
printf("3. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
addStudent(students, &studentCount);
break;
case 2:
displayStudents(students, studentCount);
break;
case 3:
exit(0);
default:
printf("Invalid choice. Please try again.\n");
}
}
return 0;
}
In this code, we’ve created a simple student record system with the following features:
- You can add new students, including their ID, name, and grade.
- You can display the details of all students in the system.
- The system uses an array of structures (
struct Student
) to store student data. - The maximum number of students is defined by
MAX_STUDENTS
. - The program provides a menu to interact with the system, allowing you to add students, display student records, and exit the program.
This is a basic implementation for educational purposes. In a real-world scenario, you may want to enhance the system with error handling, data validation, the ability to edit or delete student records, and data persistence (e.g., saving and loading student data from files).