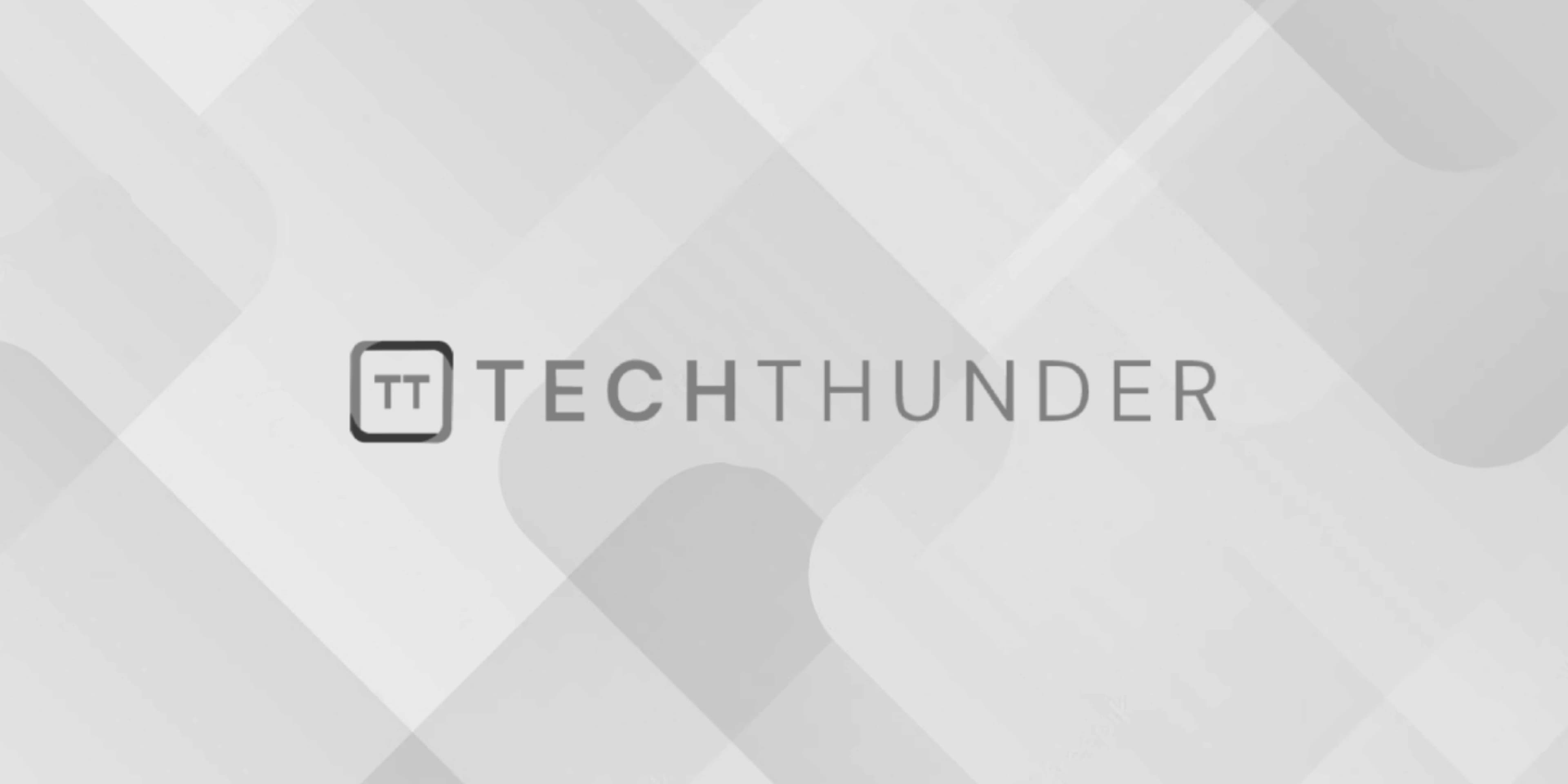
337 views
C Loops
The loops are control flow constructs that allow you to execute a block of code repeatedly as long as a certain condition is true. There are three primary types of loops in C: for
, while
, and do-while
. These loops serve different purposes and are used depending on the specific requirements of your program.
- For Loop:
- The
for
loop is used when you know in advance how many times you want to execute a block of code. - It consists of three parts: initialization, condition, and increment/decrement.
- The loop initializes a variable, checks a condition, and updates the variable at the end of each iteration.
C
for (initialization; condition; increment/decrement) {
// Code to execute repeatedly
}
Example:
C
for (int i = 0; i < 5; i++) {
printf("Iteration %d\n", i);
}
- While Loop:
- The
while
loop is used when you want to execute a block of code as long as a condition is true. - It checks the condition before each iteration and continues until the condition becomes false.
C
while (condition) {
// Code to execute repeatedly
}
Example:
C
int count = 0;
while (count < 5) {
printf("Iteration %d\n", count);
count++;
}
- Do-While Loop:
- The
do-while
loop is similar to thewhile
loop, but it guarantees that the block of code is executed at least once, even if the condition is initially false. - It checks the condition at the end of each iteration.
C
do {
// Code to execute repeatedly
} while (condition);
Example:
C
int count = 0;
do {
printf("Iteration %d\n", count);
count++;
} while (count < 5);
Loops are essential for performing repetitive tasks, such as iterating through arrays, processing data, and implementing algorithms. It’s important to ensure that the loop condition eventually becomes false to avoid infinite loops. Additionally, you can use control statements like break
and continue
to control the flow within loops and exit or skip iterations when needed.