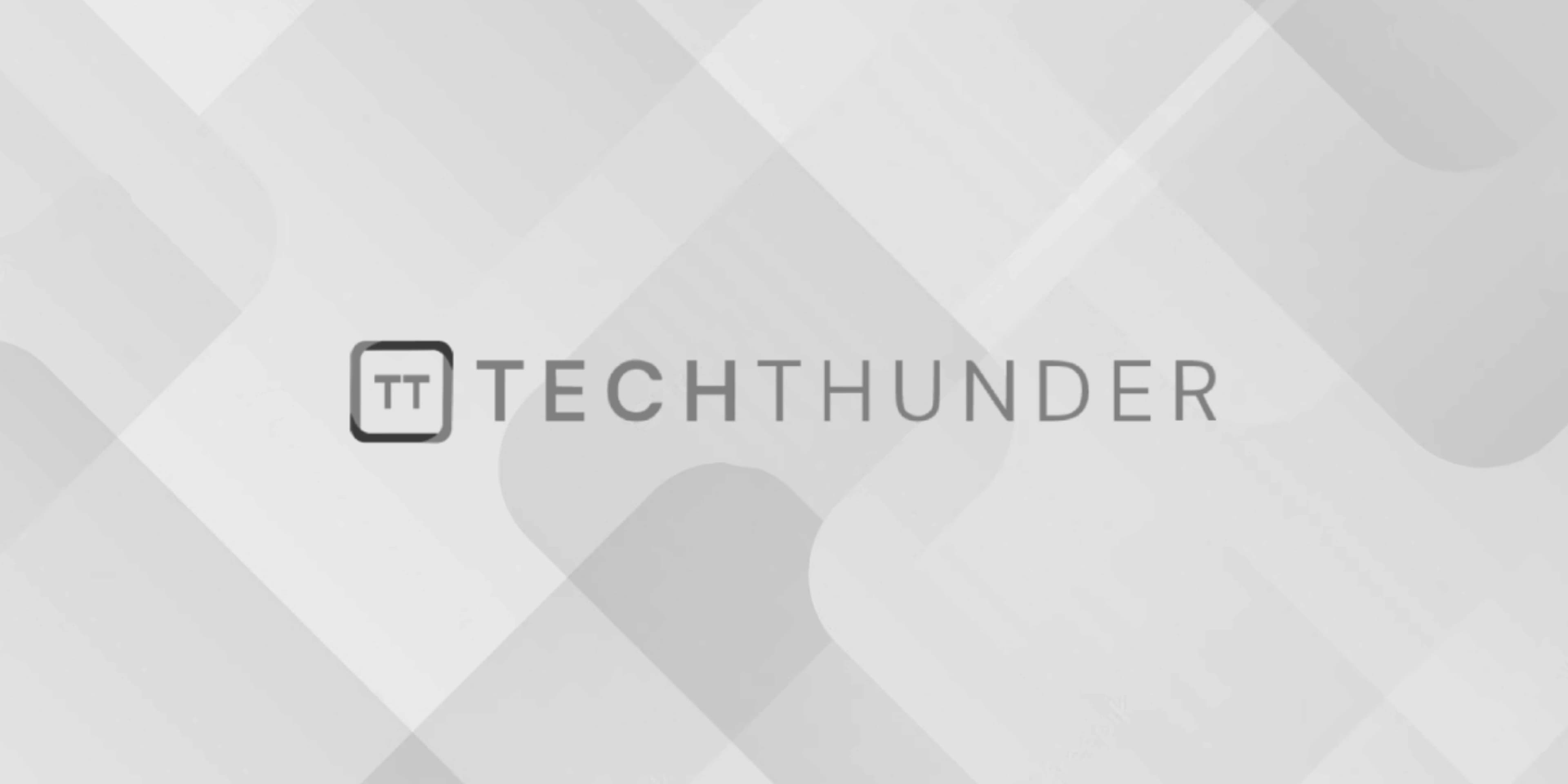
What is getch() in C
The getch()
is not a standard C library function. However, it is a function provided by some older compilers, especially those for DOS-based systems, like Turbo C and Borland C. The getch()
function is used for reading a single character from the keyboard without echoing it to the screen. It’s often used for implementing simple console-based menu systems and for reading single characters without requiring the user to press Enter.
Here’s a simple example of how getch()
can be used:
#include <stdio.h>
#include <conio.h> // This is specific to certain compilers, like Turbo C
int main() {
char ch;
printf("Press any key: ");
ch = getch(); // Read a single character without Enter
printf("\nYou pressed: %c\n", ch);
return 0;
}
In this example, we include the <conio.h>
header, which is specific to certain compilers, to access the getch()
function. The program prompts the user to press any key, and when a key is pressed, it reads that key without waiting for Enter and displays the character.
It’s important to note that getch()
is not part of the C standard library and is considered non-portable. Modern C compilers typically do not provide getch()
. If you are using a modern compiler or need portability, you should consider using standard library functions like getchar()
for character input, which does require the user to press Enter.