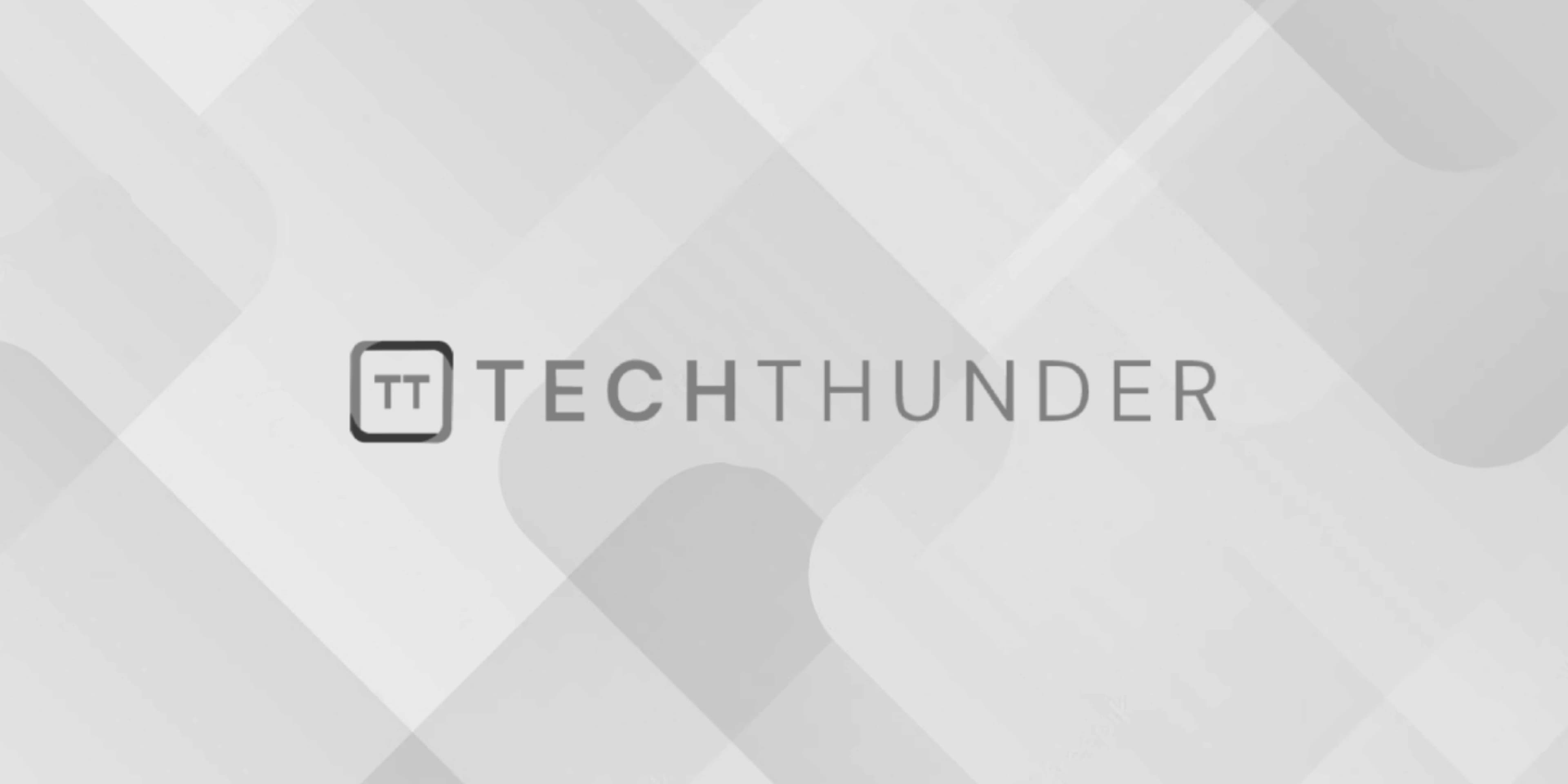
327 views
Strong number in C
The mathematics, a strong number (or strong factorial) is a special kind of number. A strong number is defined as follows:
- Start with a positive integer.
- Calculate the sum of the factorials of its individual digits.
- If the sum is equal to the original number, it is considered a strong number.
For example, the number 145 is a strong number because:
- 1! + 4! + 5! = 1 + 24 + 120 = 145
Here’s a C program to check if a given number is a strong number or not:
C
#include <stdio.h>
// Function to calculate the factorial of a number
int factorial(int n) {
if (n == 0 || n == 1) {
return 1;
} else {
return n * factorial(n - 1);
}
}
// Function to check if a number is a strong number
int isStrongNumber(int num) {
int originalNum = num;
int sum = 0;
while (num > 0) {
int digit = num % 10;
sum += factorial(digit);
num /= 10;
}
return (sum == originalNum);
}
int main() {
int num;
printf("Enter a positive integer: ");
scanf("%d", &num);
if (num <= 0) {
printf("Please enter a positive integer.\n");
} else {
if (isStrongNumber(num)) {
printf("%d is a strong number.\n", num);
} else {
printf("%d is not a strong number.\n", num);
}
}
return 0;
}
In this program:
- We have a
factorial
function to calculate the factorial of a number using recursion. - We have an
isStrongNumber
function that checks if a given number is a strong number. It iterates through the digits of the number, calculates the factorial of each digit, and sums them up. If the sum is equal to the original number, it returns1
(true); otherwise, it returns0
(false). - In the
main
function, we prompt the user to enter a positive integer and callisStrongNumber
to check if it’s a strong number. We then display the result.
Compile and run the program, and it will determine whether the entered number is a strong number or not based on the defined criteria.