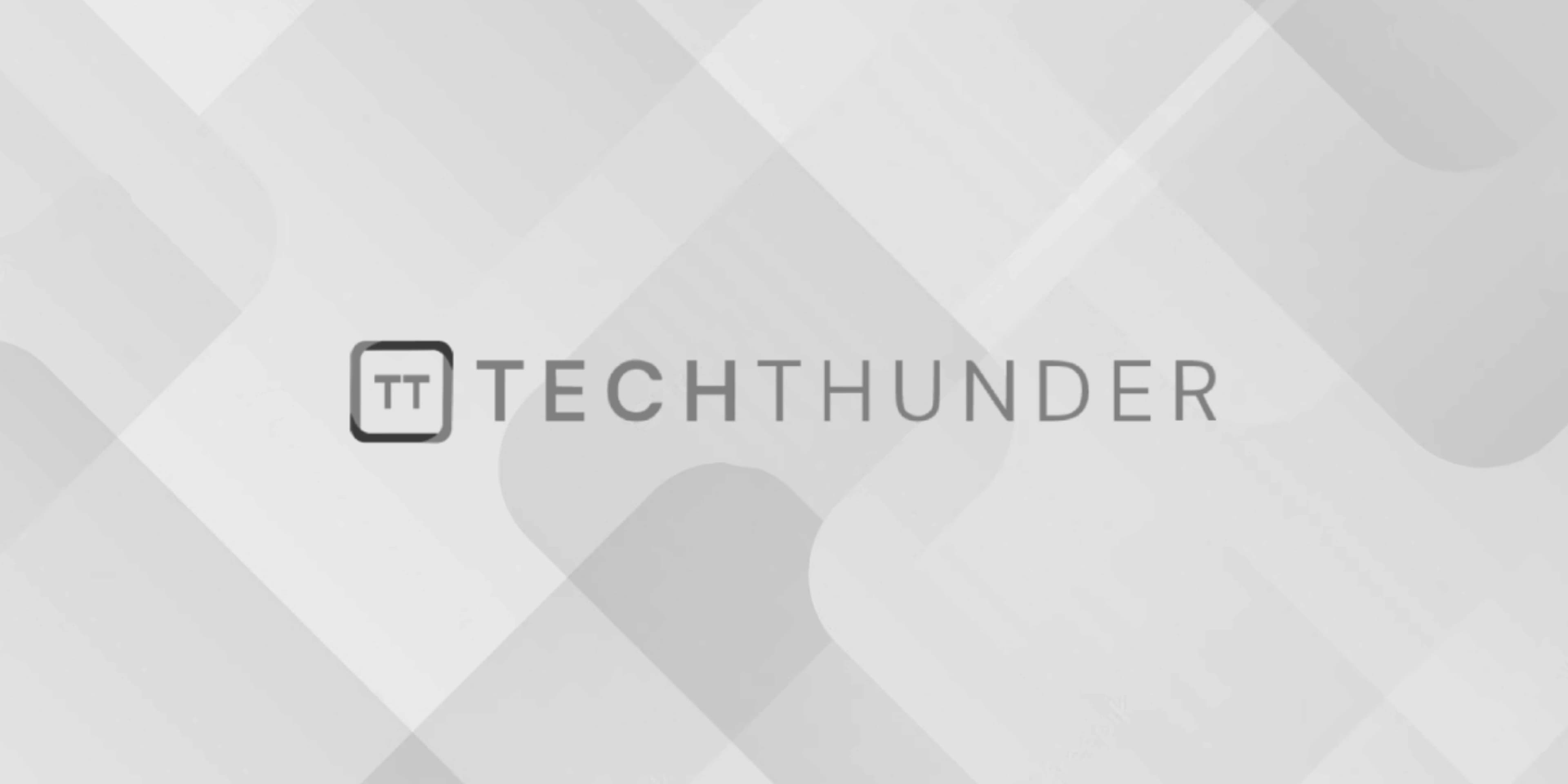
ASCII Table in C
An ASCII table is a reference table that maps characters to their corresponding ASCII (American Standard Code for Information Interchange) values. ASCII is a character encoding standard that assigns a unique numeric value (from 0 to 127) to each character, including letters, digits, punctuation marks, and control characters.
You can create a simple ASCII table in C by writing a program that prints the characters and their corresponding ASCII values. Here’s an example of how to do it:
#include <stdio.h>
int main() {
printf("ASCII Table:\n");
printf("---------------\n");
printf("Character ASCII Value\n");
printf("---------------\n");
for (int i = 0; i <= 127; i++) {
printf(" %c %d\n", i, i);
}
return 0;
}
In this program:
- We use a
for
loop to iterate from 0 to 127, inclusive, covering all the valid ASCII values. - Inside the loop, we use the
%c
format specifier to print the character corresponding to the ASCII valuei
, and we use%d
to print the ASCII value itself.
When you run this program, it will print an ASCII table that displays characters along with their ASCII values. The table will look something like this:
ASCII Table:
-----------------------
Character ASCII Value
-----------------------
N 78
O 79
P 80
Q 81
R 82
S 83
T 84
U 85
V 86
W 87
X 88
Y 89
Z 90
[ 91
\ 92
] 93
^ 94
_ 95
` 96
a 97
b 98
c 99
d 100
e 101
f 102
g 103
h 104
i 105
j 106
k 107
l 108
m 109
n 110
o 111
p 112
q 113
r 114
s 115
t 116
u 117
v 118
w 119
x 120
y 121
z 122
{ 123
| 124
} 125
~ 126
127
This table shows a subset of the ASCII characters and their corresponding values. ASCII values from 0 to 31 represent control characters, which may not be printable or displayable. ASCII values from 32 to 126 correspond to printable characters, including letters, digits, punctuation marks, and special symbols.