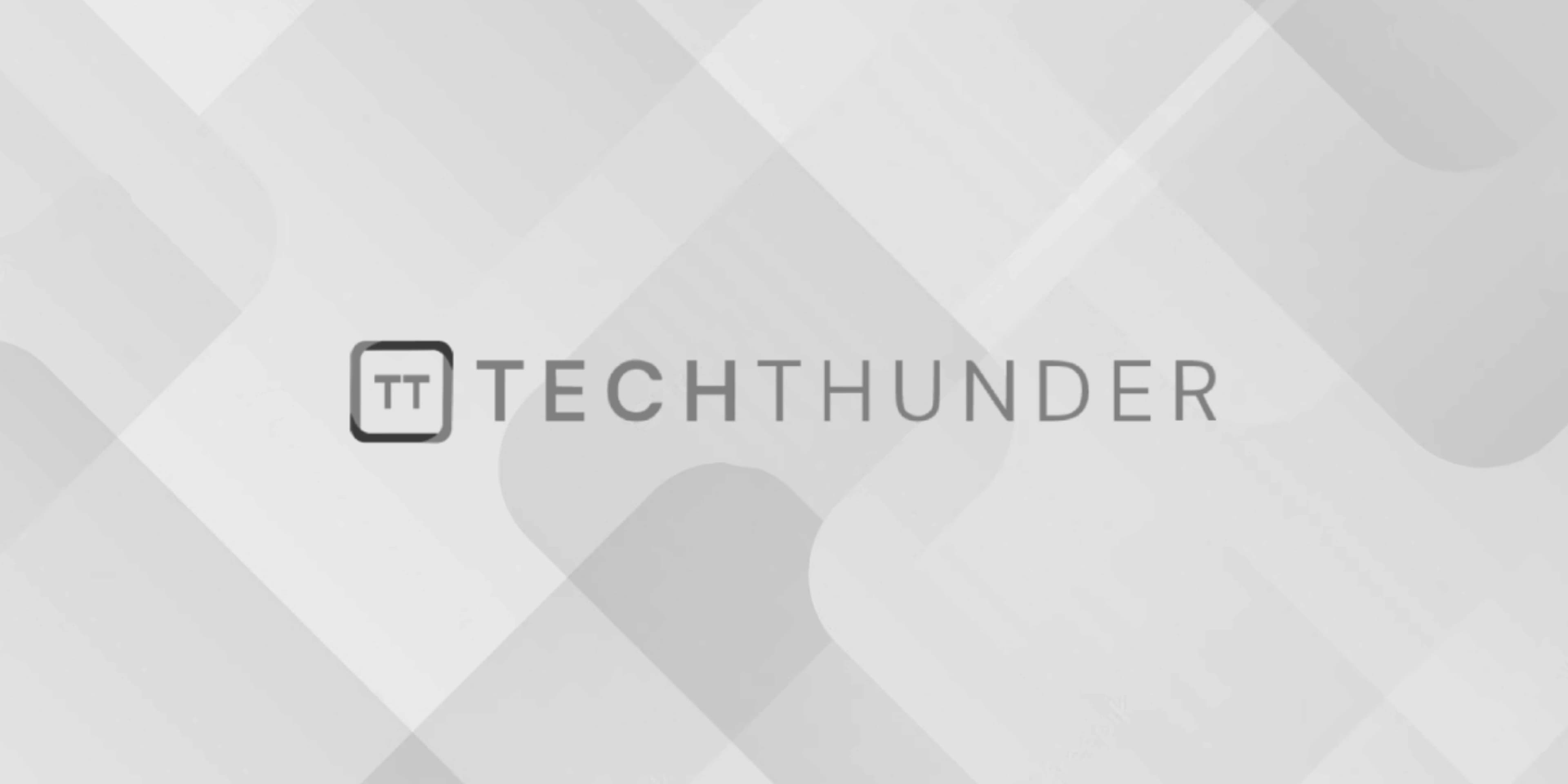
185 views
Flow of C Program
The flow of a C program refers to the sequence of actions that occur when the program is executed. C programs follow a typical flow, starting from the main
function and branching into various control structures and functions as needed. Here’s a general overview of the flow of a C program:
- Preprocessing Phase: Before compilation, the C preprocessor processes the source code. It performs tasks such as including header files, expanding macros, and handling conditional compilation using directives like
#include
,#define
,#ifdef
, and#ifndef
. - Compilation Phase: The preprocessed source code is then compiled by the C compiler. During this phase, the code is translated into machine code or an intermediate representation. Syntax and semantic errors are reported at this stage.
- Linking Phase: If your program consists of multiple source files or uses external libraries, the linker combines these files into a single executable. It resolves symbols and addresses, ensuring that functions and variables are properly connected.
- Execution Phase (Runtime): After successful compilation and linking, the program is executed. The program execution begins with the
main
function. main
Function: Themain
function is the entry point of the program. It’s where the program execution begins. You can think of it as the starting point of your program’s flow.- Control Structures: Within the
main
function and other user-defined functions, you use control structures likeif
,else
,while
,for
, andswitch
to control the flow of execution based on conditions and loops. - Function Calls: In a C program, functions are called to perform specific tasks. Function calls can be nested, and functions can call other functions. The program’s execution may jump to a called function and return to the calling function when the called function completes its task.
- Variables and Data: Variables are used to store data. Depending on their scope (local or global) and storage duration (automatic or static), they have different lifetimes.
- Input and Output: C programs often interact with the user or external data sources. Input can be read from the keyboard or files, and output can be displayed on the screen or written to files using functions like
printf
andscanf
. - Termination: The program execution continues until it reaches the end of the
main
function or until a termination statement, such asreturn
orexit
, is encountered. At this point, the program terminates, and resources are released. - Cleanup: If necessary, any cleanup operations, such as closing files or freeing dynamically allocated memory, should be performed before program termination.
- Exit Status: The program typically returns an exit status code to the operating system to indicate its completion status. A return value of 0 often indicates success, while non-zero values may indicate errors or other conditions.
The actual flow of a C program can vary widely depending on the specific logic and control structures implemented within the program. Control structures, function calls, and conditional statements determine how the program’s flow is directed based on input, conditions, and user interactions.