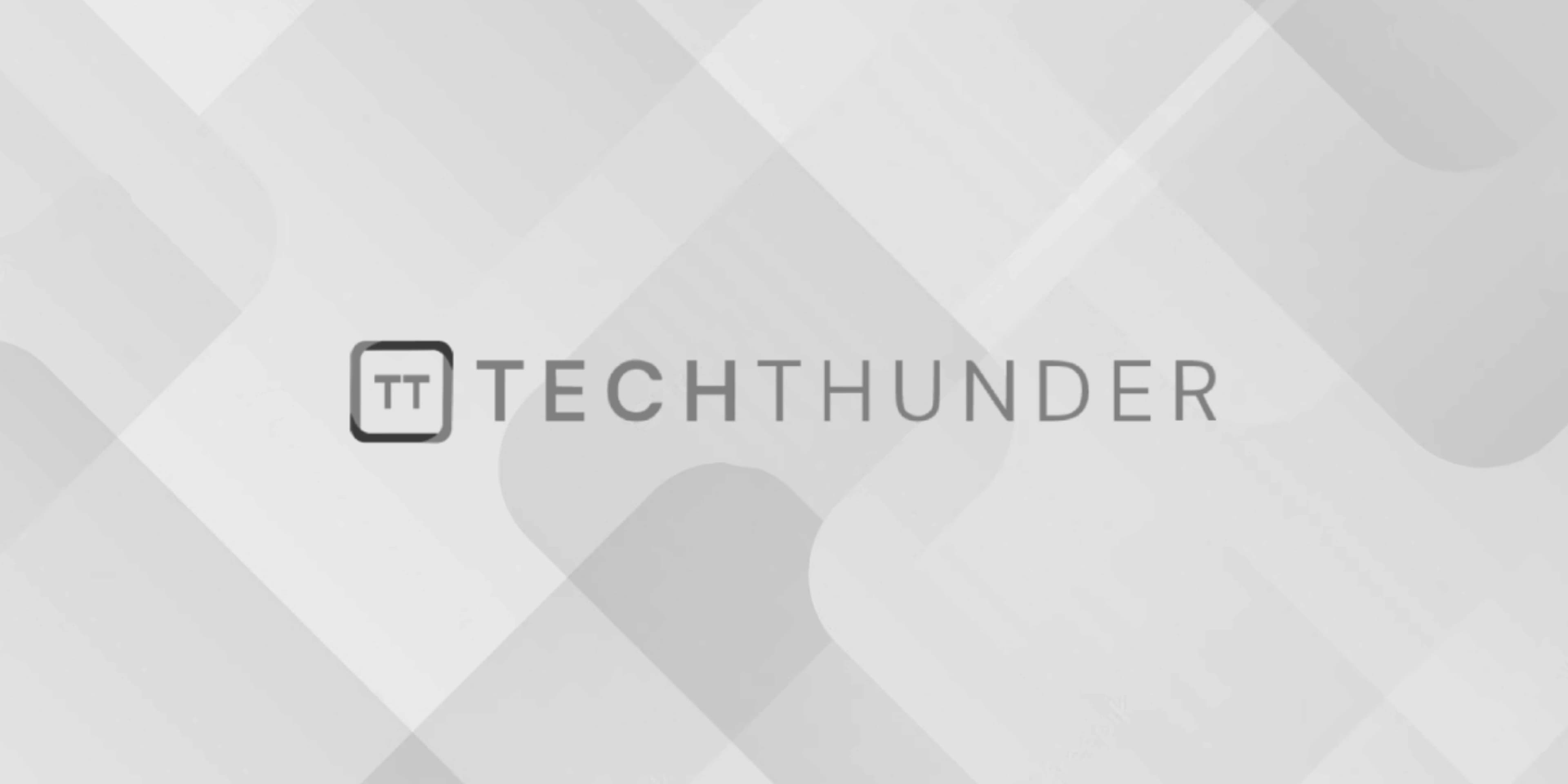
229 views
Strings Concatenation in C
To concatenate (combine) two strings in C, you can use the strcat
function or manually iterate through the characters in the strings and copy them to a new string. Here’s how to do it using both methods:
Using strcat
Function:
The strcat
function is a standard library function in C that concatenates (appends) one string to another. Here’s an example:
C
#include <stdio.h>
#include <string.h>
int main() {
char str1[100] = "Hello, ";
char str2[] = "world!";
strcat(str1, str2); // Concatenate str2 to the end of str1
printf("Concatenated string: %s\n", str1);
return 0;
}
In this program:
- We declare two character arrays,
str1
andstr2
, to hold the strings we want to concatenate. - We use the
strcat
function from the<string.h>
library to concatenatestr2
to the end ofstr1
. - Finally, we print the concatenated string.
Manual Concatenation (Iterating through Characters):
You can also concatenate strings manually by iterating through the characters in the source string and copying them to the destination string. Here’s an example:
C
#include <stdio.h>
#include <string.h>
int main() {
char str1[100] = "Hello, ";
char str2[] = "world!";
char concatenated[200]; // Create a new string to store the concatenated result
int i, j;
// Copy str1 to concatenated
for (i = 0; str1[i] != '\0'; i++) {
concatenated[i] = str1[i];
}
// Concatenate str2 to concatenated
for (j = 0; str2[j] != '\0'; j++) {
concatenated[i + j] = str2[j];
}
concatenated[i + j] = '\0'; // Null-terminate the concatenated string
printf("Concatenated string: %s\n", concatenated);
return 0;
}
In this manual concatenation example:
- We declare two character arrays,
str1
andstr2
, to hold the strings we want to concatenate, and a new character arrayconcatenated
to store the concatenated result. - We use
i
andj
as loop counters. - We copy
str1
toconcatenated
by iterating through its characters and copying them one by one. - We then concatenate
str2
toconcatenated
in a similar manner by iterating through its characters. - Finally, we add a null terminator
'\0'
at the end of theconcatenated
string to ensure it is properly terminated.
Both methods will result in the concatenation of the strings. Choose the one that suits your needs and coding style.