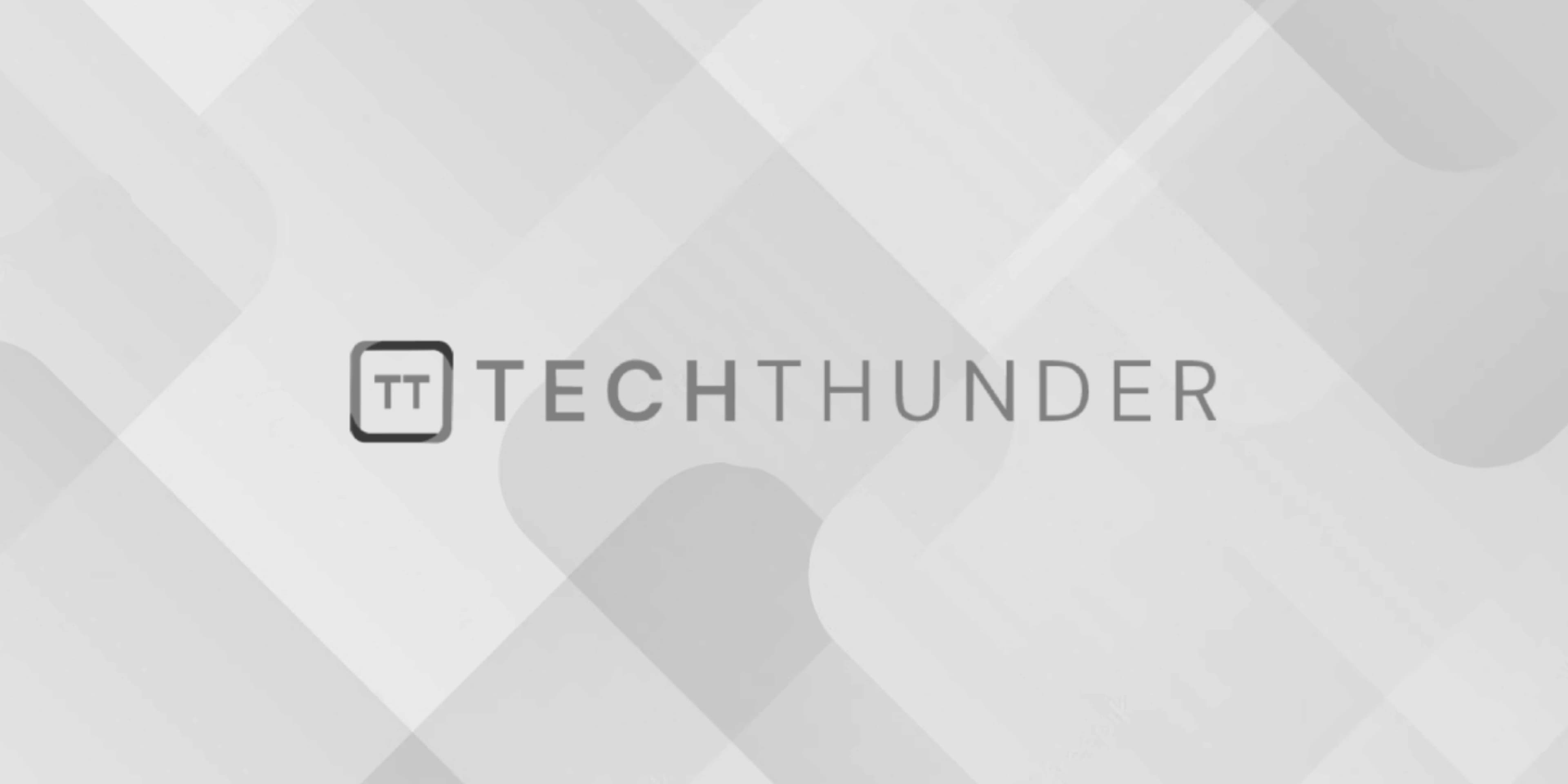
151 views
C Control Statement Test
The Control statements in C are used to control the flow of execution in a program. There are several types of control statements in C, including conditional statements (if
, else if
, else
), loops (for
, while
, do-while
), and branching statements (break
, continue
, return
, goto
). Here, I’ll provide examples of each type of control statement:
Conditional Statements (if
, else if
, else
):
C
#include <stdio.h>
int main() {
int num = 10;
if (num > 0) {
printf("The number is positive.\n");
} else if (num < 0) {
printf("The number is negative.\n");
} else {
printf("The number is zero.\n");
}
return 0;
}
Loops (for
, while
, do-while
):
for
Loop:
C
#include <stdio.h>
int main() {
for (int i = 1; i <= 5; i++) {
printf("Iteration %d\n", i);
}
return 0;
}
while
Loop:
C
#include <stdio.h>
int main() {
int count = 1;
while (count <= 5) {
printf("Count: %d\n", count);
count++;
}
return 0;
}
do-while
Loop:
C
#include <stdio.h>
int main() {
int count = 1;
do {
printf("Count: %d\n", count);
count++;
} while (count <= 5);
return 0;
}
Branching Statements (break
, continue
, return
, goto
):
break
Statement (used in loops to exit prematurely):
C
#include <stdio.h>
int main() {
for (int i = 1; i <= 10; i++) {
if (i == 5) {
break;
}
printf("Iteration %d\n", i);
}
return 0;
}
continue
Statement (used in loops to skip the current iteration and continue to the next one):
C
#include <stdio.h>
int main() {
for (int i = 1; i <= 5; i++) {
if (i == 3) {
continue;
}
printf("Iteration %d\n", i);
}
return 0;
}
return
Statement (used in functions to return a value and exit the function):
C
#include <stdio.h>
int multiply(int a, int b) {
return a * b;
}
int main() {
int result = multiply(3, 4);
printf("Result: %d\n", result);
return 0;
}
goto
Statement (rarely used and often discouraged):
C
#include <stdio.h>
int main() {
int num = 5;
if (num < 10) {
goto less_than_10;
}
printf("This will not be executed.\n");
less_than_10:
printf("Number is less than 10.\n");
return 0;
}
These examples demonstrate the basic usage of control statements in C. Depending on your specific programming needs, you can use these control statements to create more complex logic and control the flow of your program effectively.