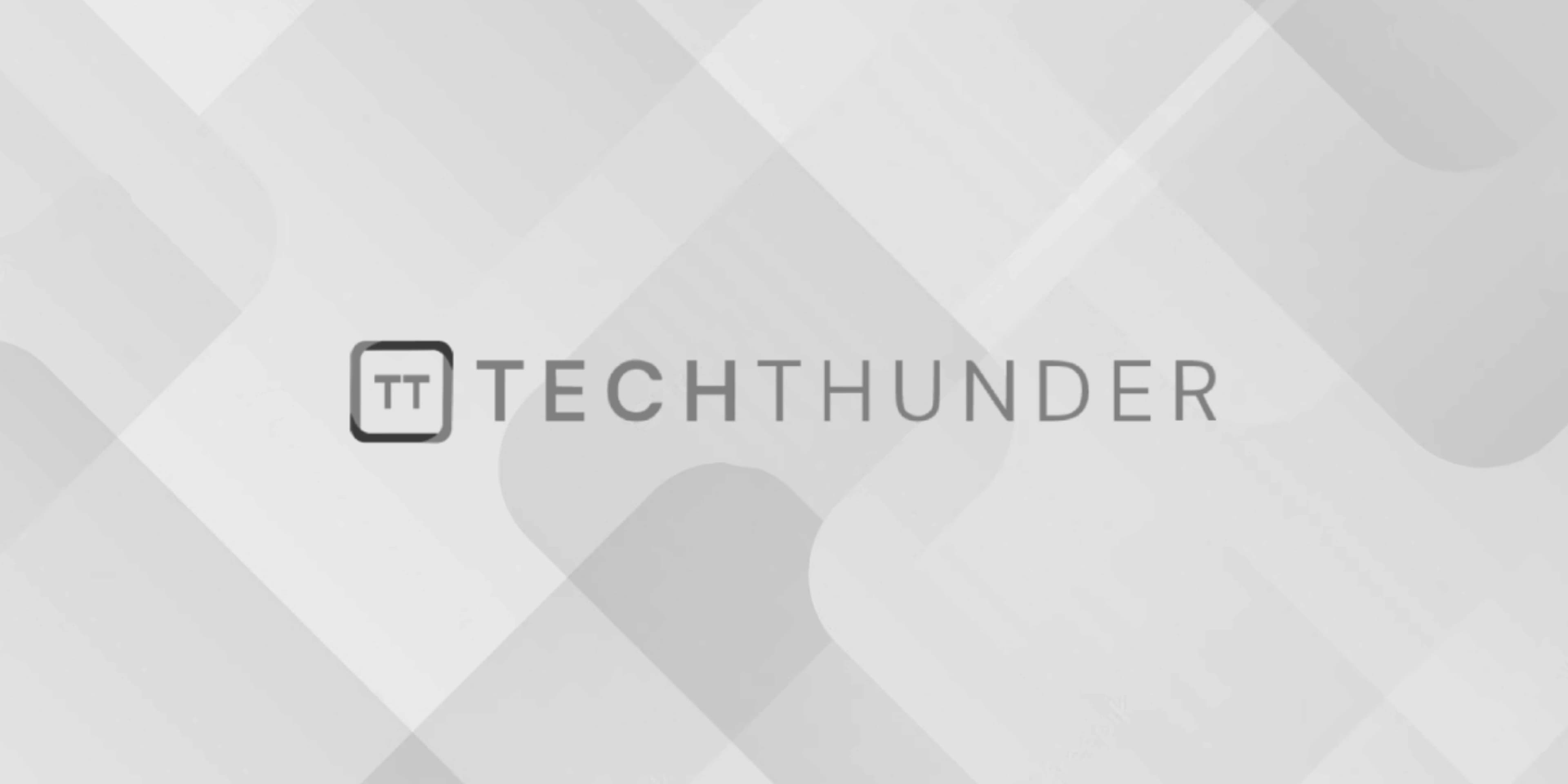
For vs While loop in C
The both for
and while
loops are used for iterative control flow, allowing you to repeat a block of code multiple times. However, they have slightly different syntax and use cases. Here’s an overview of both for
and while
loops in C:
for
Loop:
The for
loop is a control flow statement that allows you to execute a block of code repeatedly for a specified number of iterations. It is typically used when you know the exact number of times you want the loop to run.
Syntax of a for
loop:
for (initialization; condition; increment/decrement) {
// Code to be executed repeatedly
}
initialization
: This part is executed once at the beginning of the loop and is used to initialize a loop control variable.condition
: It is a Boolean expression that is evaluated before each iteration. If the condition istrue
, the loop continues; otherwise, it terminates.increment/decrement
: This part is executed after each iteration and is used to update the loop control variable.
Example of a for
loop:
#include <stdio.h>
int main() {
for (int i = 1; i <= 5; i++) {
printf("Iteration %d\n", i);
}
return 0;
}
This for
loop will run five times, and i
is incremented in each iteration.
while
Loop:
The while
loop is another control flow statement used for repetitive execution of a block of code. It is often used when you don’t know the exact number of iterations in advance, and the loop continues as long as a specified condition is true.
Syntax of a while
loop:
while (condition) {
// Code to be executed repeatedly
}
condition
: It is a Boolean expression that is evaluated before each iteration. If the condition istrue
, the loop continues; otherwise, it terminates.
Example of a while
loop:
#include <stdio.h>
int main() {
int count = 1;
while (count <= 5) {
printf("Iteration %d\n", count);
count++;
}
return 0;
}
This while
loop will run as long as count
is less than or equal to 5, and count
is incremented in each iteration.
Choosing Between for
and while
:
- Use a
for
loop when you know the number of iterations in advance or need to control the loop variable more explicitly. - Use a
while
loop when you want the loop to continue until a specific condition becomesfalse
, and you may not know the number of iterations in advance.
Both for
and while
loops are powerful and flexible constructs, and the choice between them depends on the specific requirements of your program.