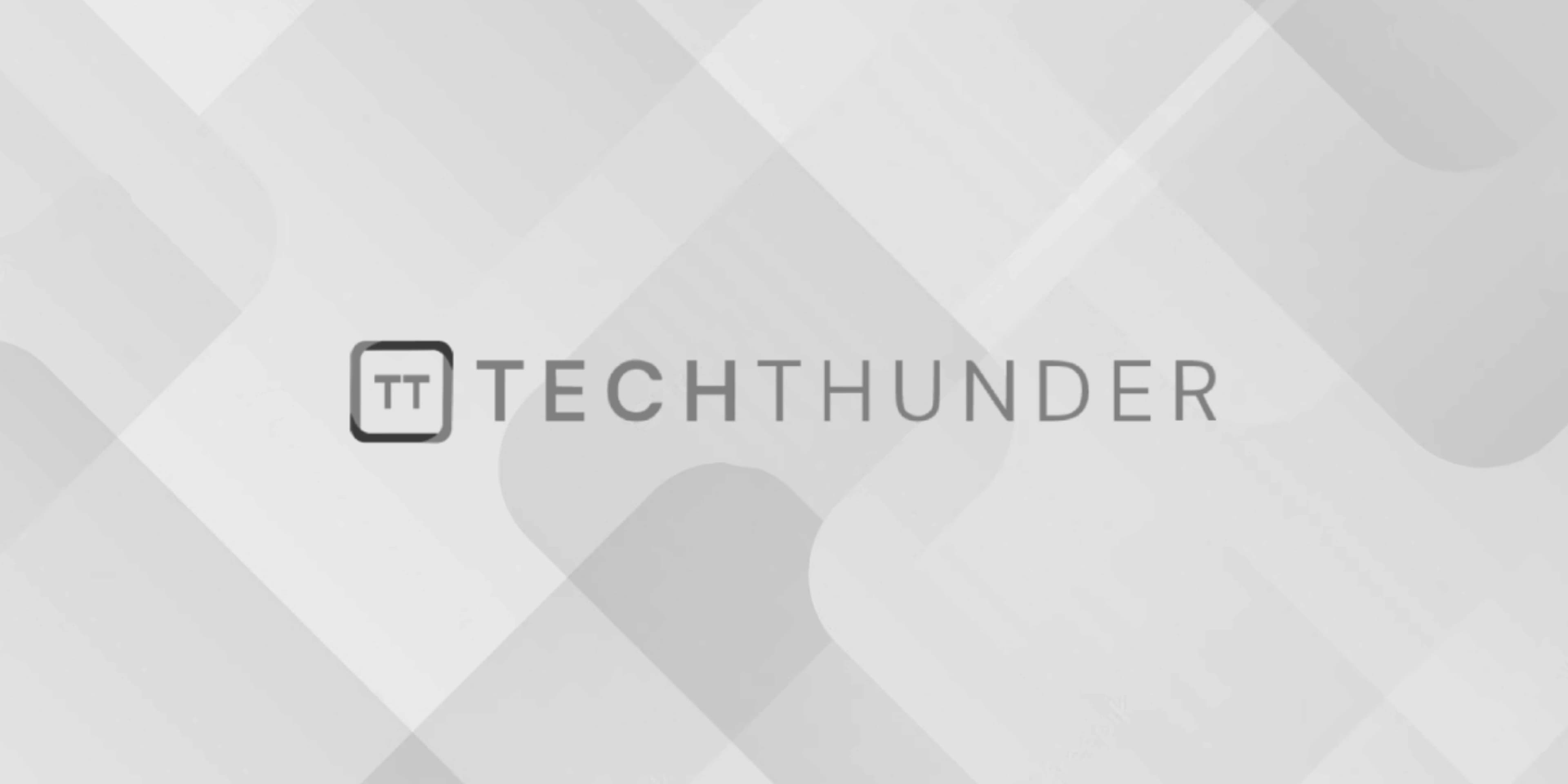
128 views
Sequence Points in C
The C and C++ has sequence points are specific points in the execution of a program where the side effects of previous expressions are guaranteed to be visible and complete. They help define the order of evaluation of expressions in an expression statement and ensure that the program behaves predictably. Sequence points are crucial for avoiding undefined behavior and ensuring that your code works as expected.
Here are some key aspects of sequence points in C:
- Order of Evaluation: C and C++ do not specify the order in which subexpressions within an expression are evaluated, except when a sequence point is encountered. Sequence points dictate when the effects of previous expressions must be visible before moving on to the next expression.
- Types of Sequence Points: There are specific situations that act as sequence points:
- At the end of a full expression (a statement or a function call). This is a major sequence point and ensures that all previous evaluations are completed before the next full expression begins.
- Before and after the evaluation of function arguments. For example, when calling a function, the arguments’ values must be determined before the function is called, and their side effects must be completed before moving on.
- The
&&
and||
operators create a sequence point. For example, in the expressiona() && b()
,a()
must be evaluated beforeb()
, and the result ofa()
will affect whetherb()
is evaluated. - The comma operator (
,
) creates a sequence point. In the expressiona, b
,a
is evaluated beforeb
. - Assignment operators (
=
,+=
,-=
, etc.) introduce sequence points. For example, inx = y = 5
, bothy
andx
must be assigned the value5
, and their values must be known before moving on.
- Undefined Behavior: Violating the sequence points can lead to undefined behavior. For example, if you modify a variable and read it in the same expression without a sequence point in between, you are in undefined territory.
Here’s an example to illustrate the importance of sequence points:
C
int x = 5;
int y = 0;
int z = (x++, y++);
In this code:
x++
incrementsx
and returns the original value (5), but it also introduces a sequence point. So,x
is incremented to 6, and the result ofx++
is 5.y++
incrementsy
and returns the original value (0), but it also introduces a sequence point. So,y
is incremented to 1, and the result ofy++
is 0.- The assignment
z = (x++, y++)
assigns 0 toz
. This is because bothx++
andy++
have sequence points, and their side effects are guaranteed to be complete before assigning their results toz
.
Understanding and respecting sequence points is crucial for writing reliable and predictable C and C++ code. Violating sequence points can lead to subtle and hard-to-debug issues in your programs.