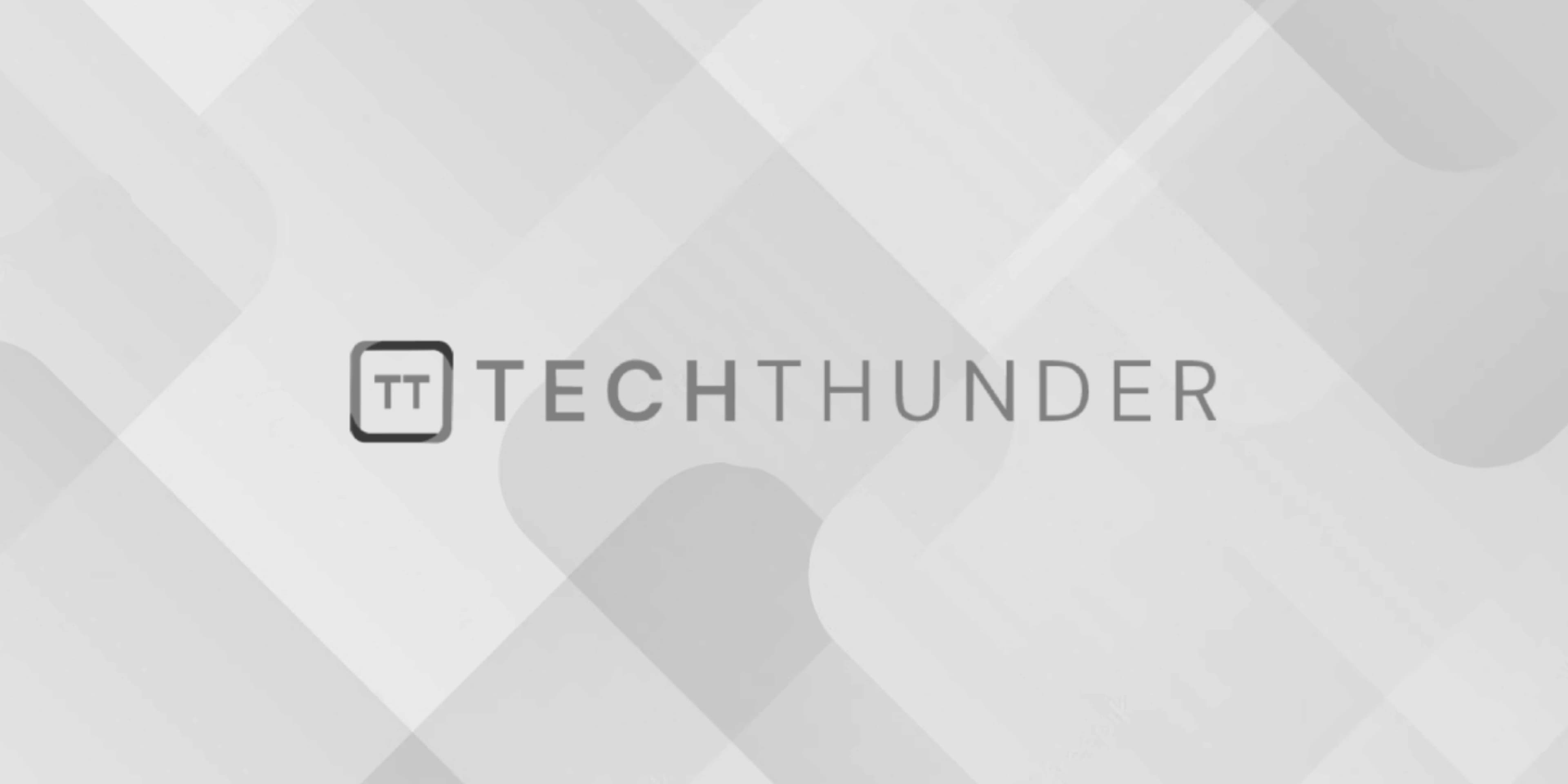
165 views
Segregate 0s and 1s into array in C
To segregate 0s and 1s into separate arrays in C, you can iterate through the input array and place each element into either the “0s array” or the “1s array” based on its value. Here’s a C program to achieve this segregation:
C
#include <stdio.h>
void segregateZerosAndOnes(int arr[], int n) {
int zeros[n]; // Array to store 0s
int ones[n]; // Array to store 1s
int zeroCount = 0;
int oneCount = 0;
// Iterate through the input array
for (int i = 0; i < n; i++) {
if (arr[i] == 0) {
zeros[zeroCount++] = arr[i];
} else {
ones[oneCount++] = arr[i];
}
}
// Copy the segregated arrays back to the original array
for (int i = 0; i < zeroCount; i++) {
arr[i] = zeros[i];
}
for (int i = 0; i < oneCount; i++) {
arr[zeroCount + i] = ones[i];
}
}
int main() {
int arr[] = {0, 1, 0, 1, 1, 0, 0, 1};
int n = sizeof(arr) / sizeof(arr[0]);
printf("Original Array: ");
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
segregateZerosAndOnes(arr, n);
printf("Segregated Array: ");
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
In this program:
- We create two separate arrays,
zeros
andones
, to store the 0s and 1s, respectively. These arrays have the same size as the input arrayarr
. - We use
zeroCount
andoneCount
to keep track of the number of 0s and 1s encountered during iteration. - We iterate through the input array
arr
, and for each element, if it is a0
, we store it in thezeros
array; otherwise, we store it in theones
array. - After segregating the elements, we copy the segregated arrays back to the original array
arr
. ThezeroCount
variable helps us determine the starting index for theones
array inarr
.
When you run this program, it will segregate the 0s and 1s in the original array into two separate sections within the same array.