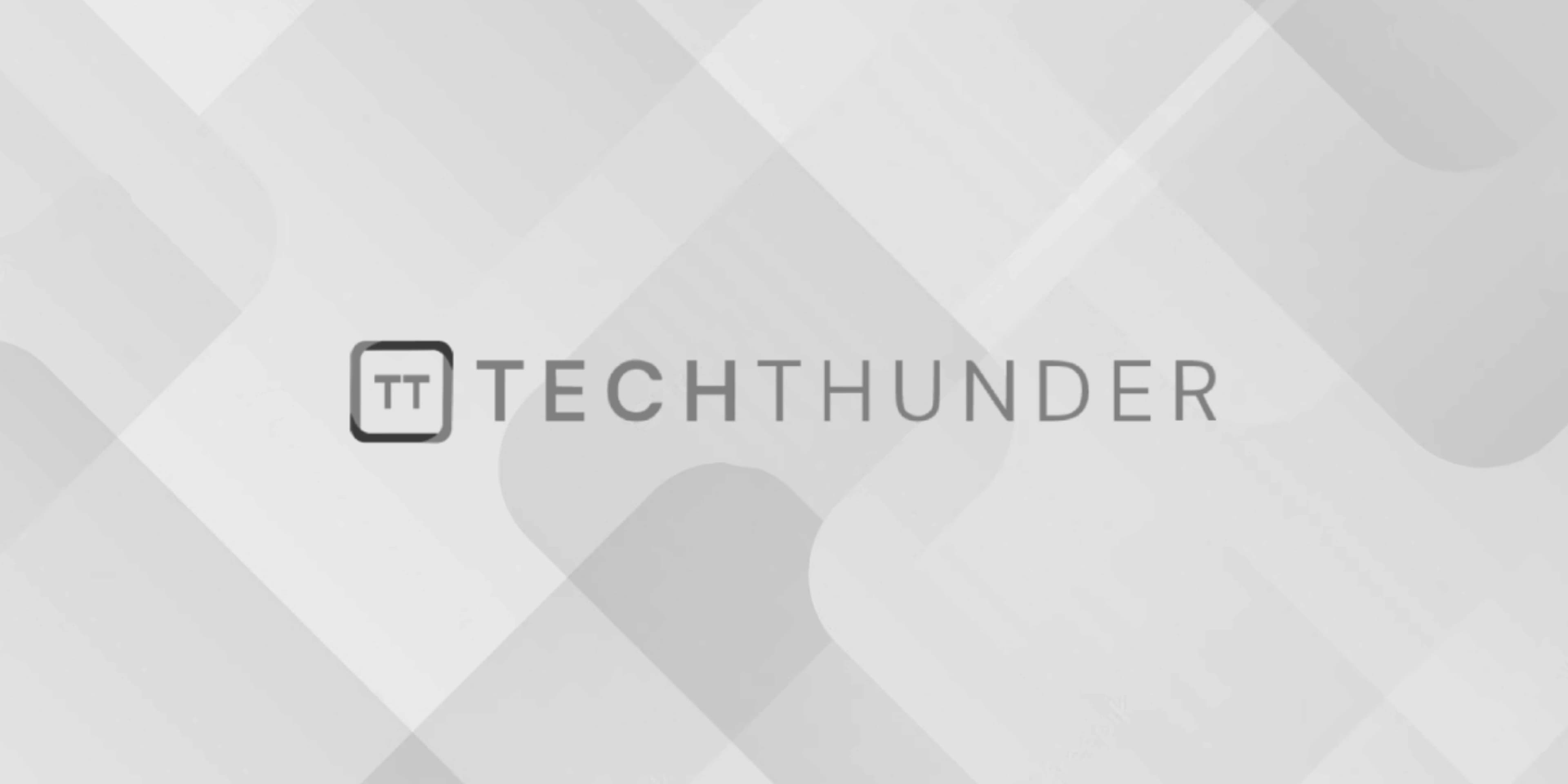
Random Function in C
You can generate random numbers using the rand()
function from the C Standard Library (stdlib.h). However, it’s important to note that the numbers generated by rand()
are not truly random but rather pseudo-random, meaning that they appear random but are generated by a deterministic algorithm. To use the rand()
function, you’ll typically follow these steps:
- Include the necessary header files:
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
- Seed the random number generator. You should do this only once in your program, usually at the beginning, to ensure that you get different sequences of random numbers on each run. You can use the
srand()
function with a seed value. A common choice for the seed is the current time:
srand(time(NULL));
- Generate random numbers using the
rand()
function. You can use the modulo operator to limit the range of random numbers to a specific interval. For example, to generate a random integer between 0 and 99:
int random_number = rand() % 100; // Generates a random number between 0 and 99
Here’s a complete example:
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main() {
// Seed the random number generator with the current time
srand(time(NULL));
// Generate and print 10 random numbers between 0 and 99
for (int i = 0; i < 10; i++) {
int random_number = rand() % 100; // Generates a random number between 0 and 99
printf("%d\n", random_number);
}
return 0;
}
Keep in mind that the rand()
function may not produce truly random results, and its quality can vary between different C libraries and implementations. If you need higher-quality random numbers, consider using external libraries like the C++ <random>
library or platform-specific functions for random number generation, such as arc4random()
on macOS and randombytes()
in libsodium for cryptographic applications.