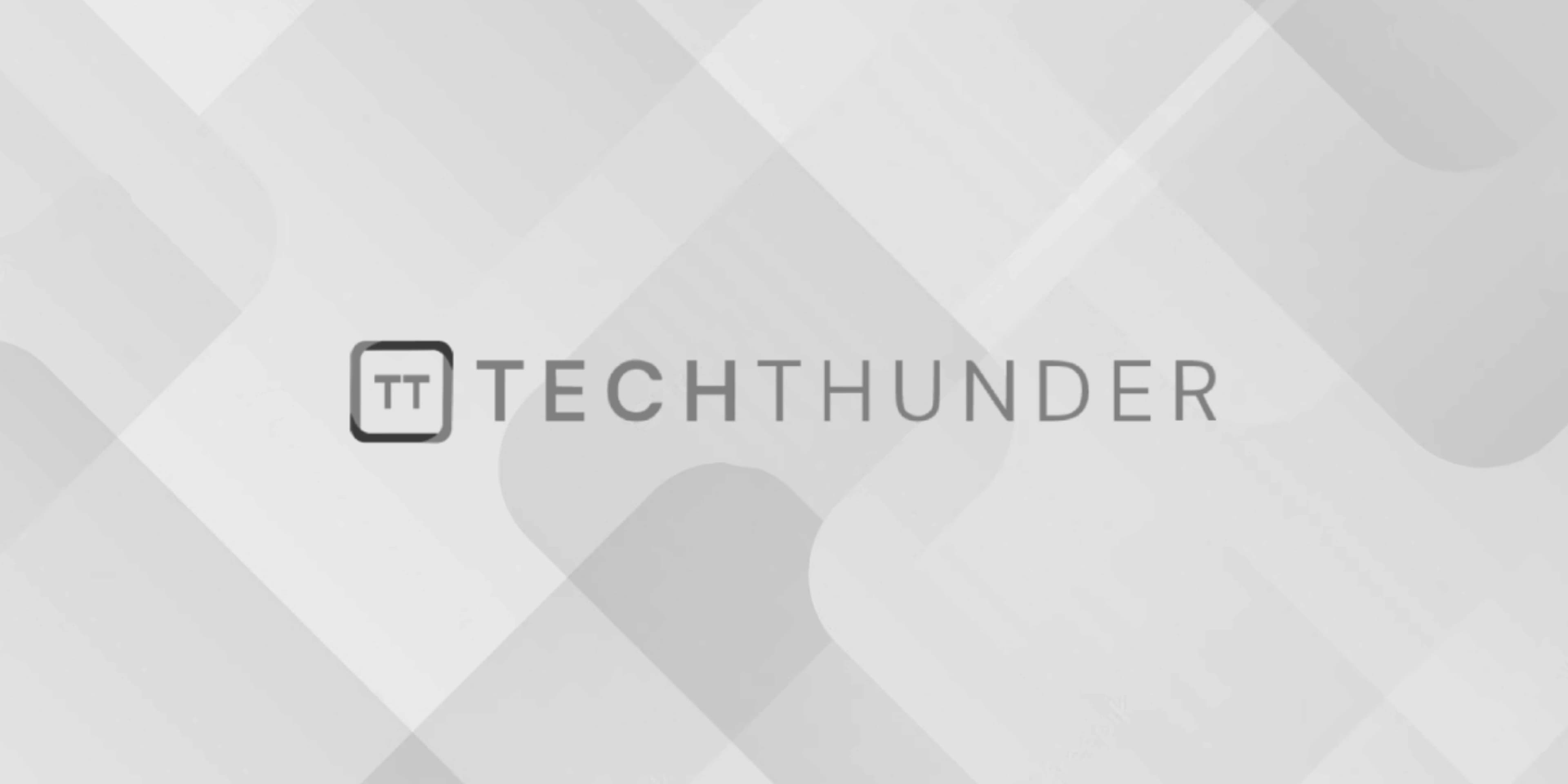
C break
The break
statement is a control statement used to terminate the execution of a loop or switch statement prematurely, without waiting for the normal loop or switch conditions to be met. The break
statement is commonly used to exit a loop when a certain condition is satisfied, or to exit a switch
statement once a specific case has been executed.
Here’s how the break
statement is used in different contexts:
- Inside Loops (for, while, do-while):
- When
break
is encountered inside a loop, it immediately exits the loop, and control resumes with the statement immediately following the loop. - It is often used with conditional statements to break out of a loop when a specific condition is met.
for (int i = 1; i <= 10; i++) {
if (i == 5) {
break; // Exit the loop when i equals 5
}
printf("%d ", i);
}
In this example, the loop is terminated when i
becomes equal to 5, and the program proceeds to the next statement after the loop.
- Inside a
switch
Statement:
- In a
switch
statement, thebreak
statement is used to exit theswitch
block after a specificcase
is executed. - Without
break
, theswitch
statement would continue executing subsequentcase
labels until abreak
statement is encountered.
int choice = 2;
switch (choice) {
case 1:
printf("Option 1\n");
break;
case 2:
printf("Option 2\n");
break; // Exit the switch after executing case 2
case 3:
printf("Option 3\n");
break;
default:
printf("Invalid option\n");
}
In this example, when choice
is 2, the program executes the code for case 2
and then exits the switch
statement using break
.
- Labeled
break
:
- In some situations, you can use a labeled
break
statement to exit nested loops. This requires defining a label before the loop you want to break out of.
for (int i = 1; i <= 3; i++) {
for (int j = 1; j <= 3; j++) {
if (i * j == 6) {
goto exit_loop;
}
}
}
exit_loop:
printf("Exited the nested loop.\n");
Here, the goto
statement with the label exit_loop
is used to break out of both nested loops simultaneously.
The break
statement is a useful tool for controlling the flow of your code, especially in loops and switch
statements. It allows you to exit these constructs when specific conditions are met, making your code more flexible and efficient.