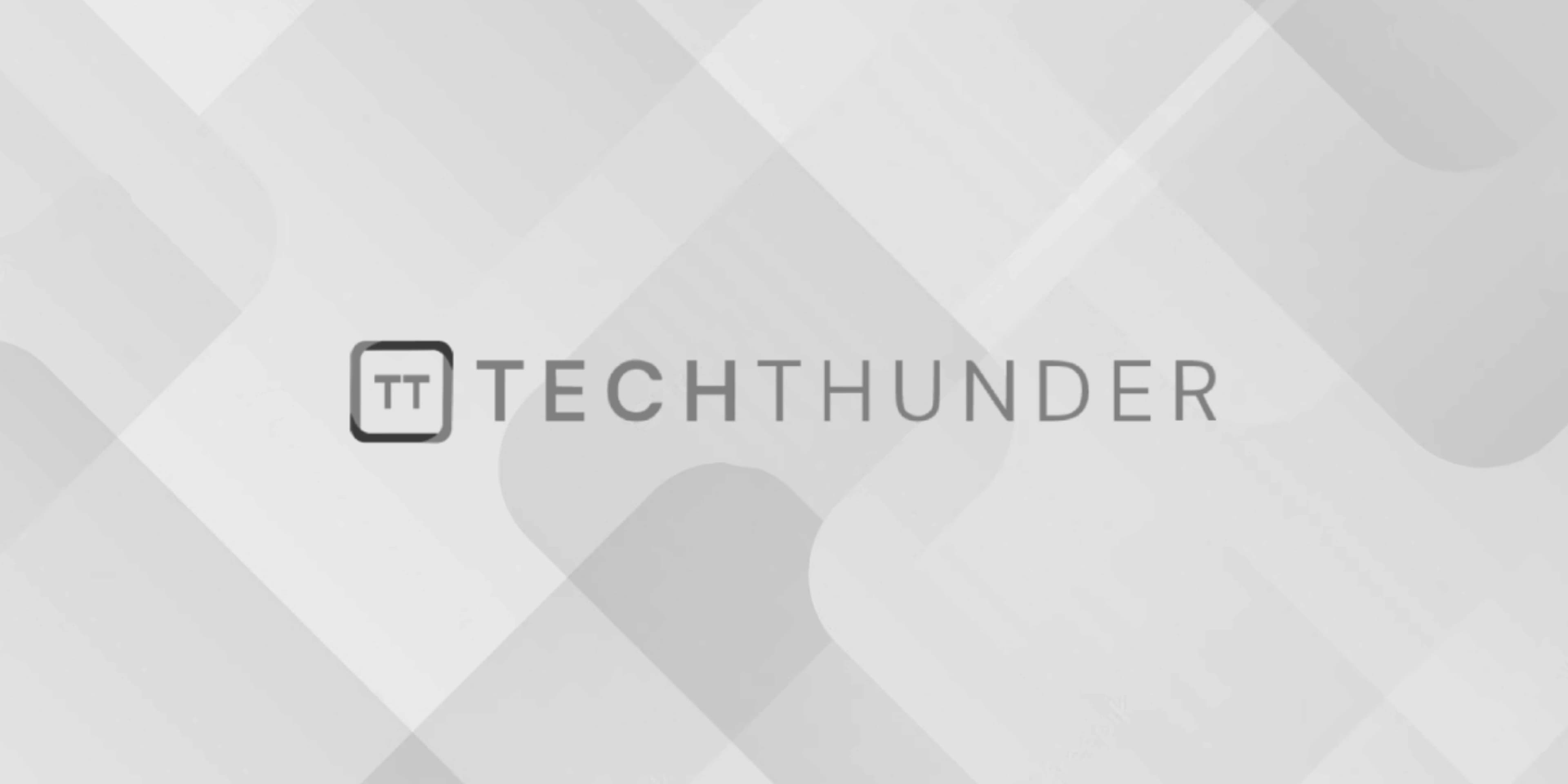
189 views
Sum of digits in C
To calculate the sum of digits in a given integer in C, you can repeatedly extract each digit by taking the remainder when dividing the number by 10 and then add those digits together. Here’s a C program to calculate the sum of digits:
C
#include <stdio.h>
int main() {
int num, sum = 0;
printf("Enter an integer: ");
scanf("%d", &num);
// Calculate the sum of digits
while (num != 0) {
int digit = num % 10; // Extract the last digit
sum += digit; // Add the digit to the sum
num /= 10; // Remove the last digit
}
printf("Sum of digits: %d\n", sum);
return 0;
}
In this program:
- We input an integer from the user.
- We initialize
sum
to 0, which will store the sum of digits. - Inside a
while
loop, we repeatedly extract the last digit ofnum
using the modulo operator (%
). This digit is stored in thedigit
variable. - We add the
digit
tosum
, accumulating the sum of digits. - We remove the last digit from
num
by dividing it by 10. - We repeat this process until
num
becomes zero. - Finally, we print the
sum
, which contains the sum of the digits of the input number.
Compile and run the program, and it will calculate and display the sum of the digits in the entered integer.