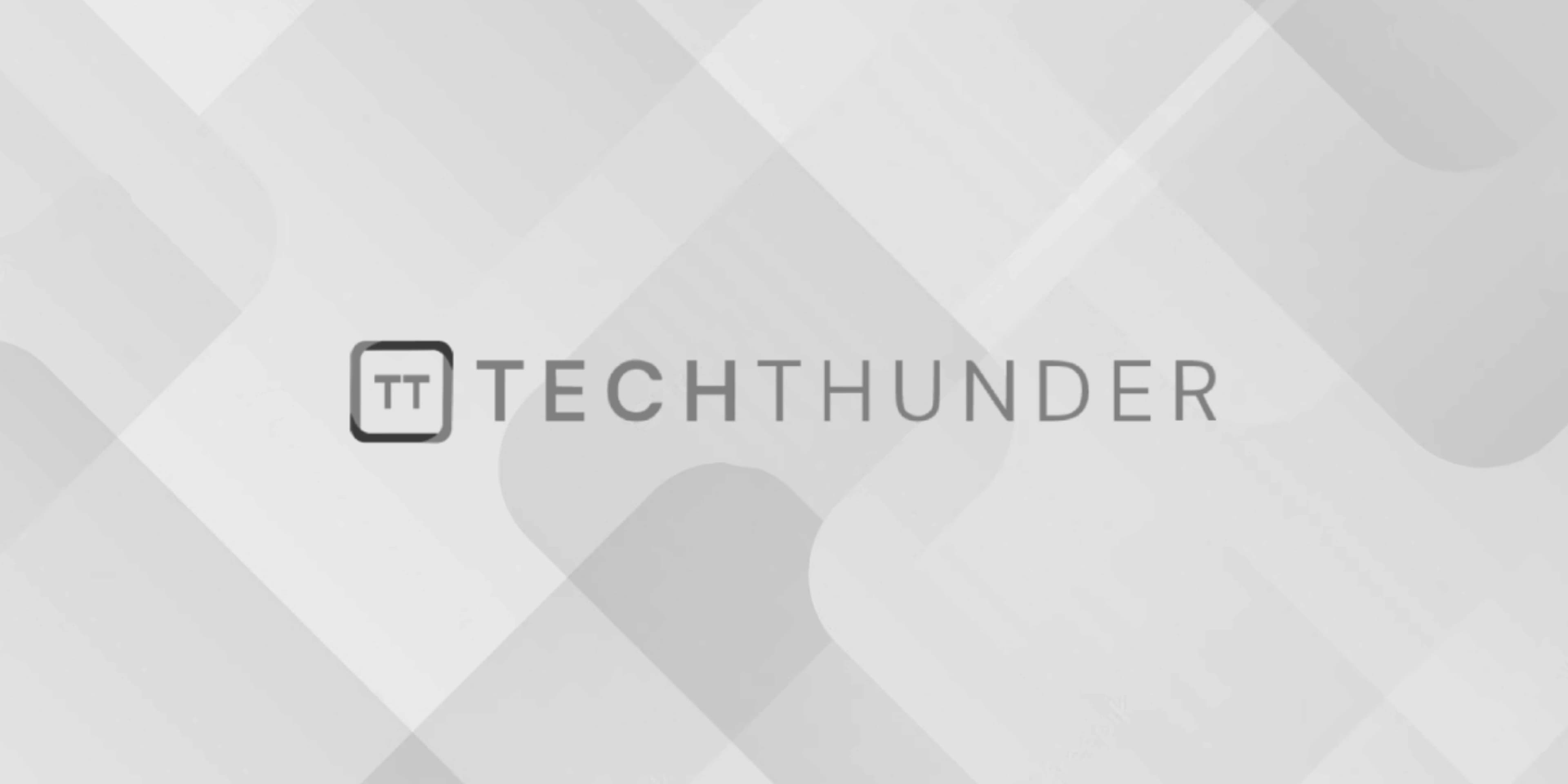
227 views
2D Vector in C++ with User Defined Size
The C++ can create a 2D vector with a user-defined size using the std::vector
container. Here’s how to do it:
C++
#include <iostream>
#include <vector>
int main() {
int numRows, numCols;
// Get user input for the number of rows and columns
std::cout << "Enter the number of rows: ";
std::cin >> numRows;
std::cout << "Enter the number of columns: ";
std::cin >> numCols;
// Create a 2D vector with the specified size
std::vector<std::vector<int>> matrix(numRows, std::vector<int>(numCols));
// Initialize the elements (optional)
for (int i = 0; i < numRows; ++i) {
for (int j = 0; j < numCols; ++j) {
matrix[i][j] = i * numCols + j; // Example initialization
}
}
// Print the 2D vector
std::cout << "The 2D vector:" << std::endl;
for (int i = 0; i < numRows; ++i) {
for (int j = 0; j < numCols; ++j) {
std::cout << matrix[i][j] << " ";
}
std::cout << std::endl;
}
return 0;
}
In this example:
- We first get user input for the number of rows (
numRows
) and the number of columns (numCols
) that the 2D vector should have. - We then create a 2D vector named
matrix
with the specified size using the constructor that takes two arguments: the number of rows and a vector of values to initialize each row. In this case, we initialize each row with a vector of integers of sizenumCols
. - Optionally, we can initialize the elements of the 2D vector using nested loops. In this example, we initialize the elements with consecutive integers, but you can initialize them as needed based on your requirements.
- Finally, we print the contents of the 2D vector to verify its initialization.
This code snippet demonstrates how to create and initialize a 2D vector with a user-defined size. You can modify the initialization logic and data types to suit your specific needs.