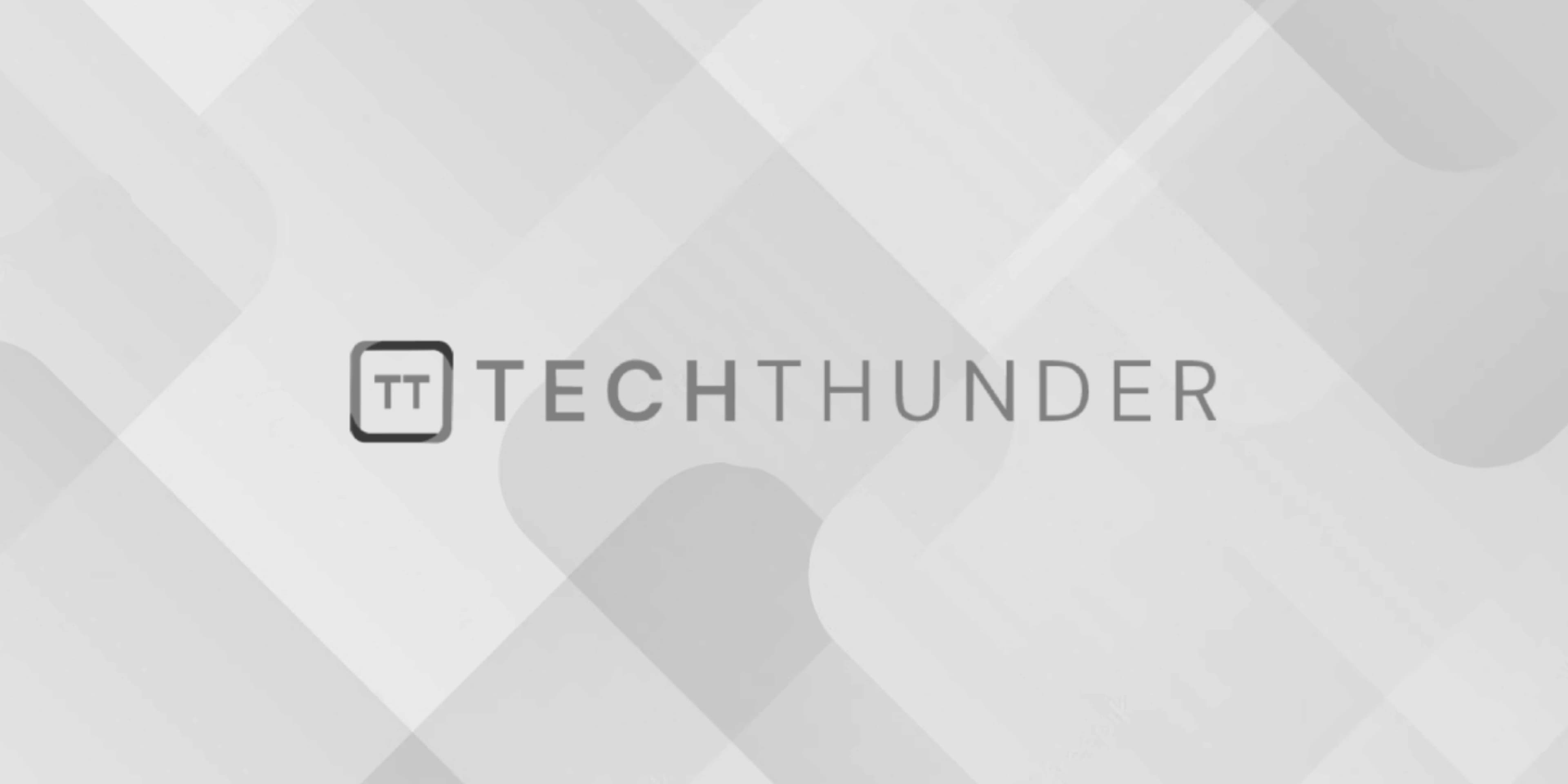
252 views
Pair in C++
In C++, std::pair
is a template class that allows you to store two values of different types as a single unit. It’s part of the C++ Standard Library and is commonly used in various situations where you need to represent a pair of values. Each value within the pair can have its own data type.
Here’s how to use std::pair
:
#include <iostream>
#include <utility> // Include the utility header for std::pair
int main() {
std::pair<int, double> myPair; // Declare a pair of int and double
myPair.first = 42; // Assign a value to the first element of the pair
myPair.second = 3.14; // Assign a value to the second element of the pair
// Access the elements of the pair
std::cout << "First element: " << myPair.first << std::endl;
std::cout << "Second element: " << myPair.second << std::endl;
// Create and initialize a pair
std::pair<std::string, bool> status("OK", true);
// Access elements using structured binding (C++17 and later)
auto [message, success] = status;
std::cout << "Message: " << message << ", Success: " << success << std::endl;
return 0;
}
In this example:
- We include the
<utility>
header to usestd::pair
. - We declare a
std::pair
namedmyPair
with the first element of typeint
and the second element of typedouble
. - We assign values to the first and second elements of
myPair
. - We access and print the elements of the pair using
myPair.first
andmyPair.second
. - We create and initialize another pair named
status
with a string and a boolean value. - We use structured binding (available in C++17 and later) to access and print the elements of the
status
pair.
std::pair
is often used in situations where you need to return multiple values from a function, represent key-value pairs in associative containers (e.g., std::map
), or store pairs of related data in various data structures. It provides a convenient way to bundle two values together.