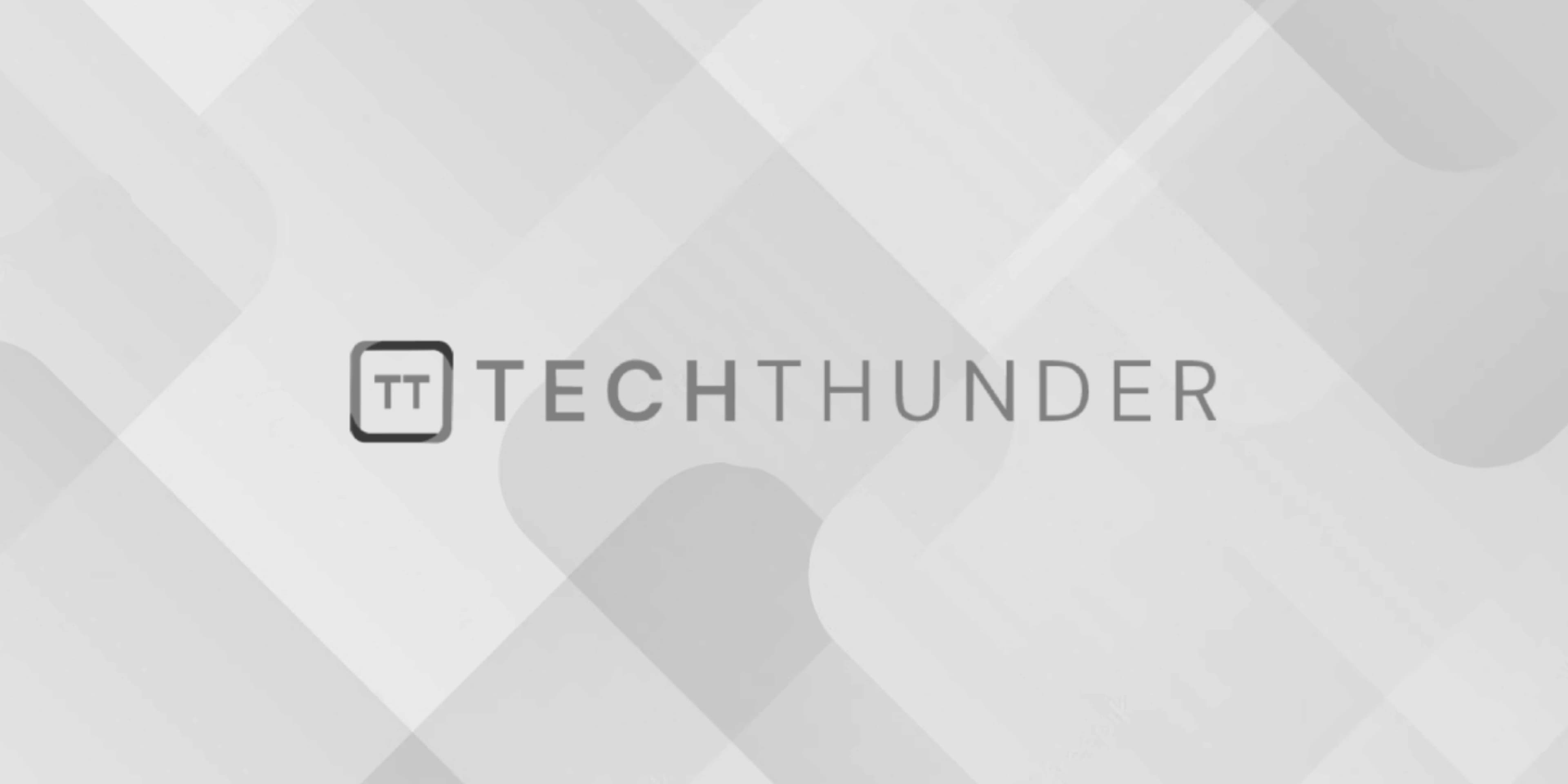
What is an abstract class in C++
The abstract class in C++ is a class that cannot be instantiated on its own but serves as a blueprint for other classes. It is designed to be subclassed (i.e., derived from) by other classes, and it often contains one or more pure virtual functions. Abstract classes are used to define a common interface or contract that derived classes must adhere to. Here are some key characteristics and concepts related to abstract classes in C++:
- Pure Virtual Functions: An abstract class typically contains one or more pure virtual functions. A pure virtual function is a function declared in the abstract class with the
virtual
keyword and no implementation. It is denoted by= 0
at the end of its declaration. Derived classes are required to provide implementations for all pure virtual functions. Example:
class AbstractShape {
public:
virtual void draw() const = 0; // Pure virtual function
};
- No Object Creation: You cannot create objects of an abstract class directly. Attempting to do so will result in a compilation error. Abstract classes exist solely for the purpose of inheritance.
- Inheritance: Derived classes inherit the interface (pure virtual functions) and can provide concrete implementations for those functions. They must override all pure virtual functions to become concrete classes. Example:
class Circle : public AbstractShape {
public:
void draw() const override {
// Implement the draw function for Circle
}
};
- Polymorphism: Abstract classes and polymorphism go hand in hand. You can use pointers or references to the abstract base class to hold objects of derived classes. This allows you to achieve runtime polymorphism and call the appropriate functions based on the actual object type. Example:
AbstractShape* shape = new Circle;
shape->draw(); // Calls the draw function for Circle
delete shape;
- Abstract Base Classes: Abstract classes are often referred to as abstract base classes because they serve as a base for other classes. Concrete classes that derive from an abstract base class are sometimes called derived classes or concrete subclasses.
Abstract classes are useful when you want to define a common interface for a group of related classes while enforcing that certain functions must be implemented by derived classes. They are commonly used in design patterns like the Factory Method and Strategy patterns, where you want to define a family of classes with a shared interface but varying implementations.