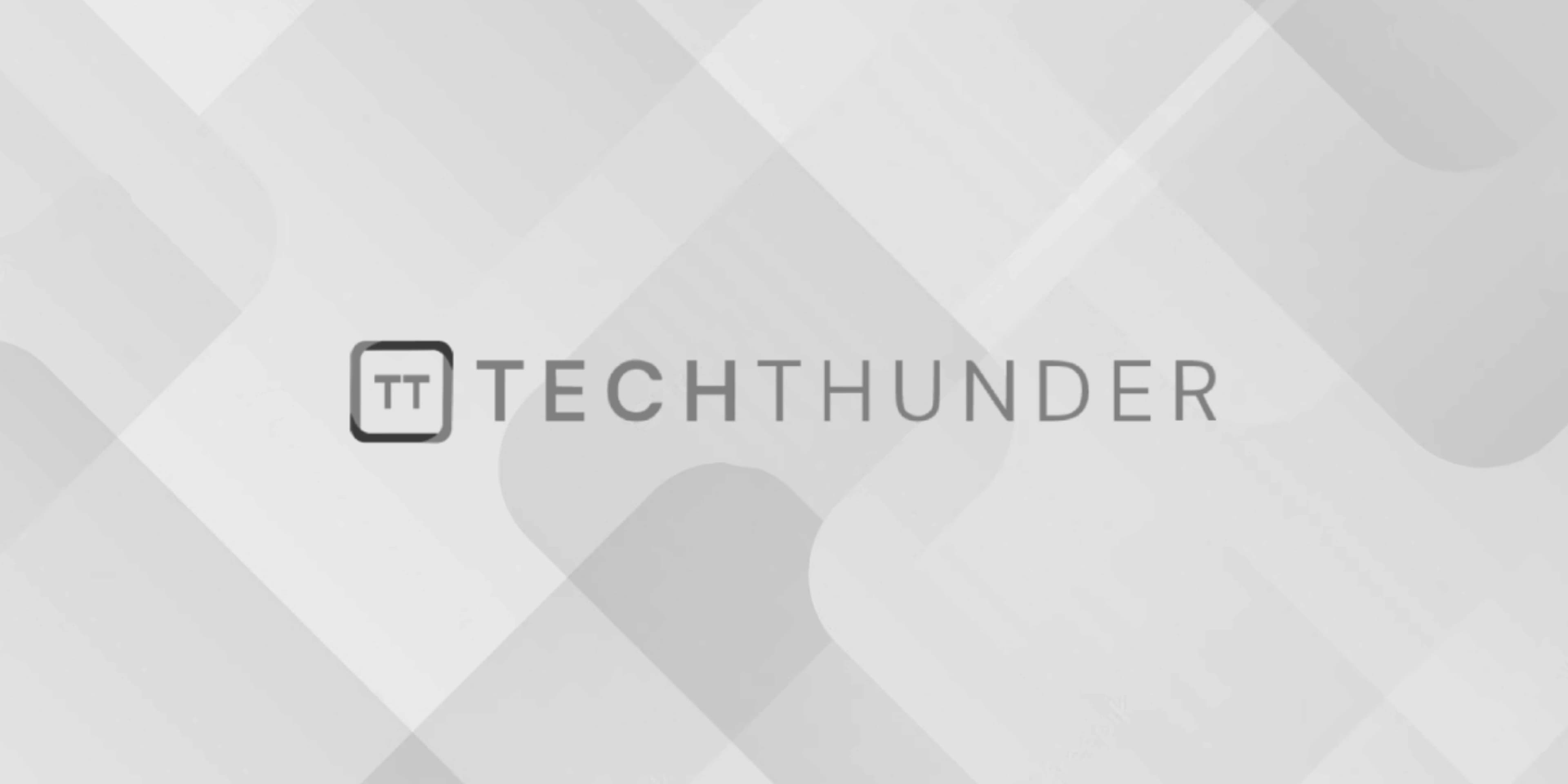
C++ Functions
The C++ functions are blocks of reusable code that perform a specific task. They help organize code into logical modules and make it easier to maintain and debug. Functions in C++ can have parameters (input values) and can return a value.
Here’s how you define and use functions in C++:
Function Declaration and Definition:
// Function declaration (also known as function prototype)
return_type function_name(parameter_type1 param1, parameter_type2 param2, ...);
// Function definition
return_type function_name(parameter_type1 param1, parameter_type2 param2, ...) {
// Function body
// Code to perform the task
return value; // Return statement (if the function has a return type)
}
Example:
#include <iostream>
// Function declaration (prototype)
int add(int a, int b);
int main() {
int result = add(3, 4); // Function call
std::cout << "Result: " << result << std::endl;
return 0;
}
// Function definition
int add(int a, int b) {
return a + b;
}
Output:
Result: 7
Function Components:
- Return Type: Specifies the type of data the function will return. If the function doesn’t return anything, use
void
. - Function Name: A unique identifier for the function.
- Parameters: Input values that the function accepts. These are defined in the parentheses. If a function doesn’t take any parameters, leave the parentheses empty or use
void
to indicate no parameters. - Function Body: The block of code that performs the task of the function. This is enclosed in curly braces
{}
. - Return Statement: If the function has a return type, it must use a
return
statement to specify the value to be returned. This statement is optional in functions with avoid
return type.
Function Overloading:
C++ allows you to define multiple functions with the same name but different parameter lists. This is known as function overloading.
int add(int a, int b);
double add(double a, double b);
Default Arguments:
You can provide default values for function parameters. These values will be used if the caller does not provide a value for that parameter.
int multiply(int a, int b = 2); // b has a default value of 2
Recursive Functions:
A recursive function is a function that calls itself. This technique is often used to solve problems that can be broken down into smaller, similar subproblems.
int factorial(int n) {
if (n <= 1)
return 1;
return n * factorial(n - 1);
}
Functions are a fundamental building block in C++, and they play a crucial role in writing modular and maintainable code. They allow you to break down complex tasks into smaller, manageable pieces.