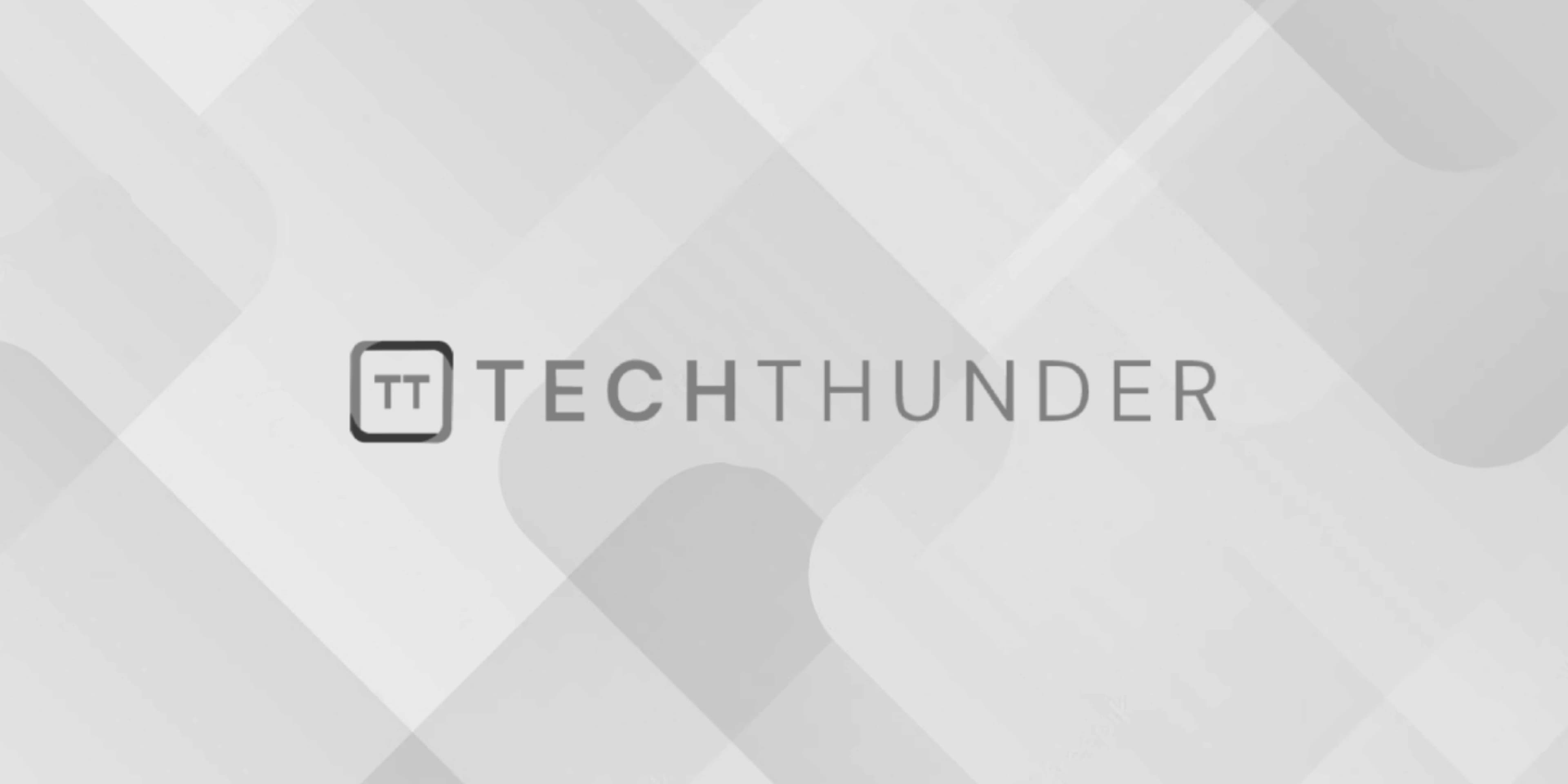
Friend Function in C++
The C++ friend function is a function that is not a member of a class but has access to the private and protected members of that class. It is declared as a friend of the class using the friend
keyword in the class declaration. Friend functions are often used to allow external functions or classes to access and manipulate the private or protected data of a class when it is necessary, while still maintaining encapsulation.
Here’s the basic syntax for declaring and defining a friend function in C++:
class MyClass {
private:
int privateData;
public:
MyClass(int value) : privateData(value) {}
// Declaration of a friend function
friend void friendFunction(MyClass obj);
};
// Definition of the friend function
void friendFunction(MyClass obj) {
cout << "Friend function accessing privateData: " << obj.privateData << endl;
}
int main() {
MyClass myObj(42);
friendFunction(myObj); // Calling the friend function
return 0;
}
In this example, friendFunction
is declared as a friend of the MyClass
class. As a result, it can access the private member privateData
of the MyClass
objects, even though it is not a member function of the class.
Some important points to remember about friend functions:
- Friend functions are not part of the class and are defined outside the class definition.
- They can be declared in the class, but their actual implementation is done outside of the class.
- Friend functions can access both private and protected members of the class that declares them as friends.
- They are often used in situations where external functions or classes need access to private members for specific operations, without violating the principles of encapsulation.
- Be cautious when using friend functions, as they can potentially break encapsulation if not used carefully. They should be used sparingly and only when necessary.