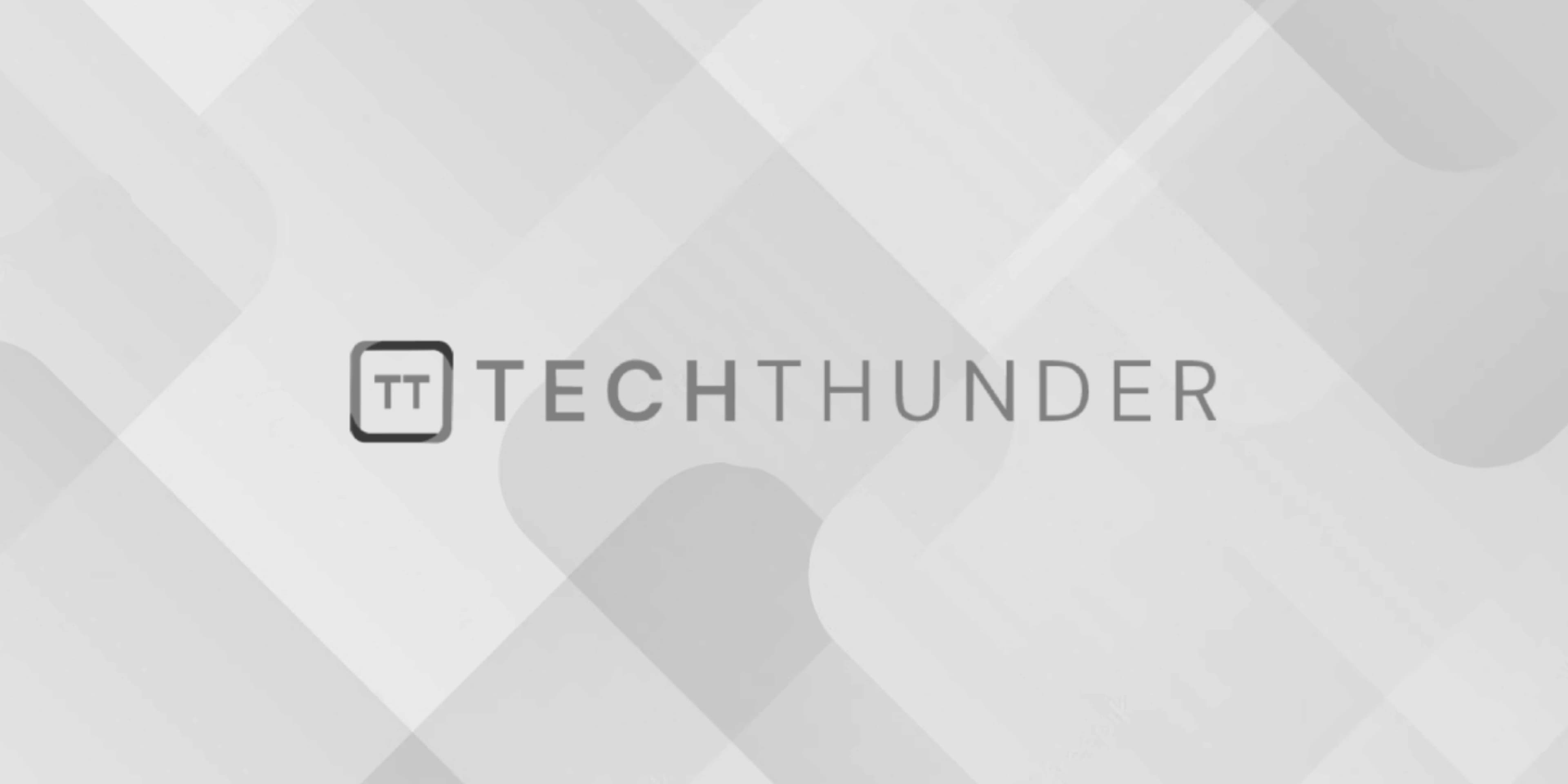
172 views
Jump Statements in C++
Jump statements in C++ are used to control the flow of program execution by transferring control from one part of the code to another. C++ provides three jump statements:
break
Statement:
- The
break
statement is used to exit the current loop or switch statement prematurely. - It is commonly used in
for
,while
, anddo-while
loops to terminate the loop before its normal termination condition is met. - It is also used in
switch
statements to exit theswitch
block after a case is matched. - Example:
C++
for (int i = 1; i <= 10; i++) {
if (i == 5) {
break; // Exit the loop when i equals 5
}
cout << i << " ";
}
continue
Statement:
- The
continue
statement is used to skip the rest of the current iteration of a loop and proceed to the next iteration. - It is often used when you want to skip some specific processing for certain loop iterations.
- Example:
C++
for (int i = 1; i <= 10; i++) {
if (i % 2 == 0) {
continue; // Skip even numbers
}
cout << i << " ";
}
return
Statement:
- The
return
statement is used to exit a function and return a value (if the function has a return type other thanvoid
) to the caller. - It can also be used to exit a function prematurely before its normal end if necessary.
- Example:
C++
int add(int a, int b) {
return a + b; // Exit the function and return the result
}
int main() {
int sum = add(5, 3); // Call the function and assign the result to 'sum'
return 0; // Exit the 'main' function and the program
}
Jump statements should be used judiciously in your code as excessive use of break
and continue
can make the code less readable and harder to maintain. Similarly, use the return
statement to exit functions when necessary but avoid using it for premature exits unless it enhances code clarity and readability.