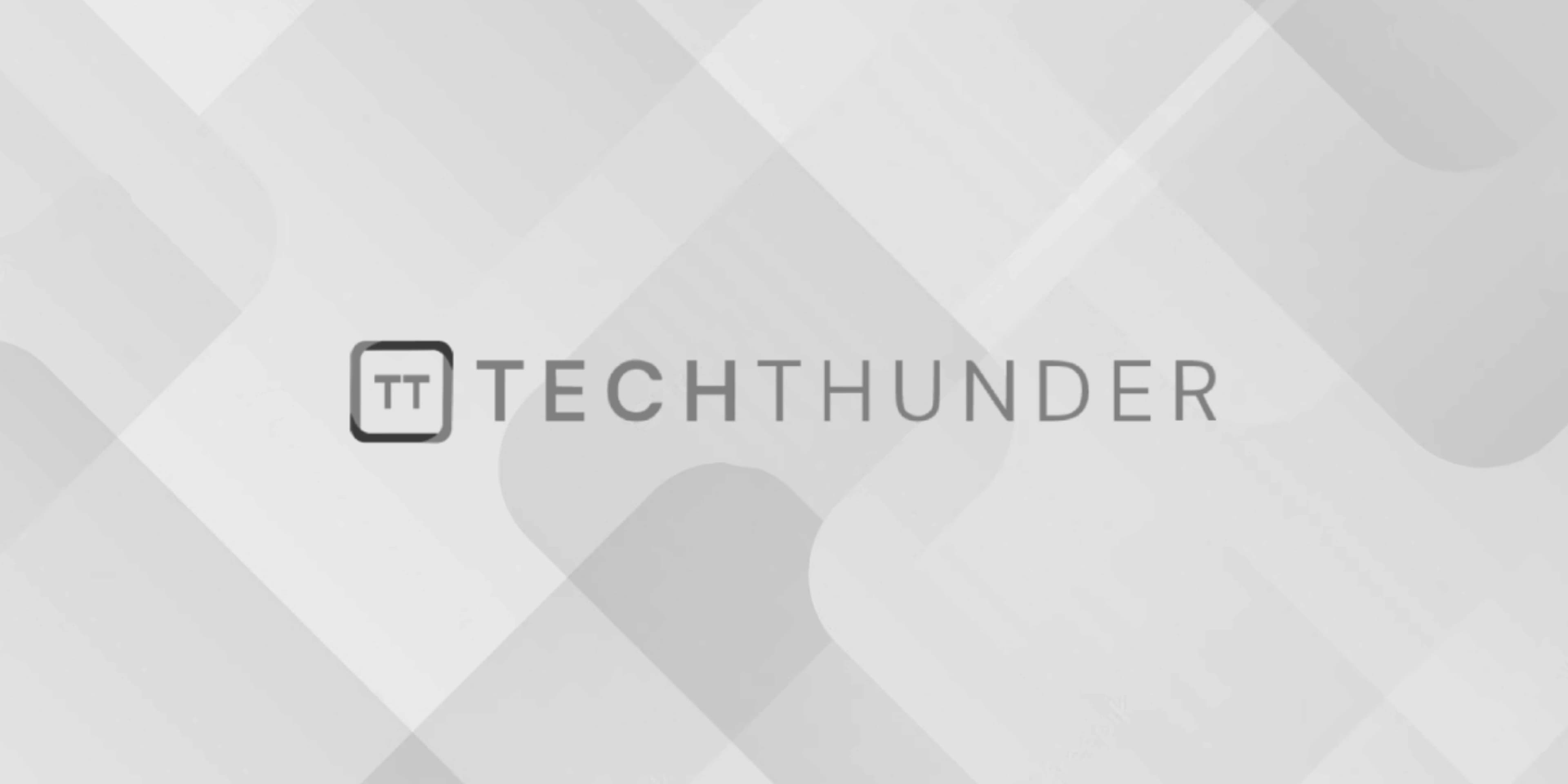
C++ Continue Statement
The C++ continue
statement is used to skip the rest of the current iteration of a loop and jump to the next iteration. It is typically used in loops (such as for
, while
, and do-while
) to control the flow of the loop based on a specific condition.
Here’s how the continue
statement works:
#include <iostream>
int main() {
for (int i = 1; i <= 5; ++i) {
if (i == 3) {
continue; // Skip the current iteration when i equals 3
}
std::cout << i << " ";
}
std::cout << std::endl;
return 0;
}
Output:
1 2 4 5
In this example, when i
is equal to 3, the continue
statement is executed, causing the loop to skip the remainder of the current iteration and proceed to the next iteration. As a result, the number 3 is not printed.
You can also use the continue
statement in while
and do-while
loops:
#include <iostream>
int main() {
int i = 1;
while (i <= 5) {
if (i == 3) {
++i;
continue; // Skip the current iteration when i equals 3
}
std::cout << i << " ";
++i;
}
std::cout << std::endl;
return 0;
}
Output:
1 2 4 5
#include <iostream>
int main() {
int i = 1;
do {
if (i == 3) {
++i;
continue; // Skip the current iteration when i equals 3
}
std::cout << i << " ";
++i;
} while (i <= 5);
std::cout << std::endl;
return 0;
}
Output:
1 2 4 5
The continue
statement is useful when you want to skip certain iterations of a loop based on a specific condition, allowing you to control which parts of the loop’s code are executed during each iteration.