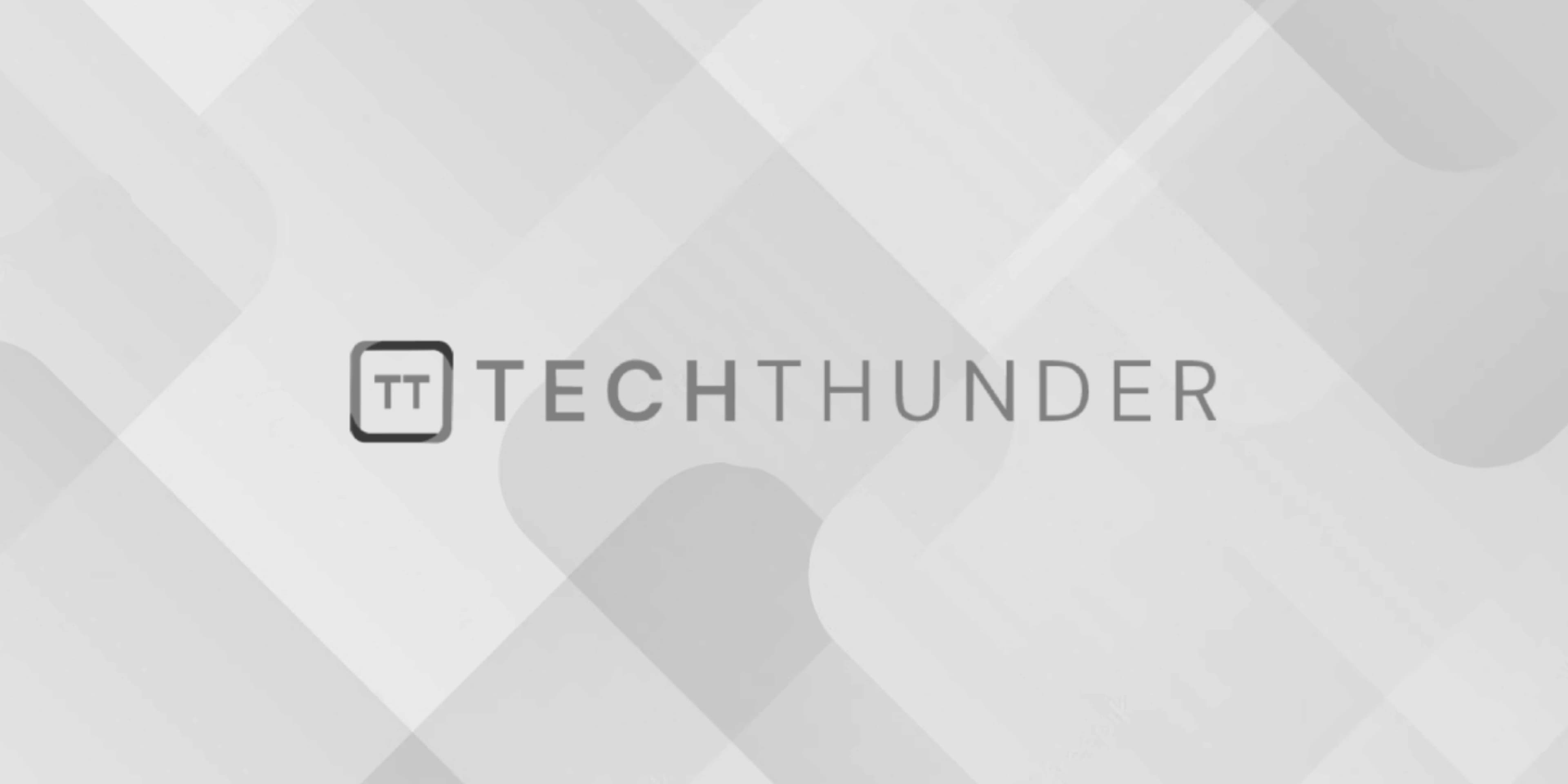
unordered_map in C++
The C++ std::unordered_map
is a container provided by the Standard Library that stores key-value pairs in an unordered manner. It is a type of associative container that provides fast access to values based on their keys. The primary characteristic of an std::unordered_map
is that it uses a hash table data structure to achieve constant-time average complexity for search, insertion, and deletion operations.
Here’s how to use std::unordered_map
:
#include <iostream>
#include <unordered_map>
#include <string>
int main() {
// Declare an unordered_map with string keys and integer values
std::unordered_map<std::string, int> myMap;
// Insert key-value pairs into the unordered_map
myMap["Alice"] = 25;
myMap["Bob"] = 30;
myMap["Charlie"] = 22;
// Access values by key
std::cout << "Bob's age is " << myMap["Bob"] << " years." << std::endl;
// Check if a key exists in the unordered_map
if (myMap.find("David") != myMap.end()) {
std::cout << "David's age is " << myMap["David"] << " years." << std::endl;
} else {
std::cout << "David is not in the map." << std::endl;
}
// Iterate through the unordered_map
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << " years" << std::endl;
}
return 0;
}
In this example:
- We include the
<unordered_map>
and<string>
headers to work withstd::unordered_map
and strings. - We declare an
std::unordered_map
calledmyMap
with string keys and integer values. - We insert key-value pairs into the unordered_map using the
[]
operator. - We access values by key using the
[]
operator. - We check if a key exists in the unordered_map using the
find
method. - We iterate through the unordered_map using a range-based for loop.
std::unordered_map
is a versatile container suitable for various use cases where you need fast lookup times based on keys. Keep in mind that the order of elements in an std::unordered_map
is not guaranteed to be in any particular order; it depends on the hash function and the underlying implementation.