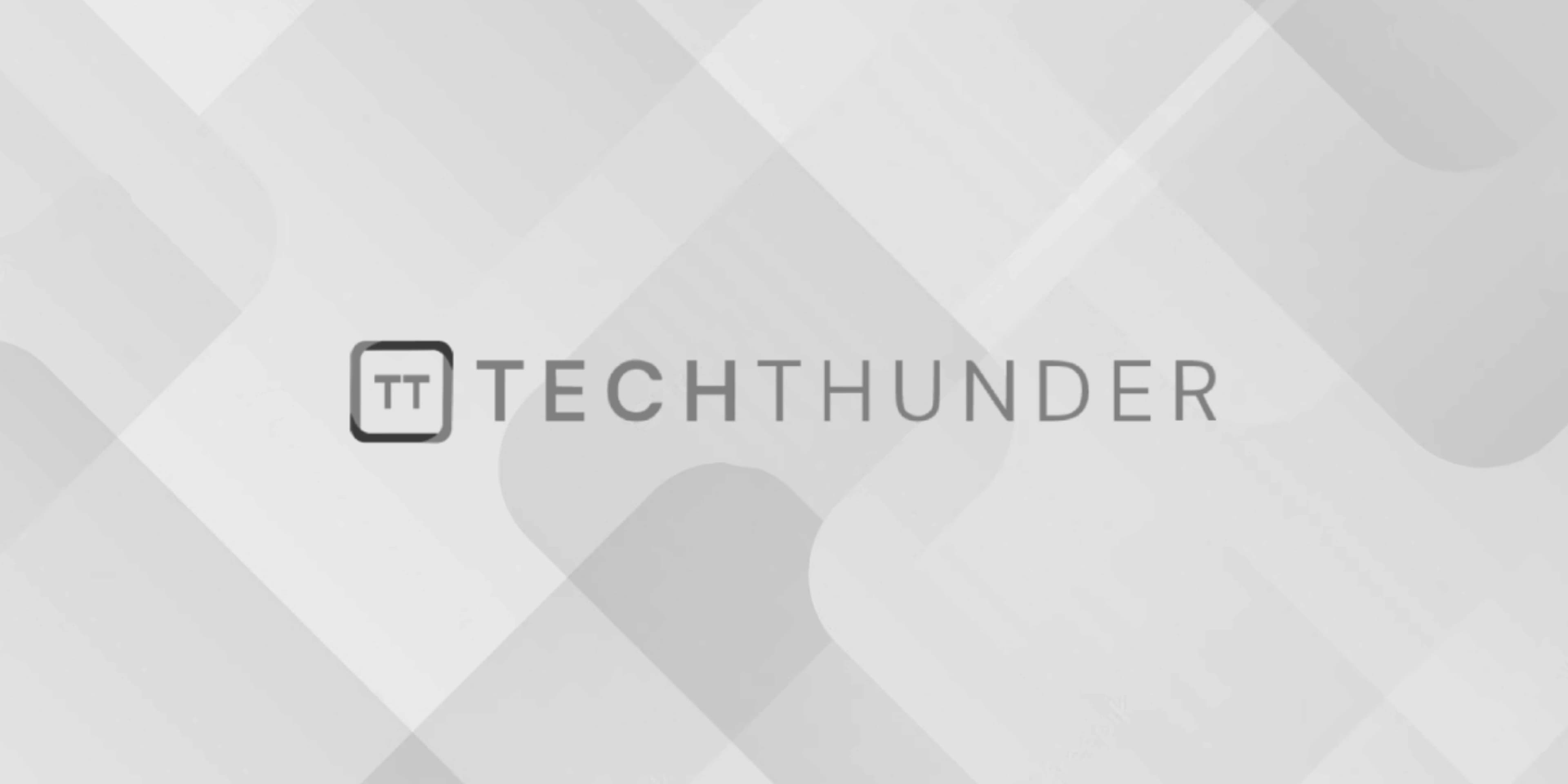
C++ Enumeration
The C++ enumeration (enum) is a user-defined data type that consists of a set of named integer constants. Enumerations provide a way to define a list of named values that are typically used to represent a set of related constant values in a more readable and maintainable way.
Here’s how you declare and use an enumeration in C++:
enum Color {
Red, // 0
Green, // 1
Blue // 2
};
In this example, we’ve defined an enumeration type called Color
with three named constants: Red
, Green
, and Blue
. By default, the first enumerator (Red
) is assigned the value 0, and subsequent enumerators are incremented by 1. So, Green
has the value 1, and Blue
has the value 2.
Here are some key points about C++ enumerations:
- Underlying Type: Enumerations have an underlying integral type (usually
int
), which determines the size and range of values that the enum can represent. You can explicitly specify the underlying type if needed:
enum Color : short {
Red, Green, Blue
};
- Enumerators as Constants: Enumerators can be used like constants in your code. For example:
Color color = Red;
if (color == Green) {
// Code for green
}
- Scoped Enumerations (C++11 and later): In C++11 and later, you can define enumerations with a specific scope to avoid polluting the global namespace:
enum class TrafficLight {
Red, Green, Yellow
};
TrafficLight light = TrafficLight::Green;
In this case, you need to use the scope operator ::
to access enum values (e.g., TrafficLight::Red
).
- Explicit Values: You can explicitly specify values for enumerators. If you do this for one or more enumerators, the following enumerators will be incremented from that point:
enum Days {
Monday = 1,
Tuesday, // 2
Wednesday // 3
};
- Forward Declarations: You can forward declare enumerations without specifying their values, and later provide the values in a separate declaration:
enum Color; // Forward declaration
enum Color {
Red, Green, Blue
};
- Enum to int Conversion: By default, you can implicitly convert enum values to integers, and vice versa:
int redValue = Red; // Implicit conversion
Color blue = static_cast<Color>(2); // Explicit conversion
- Enum Class Safety: Scoped enumerations provide better type safety compared to traditional enumerations, as their values are not implicitly convertible to integers.
C++ enumerations are commonly used to improve code readability and maintainability by giving meaningful names to sets of constant values. Scoped enumerations, introduced in C++11, provide better encapsulation and reduce the risk of naming conflicts in large codebases.