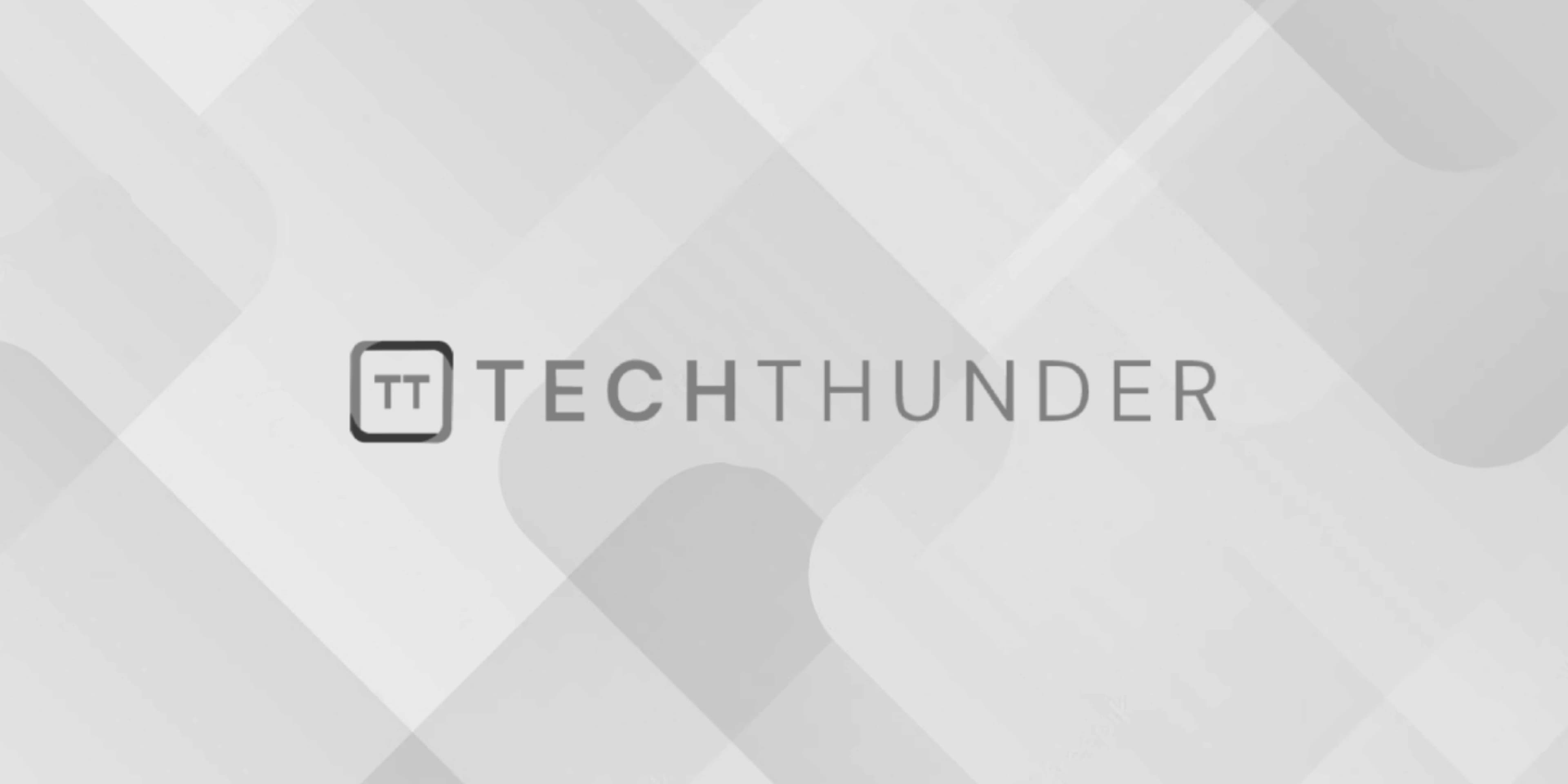
C++ Virtual Function
The C++ a virtual function is a member function of a class that is intended to be overridden by derived classes. Virtual functions enable polymorphism, which allows you to call the appropriate function implementation at runtime based on the actual object type rather than the reference or pointer type. Virtual functions are a key concept in object-oriented programming (OOP) and are often used in base classes to define a common interface that derived classes can implement as needed.
Here are the key aspects of virtual functions in C++:
- Declaring a Virtual Function: To declare a virtual function in a class, you use the
virtual
keyword before the function’s return type in the base class. This marks the function as virtual and indicates that it can be overridden in derived classes.
class Base {
public:
virtual void someFunction() {
// Base class implementation
}
};
- Overriding a Virtual Function: Derived classes that inherit from the base class can override the virtual function by providing their own implementation with the same function signature. You use the
override
keyword (C++11 and later) to indicate that you intend to override a virtual function.
class Derived : public Base {
public:
void someFunction() override {
// Derived class implementation
}
};
- Polymorphism and Dynamic Dispatch: Virtual functions enable polymorphism, which means that the appropriate function implementation is determined at runtime based on the actual object type. This allows you to use a base class pointer or reference to call a function that is overridden in a derived class.
int main() {
Base* basePtr;
Derived derivedObj;
basePtr = &derivedObj; // Pointer to a derived class object
basePtr->someFunction(); // Calls the derived class implementation
return 0;
}
In this example, even though basePtr
is of type Base*
, it calls the someFunction
implementation of the Derived
class because someFunction
is declared as virtual.
- Default Implementation: If a derived class chooses not to override a virtual function, it inherits the base class’s implementation. The base class implementation serves as a default behavior that can be extended or modified by derived classes.
class AnotherDerived : public Base {
// No explicit override for someFunction
};
- Pure Virtual Functions: A pure virtual function is a virtual function that has no implementation in the base class. It is declared with the
= 0
syntax, and any derived class must provide an implementation for it. Classes with pure virtual functions are called abstract classes, and you cannot create objects of such classes.
class AbstractBase {
public:
virtual void pureVirtualFunction() = 0; // Pure virtual function
};
Derived classes that inherit from AbstractBase
must implement pureVirtualFunction
.
Virtual functions and polymorphism are fundamental concepts in C++ and are widely used to create flexible and extensible class hierarchies. They allow you to write code that operates on base class pointers or references and can work with objects of derived classes, promoting code reusability and ease of maintenance.