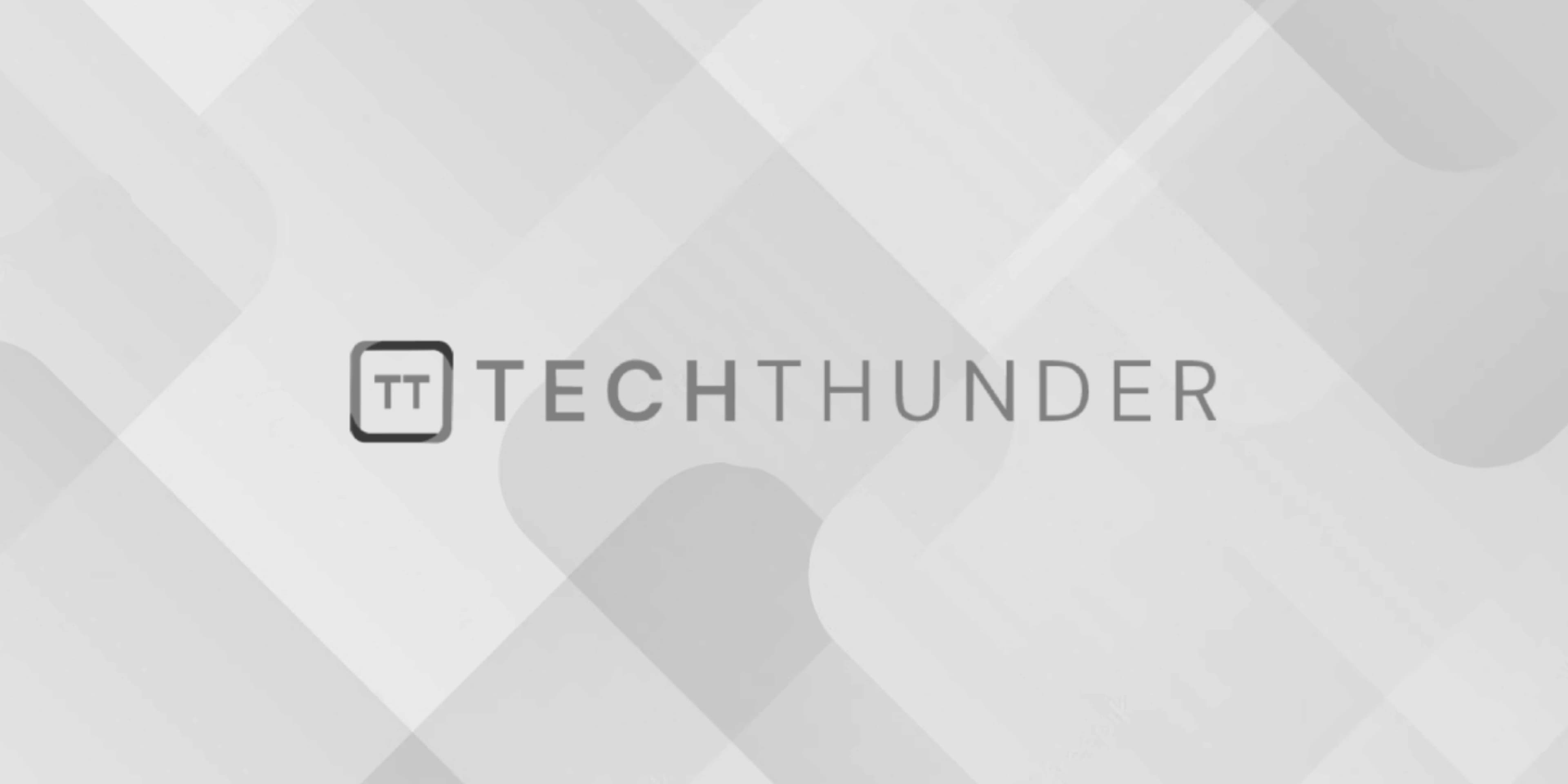
219 views
C++ cout, cin, endl
The C++ cout
, cin
, and endl
are commonly used objects and manipulators for input and output operations.
cout
:
cout
is an instance of theostream
class, which is used for sending output to the standard output stream (usually the console or terminal).- You can use
cout
to display text and data on the screen. - Example:
C++
#include <iostream>
int main() {
std::cout << "Hello, world!" << std::endl;
return 0;
}
In this example, “Hello, world!” is displayed on the console.
cin
:
cin
is an instance of theistream
class, which is used for reading input from the standard input stream (usually the keyboard).- You can use
cin
to receive input from the user. - Example:
C++
#include <iostream>
int main() {
int num;
std::cout << "Enter a number: ";
std::cin >> num;
std::cout << "You entered: " << num << std::endl;
return 0;
}
In this example, the user is prompted to enter a number, which is then stored in the variable num
.
endl
:
endl
is a manipulator that represents the end of a line or newline character ('\n'
).- When used with
cout
, it not only outputs a newline character but also flushes the output buffer, ensuring that the text is displayed immediately. - Example:
C++
#include <iostream>
int main() {
std::cout << "Line 1" << std::endl;
std::cout << "Line 2" << std::endl;
return 0;
}
In this example, “Line 1” and “Line 2” are displayed on separate lines due to the use of endl
.
While cout
and cin
are standard objects provided by the C++ Standard Library for input and output, endl
is a standard manipulator. These three components are commonly used when working with console-based input and output operations in C++.