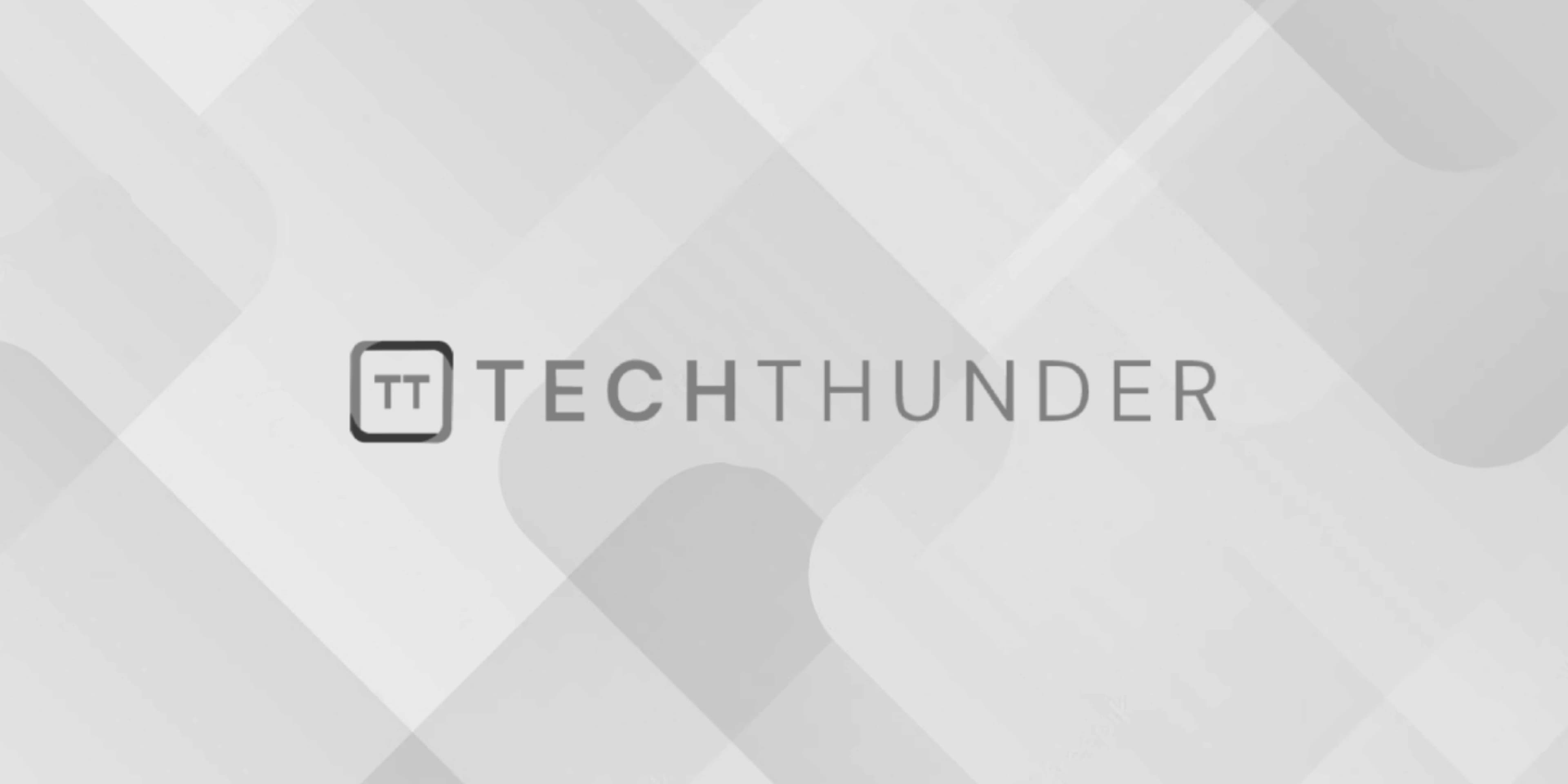
C++ Array of Pointers
The C++ array of pointers is an array in which each element is a pointer to some data type. This can be useful for various purposes, such as managing dynamic data, creating lists, or storing pointers to objects. Here’s how you can create and use an array of pointers in C++:
#include <iostream>
int main() {
// Create an array of pointers to integers
int* intPtrArray[5];
// Create some integer values
int a = 10, b = 20, c = 30, d = 40, e = 50;
// Assign pointers to elements in the array
intPtrArray[0] = &a;
intPtrArray[1] = &b;
intPtrArray[2] = &c;
intPtrArray[3] = &d;
intPtrArray[4] = &e;
// Access and print the values using the pointers
for (int i = 0; i < 5; ++i) {
std::cout << "Value at index " << i << ": " << *(intPtrArray[i]) << std::endl;
}
return 0;
}
In this example, we:
- Declare an array of pointers to integers:
int* intPtrArray[5];
- Create integer variables
a
,b
,c
,d
, ande
. - Assign the addresses of these variables to the elements of the pointer array.
- Access and print the values stored in the variables using the pointers.
Arrays of pointers are particularly useful when you need to manage dynamic data, such as arrays of varying sizes, strings, or objects. They allow you to create lists of pointers to elements that can be dynamically allocated and deallocated at runtime.
Here’s another example that demonstrates how you can use an array of pointers to create an array of strings:
#include <iostream>
int main() {
// Create an array of pointers to strings
const char* stringArray[] = {"apple", "banana", "cherry", "date", "elderberry"};
// Access and print the strings
for (int i = 0; i < 5; ++i) {
std::cout << "String at index " << i << ": " << stringArray[i] << std::endl;
}
return 0;
}
In this example, each element of the array stringArray
is a pointer to a C-style string (a sequence of characters). This allows you to create an array of strings without specifying the size of each string in advance.