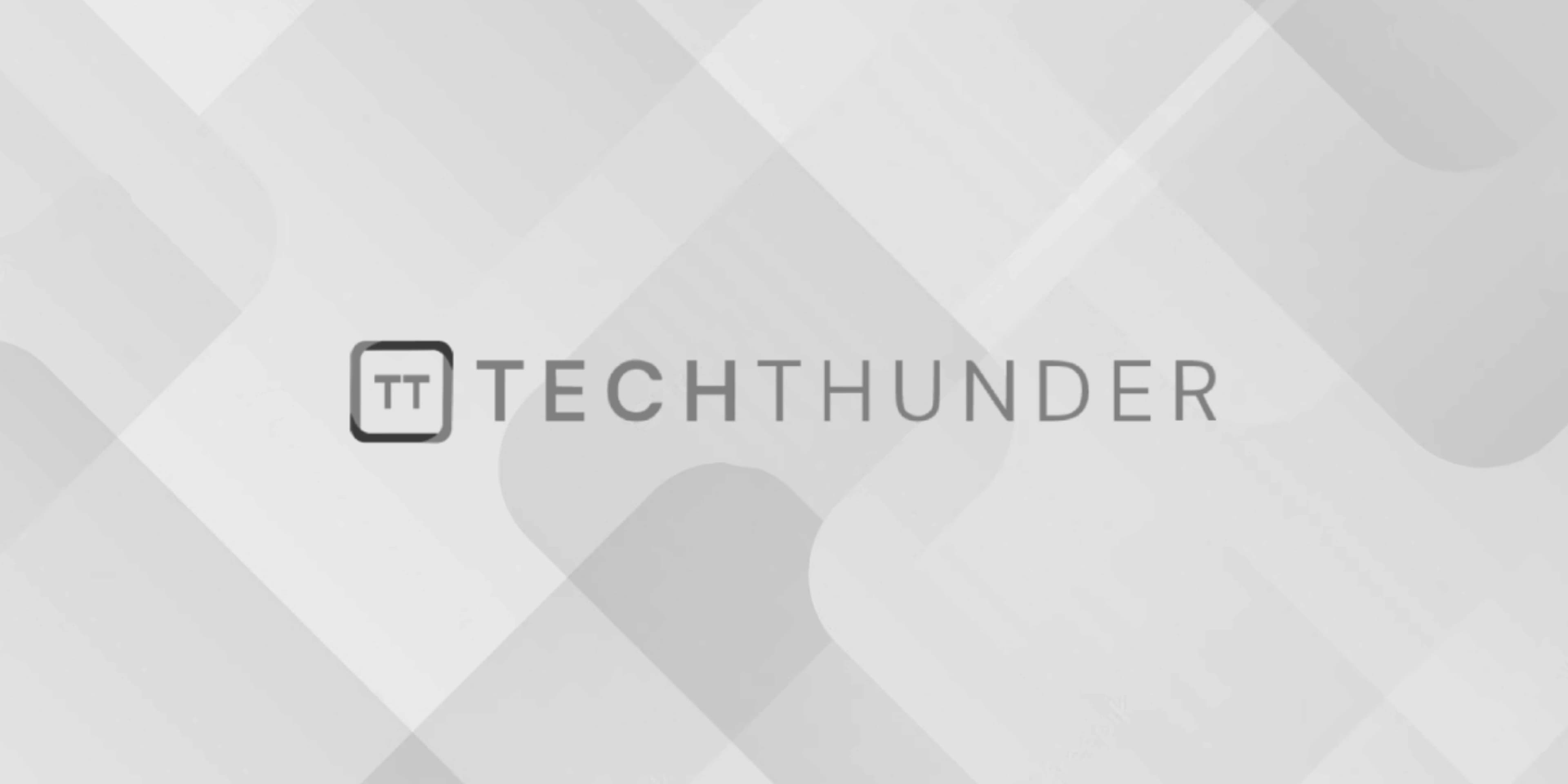
183 views
C++ Program To Sort An Array In Descending Order
You can sort an array in descending order in C++ using the standard library function std::sort
. However, since std::sort
sorts in ascending order by default, you need to provide a custom comparison function to sort in descending order. Here’s an example of how to do this:
#include <iostream>
#include <algorithm>
bool compareDescending(int a, int b) {
return a > b; // Compare in descending order
}
int main() {
int arr[] = {5, 2, 9, 1, 5, 6};
int n = sizeof(arr) / sizeof(arr[0]);
std::sort(arr, arr + n, compareDescending);
std::cout << "Array sorted in descending order: ";
for (int i = 0; i < n; ++i) {
std::cout << arr[i] << " ";
}
std::cout << std::endl;
return 0;
}
In this code:
- We include the necessary headers
<iostream>
and<algorithm>
. - We define a custom comparison function
compareDescending
, which returnstrue
ifa
should come beforeb
in the sorted order (i.e., ifa
is greater thanb
for descending order). - In the
main
function, we define an integer arrayarr
and determine its sizen
. - We use
std::sort
to sort the arrayarr
in descending order by passing thecompareDescending
function as the third argument tostd::sort
. - Finally, we print the sorted array in descending order.
After running this program, the arr
array will be sorted in descending order, as specified by the custom comparison function.