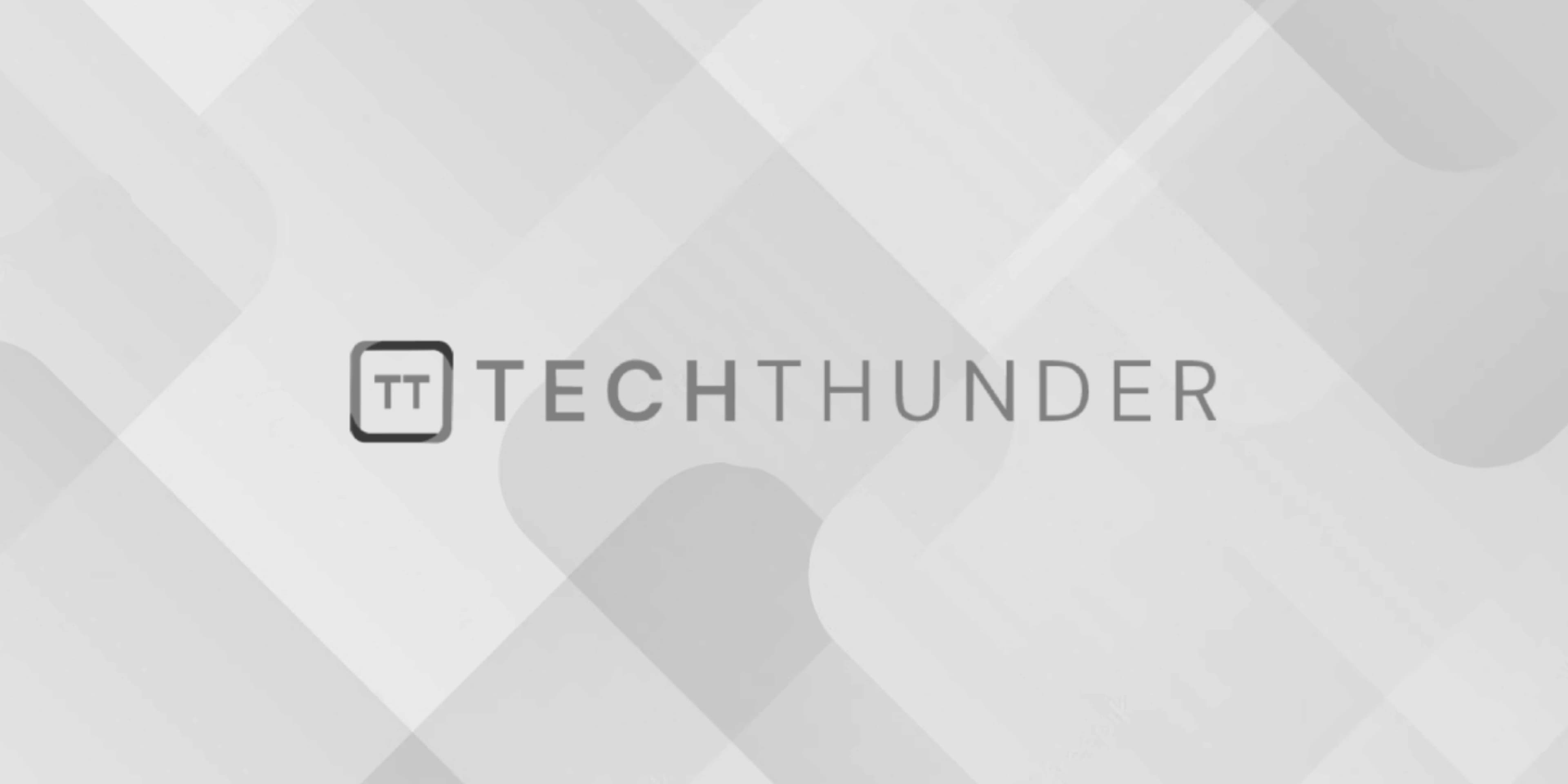
Type Inference in C++ (auto and decltype)
The C++ type inference refers to the ability of the compiler to deduce the data type of a variable or expression at compile time without the need for explicit type declarations. Two primary mechanisms for type inference in C++ are the auto
keyword and the decltype
keyword.
1. auto
Keyword:
The auto
keyword is used for type inference and was introduced in C++11. It allows you to declare variables without explicitly specifying their data types, and the compiler will determine the appropriate type based on the initializer.
Here are some examples of using auto
:
auto x = 42; // x is deduced as int
auto pi = 3.14159; // pi is deduced as double
auto name = "Alice"; // name is deduced as const char* (C-style string)
auto
is particularly useful when working with iterators, function templates, and complex data structures like iterators in a range-based for loop:
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (auto it = numbers.begin(); it != numbers.end(); ++it) {
// 'it' is deduced as 'std::vector<int>::iterator'
std::cout << *it << " ";
}
2. decltype
Keyword:
The decltype
keyword is used to deduce the type of an expression at compile time. It returns the declared type of an expression without actually evaluating it. This can be handy when you want to extract the type of an expression, especially in template metaprogramming scenarios.
Here are some examples of using decltype
:
int x = 42;
decltype(x) y = x; // 'y' has the same type as 'x', which is int
double calculateValue();
decltype(calculateValue()) result; // 'result' has the same type as the return value of calculateValue()
std::vector<int> numbers = {1, 2, 3, 4, 5};
decltype(numbers.begin()) it = numbers.begin(); // 'it' has the same type as 'numbers.begin()'
decltype
is particularly useful when you need to preserve the exact type of an expression or when you want to create aliases for complex types or return types of functions.
Both auto
and decltype
enhance code readability and flexibility by allowing the compiler to deduce types, reducing the need for explicit type declarations, especially when working with complex types or expressions. However, it’s important to use them judiciously and consider readability and maintainability when deciding whether to use type inference.