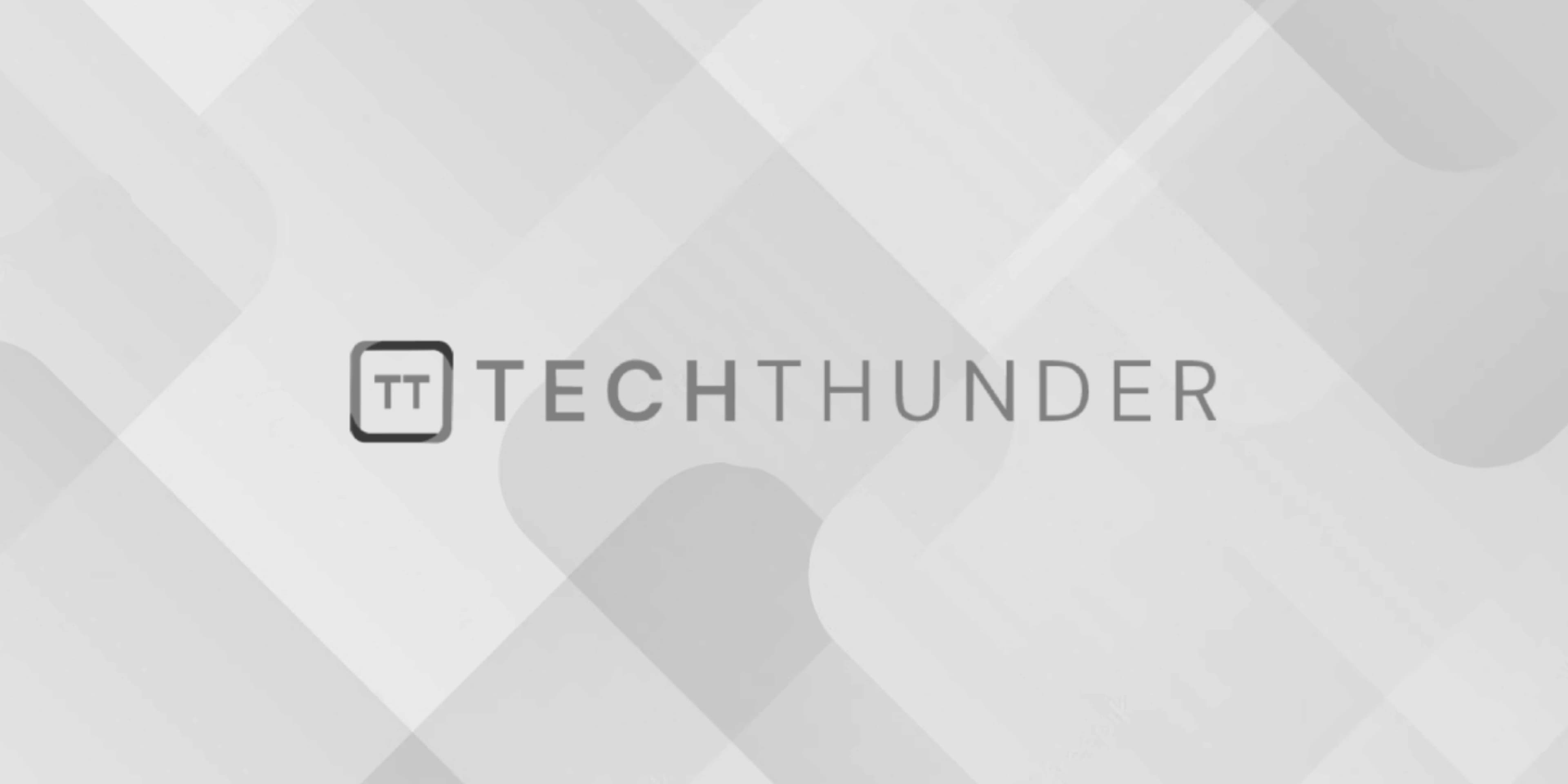
141 views
C++ Programs
The C++ programs that demonstrate various concepts and tasks. These programs cover a range of topics from basic input/output to more advanced topics like working with arrays and classes.
- Hello World:
C++
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
- User Input:
C++
#include <iostream>
int main() {
std::cout << "Enter your name: ";
std::string name;
std::cin >> name;
std::cout << "Hello, " << name << "!" << std::endl;
return 0;
}
- Basic Arithmetic:
C++
#include <iostream>
int main() {
int num1, num2;
std::cout << "Enter two numbers: ";
std::cin >> num1 >> num2;
int sum = num1 + num2;
std::cout << "Sum: " << sum << std::endl;
return 0;
}
- Factorial Calculation (using a function):
C++
#include <iostream>
int factorial(int n) {
if (n == 0 || n == 1) {
return 1;
} else {
return n * factorial(n - 1);
}
}
int main() {
int num;
std::cout << "Enter a number: ";
std::cin >> num;
int result = factorial(num);
std::cout << "Factorial of " << num << " is " << result << std::endl;
return 0;
}
- Array Operations:
C++
#include <iostream>
int main() {
int arr[] = {1, 2, 3, 4, 5};
int sum = 0;
for (int i = 0; i < 5; ++i) {
sum += arr[i];
}
std::cout << "Sum of array elements: " << sum << std::endl;
return 0;
}
- Class and Object:
C++
#include <iostream>
class Person {
public:
std::string name;
int age;
void introduce() {
std::cout << "Name: " << name << ", Age: " << age << std::endl;
}
};
int main() {
Person person;
person.name = "Alice";
person.age = 30;
person.introduce();
return 0;
}
These are just a few examples of C++ programs. Depending on your specific needs and interests, you can explore various C++ features and libraries to create more complex and specialized programs.