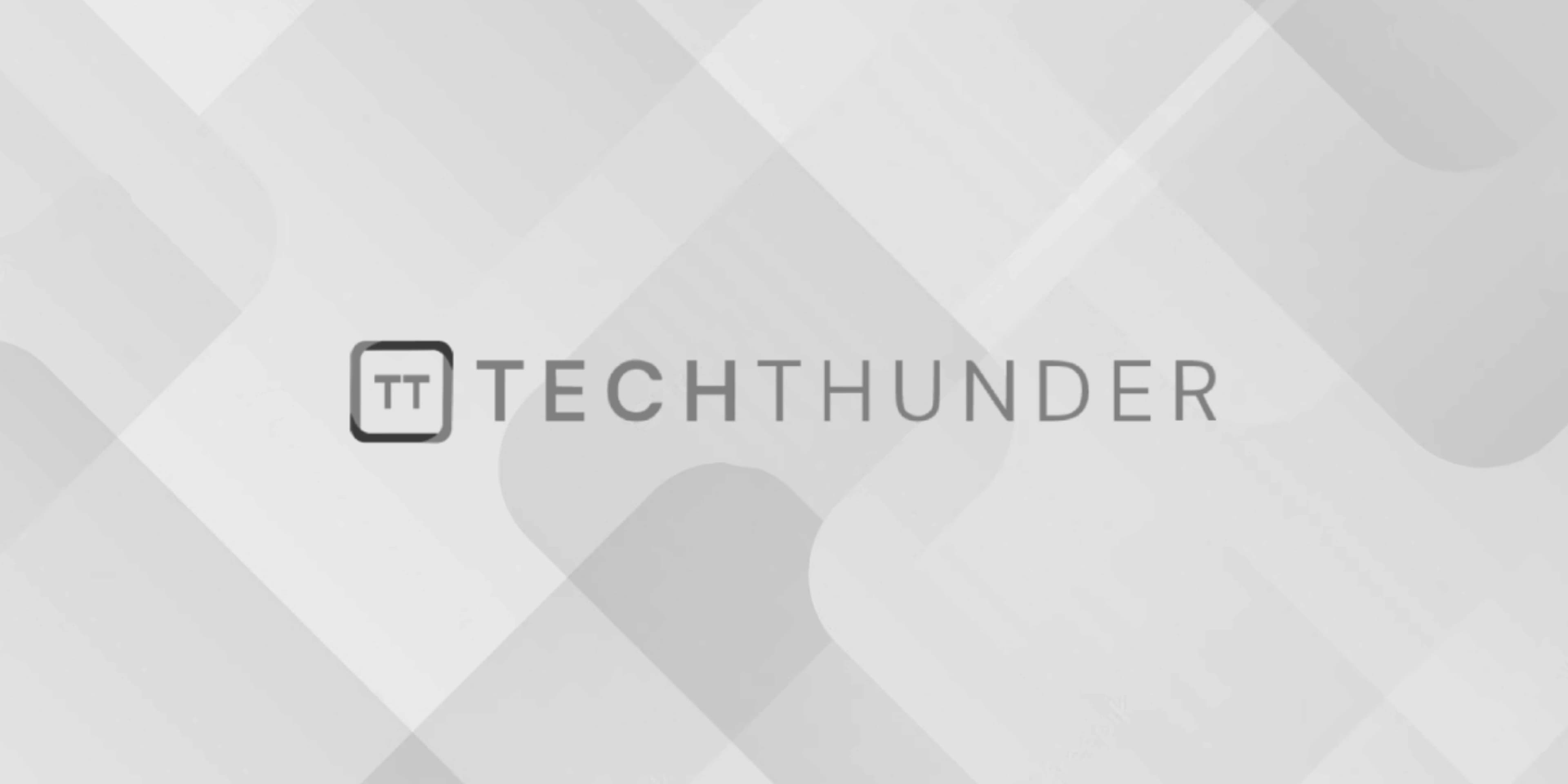
254 views
Initialize Vector in C++
The C++ can initialize a vector in several ways. Here are some common methods for initializing a vector:
- Empty Initialization:
- You can create an empty vector without any elements using the default constructor:
C++
std::vector<int> myVector;
- Initialization with Size:
- You can initialize a vector with a specified size, and all elements will be default-initialized. For example, if you specify a size of 5, you’ll get a vector with 5 default-initialized elements (e.g., 0 for
int
):
C++
std::vector<int> myVector(5); // Initializes with 5 elements, all set to 0
- Initialization with Size and Value:
- You can initialize a vector with a specified size and a default value for its elements:
C++
std::vector<int> myVector(5, 42); // Initializes with 5 elements, all set to 42
- List Initialization (C++11 and later):
- You can use list initialization to initialize a vector with a set of values enclosed in braces:
C++
std::vector<int> myVector = {1, 2, 3, 4, 5};
This syntax is available in C++11 and later versions.
- Using the Range Constructor (C++11 and later):
- You can initialize a vector with values from another iterable container, such as an array or another vector:
C++
int arr[] = {1, 2, 3, 4, 5};
std::vector<int> myVector(arr, arr + sizeof(arr) / sizeof(arr[0])); // Copy values from the array
This method is available in C++11 and later versions.
- Using Initializer Lists (C++11 and later):
- If you have a function that takes an
std::initializer_list
, you can use this to initialize a vector:
C++
std::vector<int> myVector = {1, 2, 3, 4, 5};
This syntax is available in C++11 and later versions.
- Using Other Containers (C++11 and later):
- You can initialize a vector with elements from another container using the range-based constructor. This is particularly useful when you want to copy elements from another container like a list or deque:
C++
std::list<int> myList = {1, 2, 3, 4, 5};
std::vector<int> myVector(myList.begin(), myList.end());
This method is available in C++11 and later versions.
Choose the initialization method that best fits your needs and the version of C++ you are using. C++11 and later versions offer more concise and convenient initialization options like list initialization and initializer lists.